Python Interview Questions and Answers
Python has proven itself invaluable in numerous industries from data science to web development – its versatility allows programmers of any experience level to utilize its powerful library set and simple syntax; making it perfect for novice as well as experienced coders alike! Let’s review some Python Interview Questions and Answers together…
1. How do I install Python?
To install Python, go to python.org and click on downloads. The latest version of Python is currently 3.7.2, and all materials will apply to the latest version.
2. How do I download PyCharm?
There are two versions of PyCharm available: professional edition (paid) and community edition (free). On Windows, follow the installation wizard and double-click the downloaded PyCharm icon. On Mac, drag and drop the icon onto the applications folder.
3. How do I create a new project in PyCharm?
Right-click on the Hello World folder and open a new Python file named app.py.
4. What are variables in programming?
Variables are fundamental concepts in programming that temporarily store data in a computer’s memory. They can be defined as integers, strings, or Boolean values.
5. What is a string in Python?
A string is a series of characters in Python. It is defined using quotes, and the multiplication operator can be used to draw shapes on the terminal.
6. How do I define variables in Python?
Variables are defined using lowercase letters, but capitalized for special keywords like “false” and “true”.
7. What’s the distinction between single and double quotes in Python?
Single quotes are used to define variables and string literals; double quotations are used to define strings.
8. How do Python strings and variables differ?
A string is a series of characters, while a variable is a temporary storage location for data.
9. What is the square bracket syntax used for in Python?
In Python, the square bracket syntax is used to define many lines of text, for example, a multiline email string. It allows us to start and end a string using triple quotes.
10. How can we extract a character at a given index in a string in Python?
We can use square brackets to extract a character at a given index in a string in Python. For example, if we type “0” to get the first character, we get “P.”
11. In Python, how can we retrieve several characters from a string rather than just one?
In Python, we may use the same technique to extract more than one character from a string.
For example, if we enter “0 colon three,” the Python interpreter will return all characters from this index to the second index, except the character and index three.
12. What does leaving both the start and end index do in Python?
If we leave both the start and end index, we can use the square bracket syntax to copy or clone a string in Python.
13. What is formatted strings in Python programming language?
Formatted strings in Python programming language are used to dynamically generate text with variables.
14. What is the function of curly braces in Python strings?
Python uses curly braces between quotations to define placeholders or gaps in a string.
15. What is the LAN function in Python?
The LAN function in Python is a built-in function that calculates the number of characters in a string.
16. What are some functions specific to strings in Python?
Some functions specific to strings in Python include converting characters to uppercase or lowercase using the dot operator, the find method, the replace method, and the in operator.
17. What is the order of operations in Python?
The order of operations in Python includes exponentiation, multiplication or division, and addition or subtraction.
18. What is operator precedence in Python?
Operator precedence in Python refers to the order of operations.
19. What are some useful built-in functions for mathematical operations in Python?
Some useful built-in functions for mathematical operations in Python include the pow function, the round function, and the random module.
20. What is an if statement used for in Python?
An if statement in Python is used to make decisions based on certain conditions.
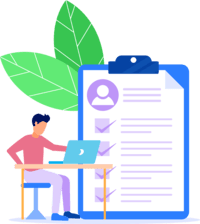
Python Training
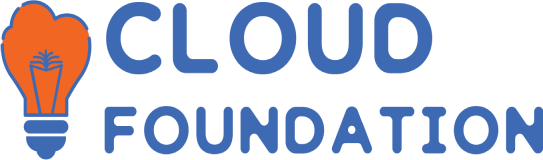
A for loop in Python goes across a string’s characters and performs an operation, the loop variable is set to the current character from the item variable.
22. How do nested loops work in Python?
Nested loops in Python allow us to generate a list of coordinates by adding one loop inside another.
This technique allows us to generate a variety of values and print them on the terminal.
23. What is a F shape using nested loops in Python?
A Python F shape utilizing nested loops requires a list of integers to determine the number of x’s on each line.
To overcome this, a for loop goes over the list and generates one integer each time. Then, an inner loop generates a five-x string.
24. What is a list in Python?
A list in Python is a collection of items, such as numbers, customers, emails, products, or blockposts, that can be defined using square brackets.
25. How do we calculate the total cost of all items in a shopping cart using a for loop in Python?
In Python, we can use a for loop to compute the total cost of all goods in a shopping cart by declaring a variable named total outside of the loop. The total variable is set to zero and incremented with each iteration.
