Spring Boot Interview Questions and Answers
Spring Boot is an open-source framework used for developing web and cloud-based apps, built upon the Spring Framework. Providing an efficient means to produce production-grade apps quickly, Spring Boot provides features like its built-in server, automated configuration management tools and support for popular build tools and libraries; making it a versatile tool ideal for both small services as well as complex distributed systems.
1. What is Spring Boot?
Spring Boot is a Java-based solution for developing web applications that makes it easier to use the Spring framework.
2. What are some of the features of Spring Boot?
Some of the features of Springboard include Auto Dependency Resolution, Embedded HTTP servers, Autoconfiguration Management, Endpoints, and Springboard CLI.
3. What is Spring Boot CLI?
Spring Boot CLI is a module of Spring that creates a Spring application project that can be run or executed.
4. How do I install and set up Spring Boot CLI?
To install and set up Spring Boot CLI, you can download Apache Maven and Spring Boot CLI from their respective official websites and follow the instructions provided.
5. What is the Spring Tool Suit?
The Spring Tool Suit is a plugin for Eclipse ID or IntelliJ that helps with the development of Spring-based applications.
6. How can I build a Hello World project with the Spring Tool Suit?
To build a Hello World project using the Spring Tool Suit, go to File, then New, then Spring Startup Project, or search for Springboard and select Spring Startup Project. Then you can set up the project, add dependencies, and start writing code for it.
7. What is Spring Framework’s Model View Controller (MVC) and why is it important?
The Model View Controller (MVC) design pattern allows inversion of control and dependency injection. Spring Framework uses it to arrange application development and class dependencies.
8. What are the four main components of the MVC pattern?
The four main components of the MVC pattern are the model, controller, view, and front controller.
9. What is dependency injection and how does it work in Spring Framework?
Objects depend on other objects for functionality via dependency injection. Spring Framework uses the dispatcher servlet to block requests and call the view component for dependency injection.
10. What are the three types of dependency injection in Spring Framework?
The three types of dependency injection in Spring Framework are constructor injection, setter injection, and interface injection.
11. What are the advantages of utilizing dependency injection in Spring Framework?
The advantages of employing dependency injection in Spring Framework include improved component interaction, simpler application expansion, better unit testing, and less boilerplate code.
12. What is the role of the dispatcher servlet in the Model View Controller (MVC) pattern?
A dispatcher servlet is a front-end controller that acts as a bridge between incoming requests and the view resolver, it examines the view resolver’s entry in the XML file and then launches the given view components.
13. What are the functions of the model and view objects in the Model View Controller (MVC) pattern?
The model object includes the application’s essential data, which might be a single object or a collection of objects sent to the controller. The view shows the information in a certain format.
14. What is the MVC pattern workflow?
The Model View Controller (MVC) pattern blocks incoming requests by the dispatcher servlet, which validates the view resolver entry in the XML file and launches the view component.
To determine application resources and appearance, the model and view objects are examined.
15. What is the role of the front controller in the Model View Controller (MVC) pattern?
The front controller is a mediator between incoming requests and the view resolver. It obstructs incoming requests and invokes the specified view component.
16. How does the Spring Framework provide a dignified solution to use the MVC pattern?
The Spring Framework provides a dignified solution to use the MVC pattern by using the dispatcher servlet.
17. What is the role of the view resolver in the Model View Controller (MVC) pattern?
The view resolver is responsible for resolving the view component from the XML file. It is called by the dispatcher servlet to determine the appropriate view component to render.
18. What is JSP?
JSP stands for Java Server Pages, which is a technology used to create dynamic web pages.
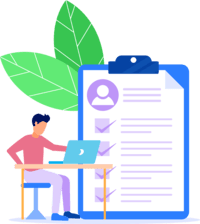
Spring Boot Training
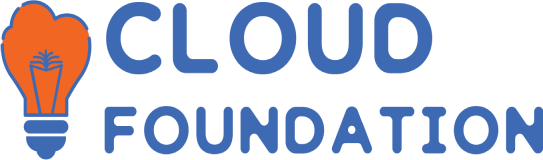
The Ad Rotator control annotation is used to configure the request in a Springboard application, it is used to specify the slash courses in the request mapping.
20. What is the use of the request mapping annotation?
The request mapping annotation is used to map URLs to webpages, it is used to specify the URLs that the application should handle.
21. What is the significance of the response body annotation?
The response body annotation is used to return specific data from the server, it is used to specify the data that the application should return.
