What is VBScript?
What is VBScript?
VBScript is a powerful tool for managing data and storing multiple values. VBScript can be used to automate testing tasks in tools like QTP (UFT) and Test Complete.
As an interpreted language, VBScript doesn’t need compilation like other programming languages like C, C++ or Java do.
By employing its Option Explicit statement and adhering to proper syntax requirements, VBScript can manage complex data structures efficiently as well as handle errors more reliably than its peers.
Automation with VBScript can automate administrative tasks on computers, such as mapping drives or managing software inventories for multiple computers.
Built into Windows OS and designed as an easy learning experience.
Visual Basic was once considered to be difficult and fun, yet now VBScript makes script writing much simpler and enjoyable.
In its full name VBScript stands for Microsoft Visual Basic Scripting edition.
Storage Efficiency with Arrays in VBScript
In VBScript, arrays offer an efficient means of storing multiple values within one variable without declaring separate variables for them separately.
Fixed arrays have an established size; dynamic arrays offer greater flexibility by using ReDim statements to adapt their number of elements as necessary.
Furthermore, Redim Preserve statements ensure existing data remains undamaged when resizing an array.
VBScript’s UBound function makes data management simpler by retrieving an array’s upper limit, making data management much simpler.
When choosing between fixed or dynamic arrays for data management purposes, each application’s requirements need to be taken into consideration, with fixed arrays being suitable for fixed sizes of information while dynamic ones allow adaptability for changing requirements.
Working With Dynamic and Fixed Arrays in VBScript
The UBound function determines the upper bound for arrays that is set at 5, using an iterative loop from zero to five.
Echo statement prints each element in the loop. When resizing an array with existing data, use ReDim statement carefully as data loss could occur without Preserve keyword applied.
Fixed arrays contain fixed elements while dynamic ones expand and contract as required.
An array named Numbers stores six values with index numbers from 0-5 for storage purposes.
FillNumbers assigns numbers from 10-12 while AverageNumbers uses an infinite loop to compute and average all numbers within its array.
Finally, its output displays this calculated average.
Implementing and Controlling Loops in VBScript
VBScript uses the For Next loop to execute code repeatedly based on a condition. A variable is initialized as zero and incremented each iteration by one, until its value reaches 5 or less; thereafter the loop terminates by incrementing by 2.
Utilising Step parameter allows custom increments such as increasing by 2 rather than 1.
Exit For statements provide flexibility when controlling loop execution and improving script performance by terminating loops when certain conditions have been fulfilled – providing early loop exits when desired.
They’re great tools for controlling loop execution and optimizing script performance!
Implementing For Next and For Each Loops in VBScript
A For Next loop enables the counter variable to rise or fall depending on its Step value; negative steps may be possible if provided, otherwise defaulting to 1. If no Step value is specified then defaults to 1.
For Each loops are used to execute code for every element in a collection such as an array or folder contents, for instance when listing files using On Error Resume Next (OERN) the script continues execution regardless of any errors that arise during its run.
Three variables-objFSO, folder and file-are declared and set using Set statement; folder is declared using an object of file system objects created and assigned through Set statement; script then iterates through all folder files by iteration using Name property to display complete pathname of files iterated through.
One method involves retrieving hard drive information from a computer using script. In order to do this, three object variables objWMIService, objHardDisks and objDrive were declared.
Utilizing GetObject, setting objWMIService = GetObject(“winmgmts:com-PC”) retrieves system information where “com-PC” represents computer name.
Additionally, setting the HardDisks variable executes a query which selects all physical disks providing information regarding total and free disk space available for storage.
The For Each Next Statement effectively processes elements within a collection. This technique can be useful in tasks like file manipulation and data retrieval from system servers.
