React Hooks Interview Questions
React Hooks Interview Questions and Answers blog provides an excellent opportunity to discover and deepen your understanding of React Hooks.
While simultaneously serving as an excellent resource for developers who may encounter questions regarding these Hooks during interviews or when working on projects using Hooks.
React Hooks, introduced with React 16.8, offers an effective means for using state and other React features without writing class components directly.
Hooks make writing functional components with complex logic easier while managing state states effectively.
No matter where you stand on React development’s spectrum, I hope the interview questions on the React Hooks blog are informative and beneficial.
Let’s dive in together and learn about React Hooks!
1. What are React Hooks?
React Hooks is a new feature introduced in version 16.8.0 of React, allowing users to use React features without writing a class.
They solve the problem of scattered code across different lifecycle methods and make it easier to understand and reuse complex components.
2. What is the difference between class components and functional components?
Class components extend the Component class and define the component’s behaviour using methods like render.
Functional components return a single element and are determined using the useState () hook.
3. How do you opt into using hooks in React?
You must use React version 16.8.0 or higher to opt into using hooks. Hooks are entirely opt-in and do not contain any breaking changes.
4. What are some benefits of using hooks in React?
Hooks can help in reusing stateful logic without changing the component hierarchy, making it easier to understand and reuse in the future.
They also help split one component into smaller functions based on related code.
5. What are some examples of hooks in React?
Some examples of hooks in React include useState () for adding stateful logic, useEffect () for adding side effects like fetching data, and useContext () for sharing context between components.
6. How do you use hooks in a functional component?
To use hooks in a functional component, you must first import the hook from the React library and call it in the element using the useEffect () hook.
For example, to add stateful logic to a component.
7. How do you use hooks in a class component?
To use hooks in a class component, you must first create a new class that extends the Component class and define methods like render () and constructor ().
You can use the use state () hook inside the constructor to add stateful logic.
8. What is the state hook, and how does it work in React?
The state hook allows users to use state within functional components in React without converting the element to a class component.
The state hook imports the unnamed “use state” from React and returns a pair of values: the current value of the state variable (count) and a method to update the state variable (set count).
9. What are the benefits of using the state hook in functional components?
Organising logic inside a component into reusable isolated units and avoiding trivial bugs and inconsistencies are benefits of using the state hook in functional components.
10. How does creating the project using the Make React app help users understand how the state hook works?
Creating the project using the Make React app helps users understand how the state hook works by providing a clear example of using the state hook to initialise a state variable to zero and create a method to increment the value.
11. What problem do React Hooks solve?
React Hooks is a new feature added in React 16.8.0 that allows users to access React functionalities without creating a class.
They address the issue of code fragmentation across multiple lifecycle techniques, making complicated components easier to comprehend and reuse.
12. Is the transition to hooks backwards compatible?
Yes, Reactuation 16.8.0 is 100% backwards compatible. Hooks do not replace existing knowledge of react concepts.
13. What is array destructuring, and how does it work in the syntax of the use state hook?
Array destructuring is a feature in ES6 that allows you to take the values of an array and assign them to individual variables.
In the syntax of the use state hook, the “use state” import statement is followed by array destructuring, which allows the current value of the state variable (count) and the method to update the state variable (set count) to be assigned to two separate variables, making the syntax more straightforward to understand.
14. What are some advanced topics in React?
Some advanced topics in React include higher-order components (HOCs) and the render props pattern.
HOCs allow you to share stateful logic between components without changing the hierarchy.
The render props pattern will enable you to reuse stateful logic by passing functions as props instead of objects.
15. Can you use the state hook in functional components created before React 0.13?
No, the state hook was introduced in React 0.13, so functional components created before this version cannot use the state hook.
16. What are the two rules for using hooks in React?
The two rules for using hooks in React are:
Call hooks at the top level of your React function and not inside loops, conditions, or nested functions.
Only call hooks from react functions within react function components, not just any regular JavaScript function.
17. When is it appropriate to call a hook in React?
The state variable is initialised with a default value in the app component by not providing any initial value for the count variable.
18. How does the state variable get initialised with a default value in the app component?
The class component in the app component is used to render the counter, which has no functionality.
19. What is the role of the class component in the app component?
The button in the app component handles the “count” variable by rendering the inner text of the button with the new count value when clicked.