22. What exactly is the objective of the Jasper dependency?
The Jasper dependency is used to add Jasper to the application, it is used to enable the JSP files to be used in the application.
23. What does the Palm dot XML file serve as?
The Palm dot XML file is used to add Jasper to the application, it is used to enable the JSP files to be used in the application.
24. What is the intent of the adorate response body annotation?
The adorate response body annotation is used to return specific data from the server, it is used to specify the data that the application should return.
25. What is the importance of the Adriatic Auto-Wired annotation?
The Adriatic Auto-Wired annotation is important because it automatically gets all auto configurations for the repository and ensures that everything is configured automatically in Spring Boot.
26. What is the goal of the REST API framework?
The REST API framework is a communication architecture that uses less bandwidth to make applications more internet-friendly.
This resource-based language is commonly called the internet language. REST API helps developers manage application resources and increase performance.
27. What is REST services?
HTTP methods (GET, POST, PUT, DELETE) are used in REST (Representational State Transfer) web service architecture to interact with resources.
These stateless REST services require the client to send the server all the information needed to interpret and perform the request. Web apps employ REST APIs to modify and transfer data.
28. What is JPA?
JPA stands for Java Persistence API. This Java-based persistence framework standardizes relational database interaction in Java applications.
JPA classes and annotations bind Java objects to database tables and conduct database actions including adding, updating, and removing data. Web apps often utilize JPA to interface with databases.
29. What is the SQL file used for?
The SQL file is used to ensure data is automatically entered into the database.
30. What is the security model used in Spring Boot?
Spring Boot offers three security models: spring security curve, brass spring security, auth, and spring security sample.
31. How can the web application be secured using Spring Boot?
To protect the web application using Spring Boot, start a new project and load the main class file.
Then, in the sources and templates folder, create an HTML page and include Spring Security in the dependency file to setup the application.
32. What is the importance of Spring Security in web applications?
Spring Security is a crucial module for web applications, as it ensures their security, it offers authentication and authorization for Java applications.
33. What is the distinction between Spring Boot and Spring?
Spring Boot is a module of Spring, which simplifies the use of the Spring framework for Java development, while Spring is more complex.
34. What are some advantages of using Spring Boot?
Some advantages of using Spring Boot include auto configuration, standalone applications with non-functional features, embedded Tomcat servers, server containers, and Jetty, an opinionated view, CLI tool for development testing, spring boot starters for dependency management, and various security metrics.
35. How can a Spring Boot application be created using Maven?
There are various approaches to creating a Spring Boot application using Maven, such as the Spring Startup Project Wizard, Spring Initializer, and Spring Maven project.
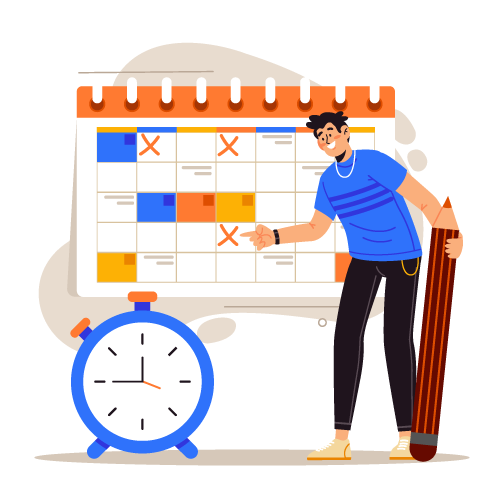
Spring Boot Online Training
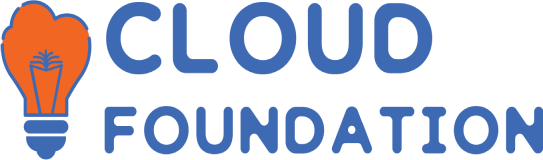
Spring Boot dependency management is a feature that allows users to automatically manage dependencies and configuration without specifying the version.
37. What are Spring Boot Dev Tools?
Spring Boot Dev Tools is a set of tools that make the development process easier.
38. What is Spring Data JPA?
Spring Data JPA is a module that provides support for JPA (Java Persistence API) and simplifies the configuration of JPA repositories.
39. What is Hibernate?
Hibernate is a Java Persistence API implementation that provides a way to map Java objects to database tables.