To display the total amount, use either the augmented assignment operator or a prepared string.
26. How do we use a nested loop to generate a list of coordinates in Python?
In Python, we may use a nested loop to create a list of coordinates by stacking one loop within another. In the first generation of the outer loop, x is set to 0, and we are aligned with an inner loop that sets y to zero.
The inner loop runs until y reaches two and then returns to line one. The outer loop then performs the inner loop three times, calculating the total cost.
27. How can we apply a for loop in Python to traverse through a list of numbers?
In Python, we can use a for loop to go through over a list of numbers by creating a variable named numbers that stores the list of numbers.
We can then use the for loop to traverse over each number in the list and do something with it.For example, we can print each number on a separate line.
28. How does Python’s get method work?
Python’s get function retrieves dictionary key values. Keys are given as arguments and their values are returned. If the key doesn’t exist, the application returns none. You may also use a default value like January 1, 1980, to get the key value.
29. What is a function in Python?
A function in Python is a collection of a few lines of code that accomplish a certain purpose, when developing big, complicated programs, it is critical to split down code into smaller, reusable components known as functions.
30. How do you define a function in Python?
Python functions are defined by using the “def” keyword, the function name, and brackets with any parameters. After the brackets, write the function code.
31. How does one call a function in Python?
To call a function in Python, just type its name followed by brackets containing any arguments the function may require.
For example, if you have a function named “greet user” that accepts a “name” parameter, you may invoke it by entering “greet user” followed by a space and the value of the “name” parameter.
32. What is a parameter in Python?
Python parameters are placeholders for receiving information in functions. When calling a function with parameters, we must specify values.
33. What is an argument in Python?
In Python, an argument is the actual piece of information passed to a function when it is called. Arguments are supplied within the brackets of the function declaration.
34. What is a keyword argument in Python?
Python keyword arguments don’t matter where they’re placed. A keyword argument that defines the parameter name and value can pass John as the first name parameter value. This lets us pass the user name without caring about parameter order.
35. What are try except blocks used for in Python?
Python try-except blocks manage errors. They detect and fix certain faults.
36. What should be the name of the function in the emoji converter program?
The name of the function in the emoji converter program should be “emoji underline converter”.
37. What should be the parameter of the function in the emoji converter program?
The parameter of the function in the emoji converter program should be called “message”.
38. What is the task of the “message” variable in the emoji converter program?
The task of the “message” variable in the emoji converter program is to store the input value passed to the magic converter.
39. What is the task of the “result” variable in the emoji converter program?
The task of the “result” variable in the emoji converter program is to store the value returned by the function and then printed to the console.
40. What distinguishes ZeroDivisionError from ValueError?
A ValueError happens when a non-numerical value is introduced into a program that requires a numerical value.
A ZeroDivisionError happens when a software tries to divide by zero.
41. What are comments in Python used for?
Python comments add remarks to programs. They clarify code, connect with other developers, and address errors.
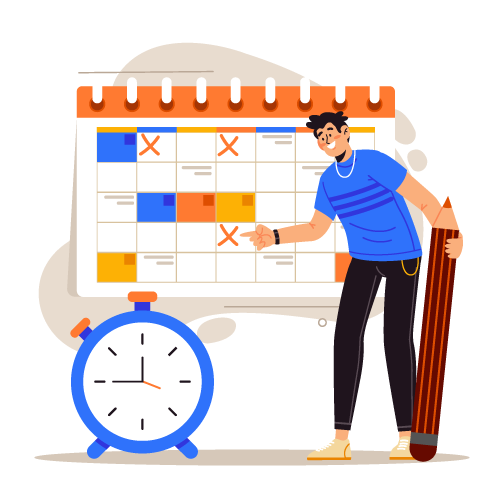
Python Online Training
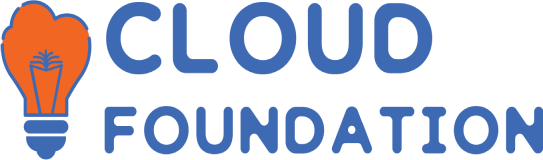
Constructors happen during object creation. Their function is to initialize and set object properties.
43. What is the significance of inheritance in Python?
Python’s inheritance system allows for code reuse. It lets you to construct new classes from existing ones, inheriting methods and properties from the parent class.