20. How does the button handle the “count” variable?
The inner text of the button is updated with the new count value in the app component by calling the set count method, which is a function that takes a single argument (the new count value) and updates the count variable with that value.
21. What is the purpose of the set count method?
The set count method is called inside the arrow function representing the button’s click event handler.
22. How does the set count method work?
The purpose of the set count method is to update the count variable with a new value, which is then displayed as part of the inner text in the browser.
23. What is the significance of the “never used on re-renders” comment in the app component?
The “never used on re-renders” comment in the app component is a comment that is added to the code to explain why the class components with the counter name in the app component are commented out and included.
The comment states that the class components are not used in the app component and were only included for completeness.
24. Why are the class components with the counter name in the app component commented out and included?
The class components with the counter name in the app component are commented out and included because they are not necessary for the app component to work, and removing them can simplify the code and make it easier to understand.
25. What is the difference between a class and functional components in React?
A class component in React is a component that uses the class syntax for creating components, whereas a functional component is a component that is created using the available syntax.
A class component is more versatile and can be used to create components that have a more complex structure.
In contrast, a functional component is more straightforward and can be used to create components that do not require additional functionality.
26. What is the critical difference between calling a hook at the top level of a React function and inside a loop,condition or nested function?
The critical difference between calling a hook at the top level of a React function and inside a loop, condition, or nested function is that a hook can only be called once per component.
In contrast, a loop, condition, or nested function can repeat the same code multiple times.
This means that a hook should be called at the top level of a React function so that it can be used throughout the component rather than inside a loop, condition, or nested function so that it can only be used once.
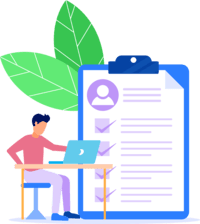
React Hooks Training
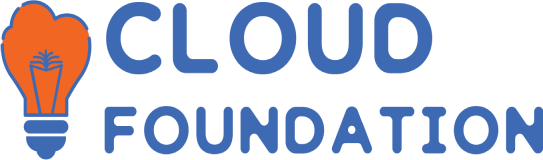
27. Why are these rules crucial for how hooks work in React?
These rules are crucial for how hooks work in React because they help ensure they are used correctly and consistently throughout a React application.
By following these rules, developers can avoid common pitfalls and ensure their code is clear, concise, and easy to understand.
28. What is the use of the setCount function in the counter component?
The set count function in the counter component is used to update the count value based on the previous state value, ensuring that the counter works perfectly fine when updating the state.
This is achieved by accepting a function with access to the old state value.
29. How does the use of state hook work in React?
Using a state hook in React allows you to create, read, and modify a state variable in a functional component.
The hook takes a single argument, an object or a primitive value and returns a state variable and a setter function for that state variable.
30. What are common ways to initialise a state variable in React?
There are several ways to initialise a state variable in React. Some common ones are:
The initial value is passed as an argument to the component constructor.
The initial value is set in the component’s state object.
The default value is set in the component’s state object.
The default value is set in the component’s constructor.
31. How would you create a counter component using class components in React?
To create a counter component using class components in React, you can define a class component with a state variable for the count value and methods for incrementing, decrementing, and resetting the count value.
You can also use the state hook to create the state variable and the setter function.
32. How would you create a counter component using functional components in React?
To create a counter component using functional components in React, you can define an active component with a state variable for the count value and methods for incrementing, decrementing, and resetting the count value.
You can also use the state hook to create the state variable and setter function.
33. How would you use the second form of the setCount function in the counter component to ensure that thecounter works perfectly fine when updating the state?
To use the second form of the setCount function in the counter component to ensure that the counter works perfectly fine when updating the state, you can pass a function as the second argument to the set count function, which has access to the old state value.
This function can calculate the new state based on the old state value and then call the setCount function to update the state.
34. How would you use an object as a state variable in the counter component?
To use an object as a state variable in the counter component, you can create a variable with an object’s default value. This object can have properties that will be initialised to empty strings. The state variable can also include a setter function for updating the state variable.
35. How would you create a class counter component equivalent to the hookcounter3.js file?
To create a class counter component equivalent to the hookcounter3.js file, you can define a class component with a private state variable for the count value and methods for incrementing, decrementing, and resetting the count value.
You can also specify a secret method for calculating the new state based on the old state value and then call the setCount function to update the state.