40. How can you connect an external database like MySQL or Oracle to a Spring Boot application?
To connect a Spring Boot application to an external database like MySQL or Oracle, add the database driver dependency to your project’s dependencies, configure the connection properties, and create a JPA repository that extends the Spring Data JPA repository for the database.
41. What is YAML?
YAML (Yet Another Markup Language) is a human-readable data serialization format.
42. What’s the variation between YAML and property files?
The main variation between YAML and properties files is that YAML uses a hierarchical syntax and allows for more complex data structures, while properties files use a simple key-value syntax.
43. What is Spring Boot Actuator?
Spring Boot Actuator is a module that provides a set of endpoints for monitoring and controlling running Spring Boot applications.
“Test your knowledge with MCQs on Spring Boot – the popular Java framework!”
1. What is Spring Boot?
a. Lightweight web framework for building RESTful APIs
b. Full-featured Java framework for building web applications
c. Database management system for storing and retrieving data
d. Messaging system for sending and receiving messages
2. Which of the following is NOT a feature of Spring Boot?
a. Embedded web server
b. Support for multiple backends (e.g. MySQL, PostgreSQL, MongoDB)
c. JPA integration
d. Support for RESTful web services
3. What is the objective of Spring Boot’s integrated web serve?
a. Enable HTTPS connections
b. Provide a secure way to run Spring Boot applications
c. Simplify deployment and setup of Spring Boot applications
d. Support a wide range of web technologies
4. Which of the following is an example of a dependency management tool used with Spring Boot?
a. Maven
b. Gradle
c. NPM
d. Yarn
5. What is the point of using Spring Boot’s auto-configuration feature?
a. Simplify the configuration of Spring Boot applications
b. Provide a default configuration that can be customized if needed
c. Force developers to manually configure their applications
d. Disable the use of external configuration files
6. What are the key characteristics of Spring Boot?
a. Supports numerous databases
b. Can create production-grade applications
c. Requires substantial configuration
d. It has a steep learning curve
7. Does Spring Boot require a separate application server?
a. Yes, it requires the Apache Tomcat application server
b. No, it can be run as a standalone application
c. Yes, it requires the Jetty application server
d. Yes, it requires the WebLogic application server
8. What is the Spring Initializer used for?
a. The program generates sample projects depending on user selections
b. Manage project dependencies
c. Configure application servers
d. It’s a tool for creating web APIs
9. Why is the Spring Boot Starter template used?
a. Pre-configured web application template
b. Pre-configured command line application template
c. Pre-configured batch task template
d. Pre-configured RESTful web service template
10. What distinguishes Spring Boot from Spring Framework?
a. Spring Boot is a separate framework with its own architecture and features
b. Spring Boot is built on top of Spring Framework and provides additional functionality
c. Spring Boot is a lean version of Spring Framework with limited features
d. There is no difference between Spring Boot and Spring Framework
11. What is the target audience for Spring Boot?
a. Small start-ups and independent developers
b. Large enterprises with complex application requirements
c. All of the above
d. None of the above
12. Which framework was created by Pivotal Software and released under the Apache Software License, version 2.0?
a. Java EE
b. Hibernate
c. Spring Boot
d. Struts
13. What is Spring Boot’s default dependency management tool?
a. Maven
b. Gradle
c. Ant
d. NPM
conclusion
Java Spring Boot Interview Questions focus around understanding its fundamentals such as dependency injection or RESTful services implementation or interoperability with external dependencies integration.
Java Spring Boot is an established framework for rapidly and efficiently developing web apps using Java programming language. Java Spring Boot makes for a versatile yet powerful development environment.
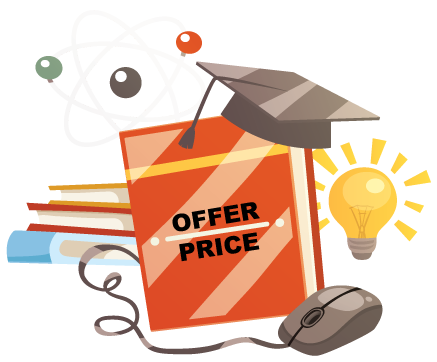
Spring Boot Course Price
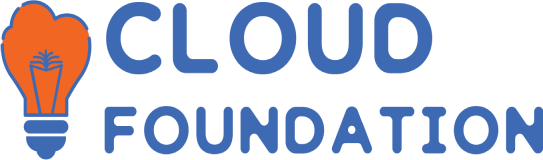
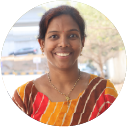
Deepthi
Author