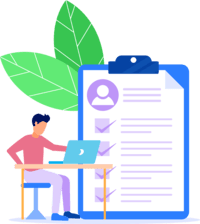
VBScript Training
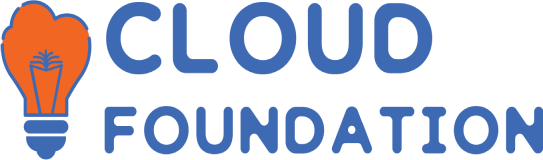
Conditional Logic and String Functions in VBScript
VBScript contains various functions and conditional statements to manipulate strings and manage program flow.
For instance, its UCASe function converts words from lowercase to uppercase while Trim removes extra spaces from strings.
Conditional execution can be accomplished using If…Then…ElseIF…End If, which allows various actions depending on a variety of conditions to take place; additionally the InStr function helps identify substrings within strings for use as logic checks.
VBScript supports numerous built-in constants to increase readability.
Utilising these constants makes VBScript effective at handling decision making processes and string operations efficiently.
String Comparison and Conditional Execution in VBScript
StrComp in VBScript compares two strings based on three arguments, such as their first string, second string and comparison mode.
When both strings match exactly, StrComp will return zero and display an information box stating: Both strings match exactly.
If one string is greater than another, StringCompare.vbs returns 1 and displays an appropriate message.
Instances in which an error arise are handled using Case Else statements which display error messages when necessary.
To use it yourself, save and run StringCompare.vbs with various string inputs as soon as you want.
By default, this function compares text in case-insensitive fashion; adding the vbTextCompare constant allows case-sensitive comparisons.
Furthermore, using Select Case…End Select statement helps evaluate expressions and take actions according to values evaluated during this search process.
Procedures in VBScript enhance code reuse by streamlining string comparison and conditional execution processes, making string manipulation and conditional execution faster and more cost effective.
Implementing Sub and Function Procedures in VBScript
Within the language itself, procedures enhance modularity and efficiency by breaking tasks down into modularized pieces and increasing task efficiency through task isolation.
There are two primary categories of procedures; Sub procedures and Function procedures.
KilosPoundsSub.vbs shows us an example of using Sub procedures without returning an output value; such as performing actions but without returning one.
Specifically, this script takes user input at command prompt window level before calling ConvertWeight Sub procedure in order to convert weight from pounds (1 kg = 2.205 pounds).
After clicking “OK”, a message box displaying the results will be opened and an EndScript procedure called to thank them for participating.
A Sub procedure may then be executed using either Call statement or by providing parameters within parentheses.
Call statements allow repeated execution and modifications (e.g. changing conversion factors) without impacting other parts of a script.
On the other hand, Function procedures in VBScript start off with Function statement and end with End Function statements respectively.
Function procedures differ from Sub procedures by returning values; for instance, KilosPoundsFunction.vbs uses user input converted into double and passed onto a function which multiplies it by 2.205.
Function procedures are useful for calculations that return dynamic values in message boxes, making function procedures an efficient means of dynamic calculations and returns.
Loops also play a vital role; For instance, While…Wend loops run code repeatedly until certain conditions become true again.
Loop1 procedure uses While…Wend’s automatic increment statements to initialize i = 1, then runs while it drops below five iterations threshold, with message box output for every iteration containing information about current iteration of Loop.
A custom increment statement must also be implemented manually so as to prevent an infinite loop scenario from developing.
This type of loop can be particularly effective when the number of iterations is unknown; execution continues so long as a condition holds true.
VBScript procedures play an integral part in automating tasks such as creating text files, HTML pages and Excel sheets.
By employing Sub and Function procedures, scripts become more organized, readable and efficient while at the same time being easier to reuse code for efficient execution.