36. How would you use a dot-jazz equivalent of the increment count function in the class counter component?
To use a dot-jazz equivalent of the increment count function in the class counter component, you can pass a function as a parameter to the increment count method, which has access to the previous state value.
This function can calculate the new state value based on the old state value and then call the setCount method to update the state.
The class counter component can also include a process for getting the previous state value.
37. How would you test the counter component?
To test the counter component, you can create test cases covering different scenarios, such as testing the default state, setCount function, and increment and decrement functions.
You can also try the class counter component equivalent to the hookcounter3.js file and the dot-jazz equivalent of the increment count function.
38. What are some best practices for using state in React components?
Some best practices for using state in React components are:
Keep the state as small as possible, and only update it when necessary.
Use the same setter function for updating the state variable, and be consistent about when you call it.
Use the same function or object to set the state variable; be consistent about how you put it.
Use the same function or object to get the state variable, and be consistent about using it. Use the same function or object to calculate the new state value, and be consistent about
39. What happens when you call the render function with the given input?
The render function will display the first name input with “dot” followed by the last name input, and the first name field will be set to “name dot first name”, and the last name field will be set to “name dot last name”. Then, the last name will be empty.
40. How are the app. chess component’s first and last names updated?
The first and last names are updated by setting the value attribute of the corresponding input fields and then using the on-change event to update the state variables.
The useState hook is used to set the state manually, while the setState function merges the state.
41. Using the spread operator, how is the first name field overwritten in the third example?
The first name field is overwritten in the third example using the spread operator instead of directly assigning a new value to the first name property.
This allows the spread operator to overwrite the existing first name field with a different value.
42. How do the setter functions provided by the useState hook and the setState function differ in behaviour?
The setter functions provided by the state hook do not automatically merge and update the objects in their respective components.
Instead, they must be manually connected and passed to the setter function to update the state variables.
The setState function, on the other hand, combines the state, allowing updated values to be automatically applied to the state variables.
43. What does the setItems() setter function do in the code snippet?
The systems setter function appends a new object with a randomly generated number between 1 and 10 to the current items array.
44. What is the use of a state hook in functional components?
A state hook allows adding a state to functional components and returns an array with two elements: the current state value and a state setter function.
45. What is the purpose of passing a function to the setter function when creating an array in the state hook?
Passing a function to the setter function allows the new state value to depend on the previous value, ensuring the expected behaviour.
46. How does the spread operator help update the state variable in the given code snippet?
The spread operator is used to copy all items in the current items array and then appends the new objects with the random number generated by the setItems setter function.
47. How does using a state hook impact the rendering of items in the JSX URL tag?
Using a state hook ensures that a single piece of state is maintained in the component, allowing consistent behaviour and avoiding state mutations in functional components.
48. How does using a state hook help keep the component’s state consistent?
Using a state hook ensures that the component’s state is consistent by returning an array with two elements: the current state value and a state setter function.
This allows the state to be easily updated and ensures that the application behaves consistently.
49. What is the purpose of the “setItems” function in the given code snippet?
The “setItems” function updates the state variable with the new items added by the button.
50. How is the “items” array passed as a prop to the “Item” component?
The “items” array is passed as a prop to the “Item” component by declaring it as a prop in the “Item” component.
51. How does the spread operator help in handling objects and arrays in the given code snippet?
The spread operator is used to copy all items in the current items array and then add the new objects with the randomly generated number and the “items” array.
This is helpful when working with objects and arrays, ensuring consistency and correct behaviour.
52. What is the benefit of using a functional component with a state hook instead of a class component with astate variable?
Using a state hook in a functional component allows adding an array of items as a state to the element, making it easier to manage and update.
Additionally, available components have more benefits, such as being more concise and accessible to reason.