44. For what does Python’s module system exist?
Python modules divide code into numerous files like supermarket sections. They enable code fragmentation and reuse in other programmes.
45. What are Python modules?
Organising code and including similar functions and classes requires modules. Python modules include code that may be imported into other Python applications. Modules improve code reuse and organization.
46. In Python, what’s what separates between loading a module and a package?
Python modules can be imported into other Python applications. However, a package contains related modules.
To create a package in Python, use an underscore before the directory name. This makes Python treat the directory as a package.
47. What is the variance between `import` and `from import` in Python?
The ‘import’ statement imports a whole module into your program, whereas the ‘from import’ line imports a particular function or class from a module.
The ‘from import’ statement allows you to use the imported function or class directly in your code, whereas the ‘import’ statement generates a new object that represents the module and provides access to its functions and classes via the dot operator.
48. Why does Jupyter’s describe method exist?
By supplying basic information about each status set column, Jupyter’s describe function simplifies data visualization. Each column’s count, mean, standard deviation, and minimum are returned.
49. What is the aim of Jupyter’s autocomplete and intelligence features?
Jupyter provides auto completion and intelligence, allowing users to easily access information and code snippets while typing.
50. What is Django?
Django is a web framework designed for perfectionists with deadlines, offering a quick, scalable, and secure solution for websites such as Instagram, Spotify, YouTube, and the Washington Post.
It is a collection of reusable modules that support typical activities like processing HTTP requests, URLs, sessions, and cookies.
51. Why does Django have an administrator panel?
The administration panel enables users to manage users and groups, add new users, adjust or remove existing ones, and save data in various fields.
“Test your knowledge with MCQs on Python – the versatile language for data science and automation.”
1. Which programming language is known for its simplicity and readability?
a. Java
b. C++
c. Python
d. Ruby
2. Which of the following is a popular open-source web development framework built on top of Python?
a. Angular
b. React
c. Flask
d. Django
3. Python is commonly used for?
a. Windows desktop development
b. Mobile app development
c. Web development
d. Gaming development
4. What is the name of the popular Python library for data analysis and visualization?
a. NumPy
b. Pandas
c. Matplotlib
d. Scikit-learn
5. Who created Python?
a. Tim Berners-Lee
b. Guido van Rossum
c. Linus Torvalds
d. Mark Zuckerberg
6. What is Python’s most popular library for data analysis?
a. NumPy
b. Pandas
c. Matplotlib
d. Seaborn
7. What is Python’s most popular library for web development?
a. Flask
b. Django
c. Ruby on Rails
d. Express.js
8. What is Python’s most popular library for machine learning?
a. TensorFlow
b. PyTorch
c. Scikit-learn
d. Keras
9. What is Python known for?
a. Speed and efficiency
b. Simplicity and readability
c. Ability to run on multiple platforms
d. Versatility and wide range of applications
10. What is the most popular version of Python?
a. Python 2.x
b. Python 3.5
c. Python 3.7
d. Python 3.9
Conclusion
Python programming interview questions help assess your understanding of its various concepts – being prepared can greatly enhance chances of landing their dream job and furthering a career in this sector of Python development.
Python programming has quickly grown popular over time due to its ease, versatility, and large community of developers. Due to this growing interest in this programming language’s application in development projects, there have been an influx of job openings available for individuals with these skills.
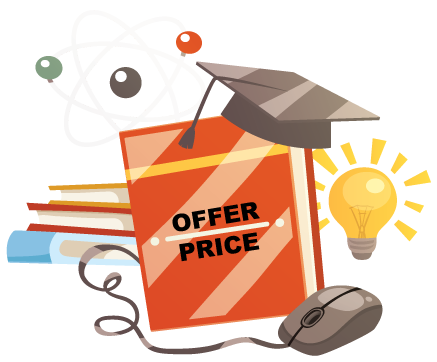
Python Course Price
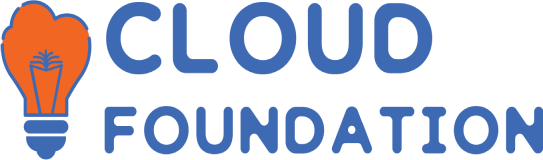
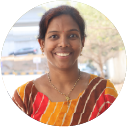
Deepthi
Author