React Hooks Tutorial
What Are React Hooks?
React hooks are functions that enable users to add State and other React features into functional components, for instance by utilising set, state, and method hooks within class components as well as any of React’s other features.
Before React, developers used React Class Components which were utilized in the Cycle React Cycle and often confused developers further.
Such methods proved complex and led to discord among members of development teams.
Hooks can be used to quickly create and manipulate elements within a system for more efficient development.
How to set up hooks in a functional component?
A functional component, also referred to as an element that adds variables or values, requires hooks be created within it in order to work effectively.
An example would be adding them in counter components which allow users to update by entering new values or variables into its value field.
Create a button that increments a counter when users press it; or enable this capability by pushing an existing button.
A second example would involve input fields which could be updated by entering new values for them within components.
The third example shows objects which can be updated by adding values to individual components.
A counter is created so users can print its value using “used”, while using the “ok” method it returns an array with initial value entered and prints out its values as they change.
Uses of Hooks in React
Hooks can be an indispensable resource when used within React components to manage state, encapsulate logic and optimise performance while creating cleaner code.
These hooks can be useful in various circumstances, including managing state in components, creating side effects and optimizing performance across multiple components, using context APIs to pass data down into child components, as well as taking advantage of useContext hook to consume context within components.
Some main rules include only calling hooks at the top level of a functional component rather than inside it, for instance.
Utilizing state hooks such as useReducer will ensure your components work effectively and efficiently.
State hooks allow for more complex scenarios to be managed efficiently.
However, if you need to perform side effects after rendering such as retrieving data from an API callback endpoint, an effect hook is an ideal way to perform these actions post-rendered.
Reusability of hooks is another significant advantage associated with their usage.
If a piece of logic needs to be reused across various components, you can create a hook to store that logic and minimize reuse across them all.
Furthermore, performance optimization can also be accomplished using performance hooks like callbacks or memoisation that prevent unnecessary re-renders of web pages.
An API that passes data down to child components enables them to take advantage of it in their hooks, ultimately producing cleaner and easier-to-read code compared to class components.
Use of functions, conditions, and hooks in React JavaScript
React JavaScript provides several examples for using functions, conditions and hooks within functions as a way of making code more reusable and manageable. When used together these elements have their limitations (using them with functions only); using regular functions inside other regular functions; as well as hooks inside regular functions themselves can create custom components which behave exactly how expected by developers.
Functions should abide by three basic principles when creating React apps: nesting hooks within loops, using conditions or functions as necessary and verifying proper usage through ESLint via scope plug.
If ESLint has been enabled with its plugin enabled, then you can view React hooks by hovering over each hook.
Or if your code provides access to its hook, this way too gives an indication of React hooks.
Conditionally responding and making calls in their correct order.
Utilisation of arrow functions and regular functions; setting inside functions.
As mentioned previously, when creating a React app it is essential that ESLint be configured properly with specific scope to detect errors quickly and efficiently.
Also important are having both configuration of ESLint as well as plugin configuration set properly as well as having enough hooks BS code available as reference points for debugging purposes.
Utilizing custom hooks in React applications
Utilizing custom hooks can take time for each field; for more efficient execution, extract hooks into their specific states by extracting custom hooks that handle them into one unique hook named “x”.
Eventually this could create one more way in which custom hooks could help streamline processes in React applications.
Hooks use “form.js,” with use inserted before.
Their name convention begins with an “x,” while form state storage occurs under “use form”.
“Use Form” should be used to save initial values into state.
You need to import various React components (for instance: import React from react), which then serve to save initial values into the state.
Initial values play an essential role in applications, being stored in their state.
When using “import from react”, initial values need to be imported as part of that import operation.
By extracting hooks from existing components and storing them in their state, users can quickly and efficiently set values in their applications.
Creating Reusable Components with Custom Hooks
Hooks provide an effective means of quickly handling changes to UI components without producing ugly values, creating custom hooks which can then be reused without sharing their logic with any other codebases.
This allows the creation of multiple forms without sharing specific UI elements between forms, while reuse effects provide another useful option that can be employed across numerous situations; though its usage might take some getting used to.
This function will be called whenever any component re-renders or is rendered, meaning any time this occurs the function will be invoked and can be seen by watching console logs which display that this event took place.
Challenges faced when using a React memo on a component
One challenge when using React memo for components involves its limited rendering when increments change; this issue arises when increments vary frequently causing issues when adding logic into them.
Console.log and console.log renders, along with renderings Plus+ are utilized here, as is using custom hooks multiple times; rendering count is utilized here while keeping logic within this hook is essential to success.
Exporting the function “export can’t render” can be accomplished by specifying that its export cannot use the count method, then pasting or placing its code within either of two commands: “Use Count Renders” or “Use Count”.
Maintaining logic within a “use count” loop can save time by increasing usage by incrementing only when necessary and maintaining unchanged function calls.
Recurring functions should perform the same function each time they’re called; to accomplish this goal, use different names for functions (for instance “export can’t render”) which imply that only when increment is set to zero should components render.
Challenges arise when using React memo on components with constantly fluctuating increments, creating additional strain on resources and performance. Assigning different names to them while maintaining function could improve their performance and efficiency significantly.
Use of the reference hook in JavaScript
One use for JavaScript’s Reference Hook is to store references to components, like an input field or div node, so they can later be referenced within an application.
As an example, you could create a button which, when clicked, focuses the input field; and use its reference within your application. Within your app’s code using userF4 hook you could also create variable named userF4.
This variable can be used to provide references to components in your application, such as input fields. Utilising user F4 hook, for example, you could create a button which focuses input field when clicked – then use that variable within your program as reference!