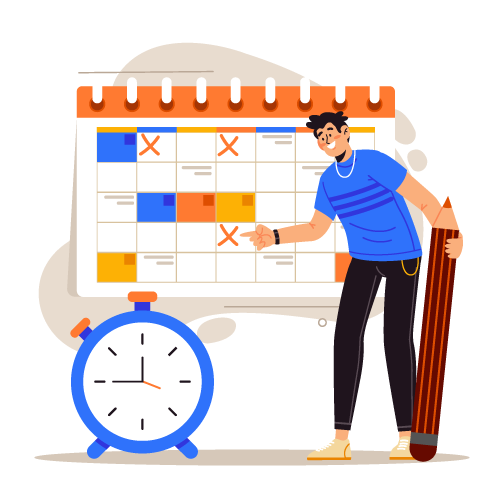
React Hooks Online Training
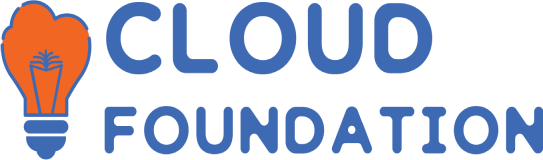
53. What is the Use Effect hook, and how does it allow for side effects in functional components?
The UseEffect hook is a React hook that allows for side effects in functional components.
It addresses the issues of componentDidMount, componentDidUpdate, and componentWillUnmount.
UseEffect handles these lifecycle methods and allows for side effects in functional components.
54. How can we use the Use Effect hook to set the document title on the initial render of a component andsubsequent renders when the component updates?
We can use the Use Effect hook to create an effect that sets the document title whenever the component updates.
55. How can we mimic componentDidMount using effect and functional components?
We can mimic componentDidMount by creating an effect that runs only once when the component mounts.
We can achieve this by passing an empty array as the second argument to the use effect hook.
56. How is the mouse position tracked in a class component using componentDidMount?
The mouse position is tracked in a class component using an event listener added in componentDidMount.
The event listener listens to the mouse move event and logs the position in the state variables.
This event listener is set up only once in componentDidMount.
57. How can we implement the same mouse tracking functionality in a functional component using effect?
In a functional component, we can implement the same mouse tracking functionality using useEffect by creating a state for the mouse position and using useEffect to update the state whenever the mouse moves.
58. What is the effect hook in React, and what are its uses?
The effect hook is a feature in React that enables the creation of side effects in functional components.
It is used to manage lifecycle methods such as component did mount, component did update, and component did unmount.
59. How is the effect hook used?
The effect hook is used to mimic the component that is mounted and the component that is updated in functional components.
The component initialises the count value to zero and sets the document title to the count value using the effect hook.
The document title is then updated with the counter value whenever the component renders.
60. Why is using the effect hook in the example more efficient and effective?
In the example, the effect hook allows the component to be called only once on the initial render and forgotten about when the mouse is moved around. This approach avoids unnecessary re-renders and improves performance.
61. How can a simple counter be created in a functional component using the effect hook?
To create a simple counter in a functional component, import the useState hook and create a state variable and corresponding setter function.
In the JSX, add a button with a click handler to increment the count.
Then, use the effect hook to change the document.
62. What is the purpose of using the effect hook in hook counter one dot JS?
The effect hook is used in hook counter one dot JS to execute side effects after every component render.
It allows easy access to the component’s state and props without writing additional code.
63. How does the effect hook run in a functional component?
The effect hook runs after every render in a functional component, enabling the execution of desired side effects.
64. How does a class component with a state variable for a counter work?
In a class component, a state variable named count is initialised to zero.
When the count value increments, the component re-renders, causing the document title to update to the new counter value.
65. How is a text input implemented in a class component?
An empty string is initialised as the state variable for a text input in a class component.
An input element is added to the JSX, and an on-change handler is added to capture the input element value.
The component updates the state variable when the text changes, adding a log statement to edit the document title.
66. What is the advantage of using the effect hook over class components for side effects?
Using the effect hook in functional components is a straightforward and efficient way to achieve desired side effects, as it eliminates the need to write additional code found in class components.
67. What is the purpose of conditionally executing an effect in React?
Conditionally executing an effect helps optimise the application by preventing unnecessary re-runs of the impact when the values it depends on haven’t changed between renders.
68. What happens if the values in the array passed to the effect don’t change between renders?
The effect is not run, which is an optimisation technique.
69. What is the issue with the given component’s use of the effect hook and RFCE snippet?
Despite unmounting the component from the DOM using the RFCE snippet, the event listener is still logged, indicating a memory leak in the application.
70. What is the purpose of returning a cleanup function from a hook in React?
Returning a cleanup function from a hook in React is to execute code when the component unmounts.
This can include logging statements, removing event listeners, cancelling subscriptions, or other necessary actions to prevent memory leaks or unwanted side effects.