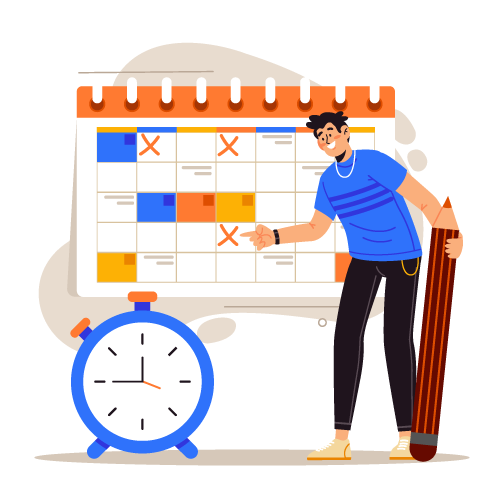
VBScript Online Training
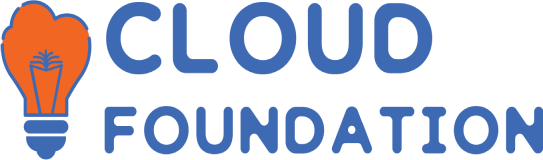
Utilising Loops in VBScript for Automating Report Generation
A while loop is used to increase variables until they meet a given condition, stopping execution when that condition no longer applies and thus terminating execution when its unmet.
To generate HTML reports using file system objects in VBScript. Write Head and Body are separate subprocedures used to generate HTML markup; respectively.
Write Head defines an HTML head section while Body provides body structure using headings, paragraphs and tables; this script creates an HTML report showing order details dynamically.
Do Loop statements in VBScript offer two versions.
Do While Condition executes only when its condition initially meets.
Do -Loop While Condition executes at least once prior to checking its condition.
Python scripting demonstrates this looping concept by controlling execution according to conditions, while for Excel report automation purposes VBScript scripts create and modify Excel files automatically.
FileDelete and WriteExcelReport provide automation that enhances efficiency when it comes to dynamic reports generation using VBScript, by clearing away existing files while offering structured data entry via Excel.
Creating HTML and Excel Reports With VBScript
VBScript allows for the automation and creation of Excel files by adding workbooks, assigning values to cells and applying formatting.
Furthermore, it enables alteration to cell colors, font sizes and structured data such as “Orders Placed” and “Number of Customers.”
This script makes use of Active Cell and Entire Column formatting techniques, applies AutoFit method for text alignment purposes, and employs WriteExcelReport script for writing formatted report.
A FileDelete subprocedure deletes old files prior to creating updated version of them.
The While Wend statement provides an efficient looping mechanism by running code when an specified condition remains True, while Do Loop allows more flexible control by checking conditions at either end of a loop cycle.
The Do While Condition variation only executes if its condition initially evaluates as True; while with Do-Loop While Condition execution takes place at least once prior to evaluating this condition.
These techniques enable more efficient automation of repetitive tasks using VBScript, improving report generation and data formatting capabilities.
Conclusion
VBScript has long been one of the more widely-used scripting languages, used for numerous applications like automating tasks and designing dynamic web pages to constructing standalone applications.
While other scripting languages have emerged and seen greater adoption over time, VBScript continues to be used by developers who work within Windows-based environments.
Because of its simplicity, compatibility with Microsoft products, and adaptability, Powershell can serve as an indispensable resource for developers looking to automate workflows and develop dynamic web experiences.
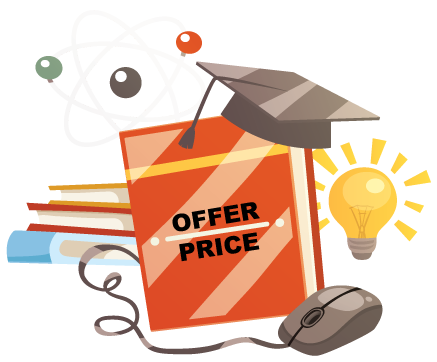
VBScript Course Price
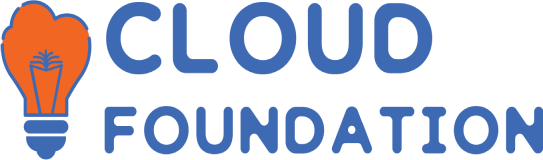
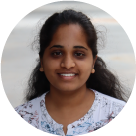
Vanitha
Author