User F4 is another use for this hook, where the Const keyword allows you to store references to components like an input field or div node and reference that node within your application.
Create a button which, when activated, focuses the input field; use its reference in your application;
JavaScript’s reference hook enables you to quickly create references for components, like an input field or div, within your application and refer back to them later for use within it. By doing this, it provides access to their reference and makes use of its features easier in your code.
JavaScript’s Custom React Hook Concept
To define a React hook using this concept, pass in its ref and measure. Copy and return that rectangle.
Import all required components from the source code, including React, state, ref, and layout. Understand and consider all effects and dependencies related to each layout effect used on a page layout effect; must pass in required dependencies for that effect; also considering literal types as part of dependency lists
JavaScript alerts us that it cannot statically validate a dependency list passed in by a function call, yet cannot verify them statically. To address this, the command followed by periods can be used to turn off every hook for each period and ensure the code runs without issue.
To create a hook, we can substitute whatever referent is passed via div ref with whatever value should be passed into it – thus reaching our desired result more efficiently.
Use layout and React hooks in JavaScript
“Use Layout” allows users to measure component sizes with precision. A custom hook allows further measurement.
Utilized the callback hook, which prevents functions from being created on every render, we begin by passing down our function into Hello component which then renders this function.
After creating the Hello component and setting its simple counter value, creating a state using createState hook follows next. In this state containing simple counter, count/setCount functions are utilized to set and clear counts respectively.
Uses useState hook to pass function down to Hello component and uses increment function for setting count of components in state; and uses set function as count control function in set state component.
Understanding the Basics of the useState Hook for Custom Logic in Forms
Learning about use state is integral in developing custom logic that fits within forms. Utilise State Hooks can assist in outlining and extracting this logic within forms for further use throughout. Essentially these hooks help define, extract and use logic within forms throughout.
One such form is represented by a button with email and password fields that display on it, where event and value settings may equal or surpass event target values.
This logic can then be copied onto event target values for use elsewhere in other logic. For instance, if all fields contain “set email”, set that value to “set password”. Using this approach in other situations to set all fields with password value.
However, it is essential to keep in mind that setting “email” as the default for all fields may not always be the optimal approach. If you wish to set password for all fields simultaneously then use both “set password” and “set email”.
UseMemo Hook
The useMemo hook is an invaluable way of optimizing computed values within applications and helping reduce how often code needs to recompute itself, leading to faster or less complex applications.
To maximize computed values, it is advisable to start out by creating an example without using memo hook and then later adding one as required. A button is used to increase count increment, with its display appearing on a screen.
Calculating Kanye’s count involves gathering quotes from his REST API which contains quotes taken directly from his tweets, retrieving JSON files containing his tweets, and copying URLs with lists displaying each longest word he used in tweets.
Calculating a prominent tweet involves analyzing its characters by counting all words used and total characters contained within each one, plus total words used overall.
Calculation: the most prominent tweet can be identified by dividing its character count by total tweet character count.
UseContext Hook
JavaScript’s useContext hook provides an essential solution when dealing with large states or reducers; it enables you to save users to a context for reuse throughout your app. Especially helpful is keeping track of users stored there!
User context hook is one of the most frequently requested functions within an application, providing an efficient method for you to store users within context and share them across pages of an application – typically an “About” and an “Home” page respectively.
The useContext hook can be an extremely useful way of managing state changes and handling immutable modifications, helping make state maintenance much simpler and manageable. By creating immutable updates for each state and reducer using useContext hook, managing changes becomes much simpler allowing easier management and maintenance of state.
When dealing with complex use reducers and states, this approach proves extremely helpful.
UseReducer Hook in JavaScript
A useReducer is a method which manages various actions within an application by passing them through various reducer elements before activating them with calls back into the app itself.
Initial state of an application is represented as a sort, while its function to manage this state, called a reducer, is then responsible for updating that state according to logic of updating that state.
However, when dealing with more complex states it’s crucial to evaluate the benefits of overuse states. When managing multiple actions within an application at once it helps manage data types effectively by using UseReducer as an effective management tool for handling actions across it all.
UseEffect Hook
The UseEffect Hook in JavaScript serves to emphasize the necessity of understanding closure in terms of implications on language use. A cleanup function allows one to safely unmount or mount components without risk.
Add cleanup logic to the component, then remove it when no longer required. Hello GS is an example of such an intuitive solution which allows users to toggle whether to show or hide its elements as desired based on user actions.
It can also cause unintended disconnections each time it’s used, leading to frequent unmounting and remounting. To address these problems, copy over the code from export and use “react out” function to create “Hello GS”.
When toggled by the user or displayed and then hidden again, this component will show up and go away automatically. By default it displays “hello”, “set”, “hello set”, and “your state”.
Closures may lead to unexpected behavior, including components dismounting and being remounted multiple times.
Conclusion
React Hooks have revolutionised how developers approach functional components by offering more efficient and cleaner ways of controlling state, performing side effects and encapsulating logic.
Utilising hooks such as useState, useEffect, useReducer and custom hooks can enable developers to craft code that is reusable, optimised and maintainable while improving both readability and performance.
Custom hooks offer React applications an opportunity to encapsulate and share logic between components, increasing modularity.
However, developers must abide by the rules of hooks and be wary of potential challenges when using them – for instance when handling component renders or state updates. By making optimal use of hooks effectively developers can build sophisticated yet highly performant React applications easily.
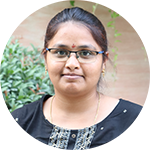
Sai Susmitha
Author
The Journey of Personal Development is a Continuous path of learning and growth.