71. What happens when a component unmounts and a cleanup function is not returned from the useEffect hook?
When a component unmounts, and a cleanup function is not returned from the use effect hook, any resources or event listeners created during the component’s lifecycle will not be cleaned up.
This can lead to memory leaks or unwanted side effects.
72. What are the common mistakes beginners make when using hooks or class components to implement asimple counter that automatically increments every second?
The common mistake beginners make when implementing a simple counter that automatically increments every second using hooks or class components is not clearing the interval timer when the component unmounts.
This can lead to memory leaks and unwanted side effects.
73. What examples of resources or event listeners should be cleaned up when a component unmounts?
Examples of resources or event listeners that should be cleaned up when a component unmounts include timers, subscriptions, and event handlers. Failing to clean up these resources can lead to memory leaks and unwanted side effects.
74. What is the significance of the example about a simple counter that automatically increments every second?
The example of a simple counter that automatically increments every second is significant because it illustrates the importance of cleaning up resources or event listeners when a component unmounts.
In the case of the class component implementation, not clearing the interval timer can lead to memory leaks and unwanted side effects.
The example also highlights the benefits of using hooks and returning a cleanup function from the use effect hook to make component cleanup more accessible and efficient.
75. What is the issue with the hook counter-example causes the counter not to increment every second?
The problem is that an empty dependency list was specified in the use effect hook, causing React to ignore watching for changes in the counter variable.
76. Why should the dependency array, in effect, be seen as a way to let React know about everything that theeffect must watch for changes?
The dependency array tells React which values the effect depends on so that it can be re-run when those values change.
77. What should be done to fix the counter issue in the hook counterexample?
The counter should be set up once on the initial render and destroyed after every render. Add a variable to the dependency array if you want to watch it.
78. Why is it recommended to define a function within useEffect when calling it?
Defining the function within the use effect makes it more visible when reading through it, increasing the likelihood of noticing that a prop needs to be specified as a dependency.
79. What is the purpose of using state and effect hooks in React?
Using state and effect hooks in React aims to group related code into different lifecycle methods while keeping unrelated code together.
Hooks allow for multiple use effect calls within the same component, ensuring the corresponding code is organised and separate from unrelated code.
80. What is the future of data fetching in React?
In the future, a new feature called suspense will be responsible for data fetching in React.
Suspense is a higher-order component that allows you to suspend an element’s rendering until data is brought, making it easier to manage data conveying and handling errors.
React Hooks have revolutionised how we write stateful functions in React, offering an alternative to class components and making managing state and side effects more straightforward for functional components.
In this React Hooks interview questions for experienced blog posts, we addressed some frequently asked interview questions regarding React Hooks, such as their advantages vs class components comparisons and popular Hooks like useState, useEffect and useContext usage examples.
Understanding React Hooks is essential for any React developer as they offer a more concise and flexible means to write component logic.
With this blog post’s information on React Hooks and PDF as your guide, you will be ready for any interview questions related to React Hooks.
Understanding React Hooks is of utmost importance for any React developer, and answering interview questions related to React Hooks confidently can make you stand out in the job market.
We hope this React Hooks questionsblog for freshers has provided a solid basis to build as you further explore React Hooks.
Experience is critical when mastering React Hooks try creating your Hooks for specific use cases and testing different combination Hooks until you gain an in-depth knowledge of them and their capabilities.
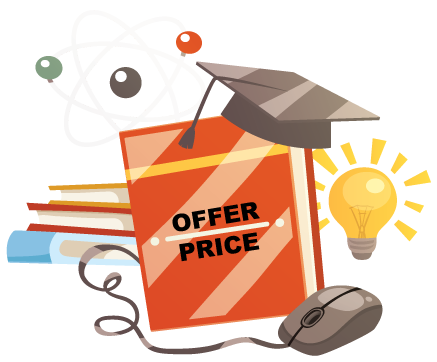
React Hooks Course Price
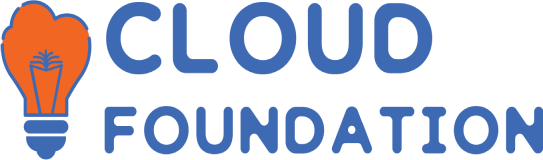
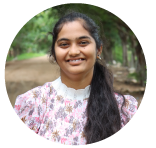
Sindhuja
Author