NUnit Interview Questions and Answers
As part of my blog, I aim to assist you in passing an interview by offering expert knowledge of NUnit interview questions and answers.
NUnit is an open-source framework used for unit testing of .NET apps. With simple yet versatile functionality for testing.NET apps, NUnit helps developers identify errors quickly while increasing performance by rapidly and reliably assuring code quality and reliability.
1. What is NUnit?
NUnit is an open-source unit testing framework available across .NET languages such as .NET 5 and the Core Framework.
2. What are the advantages of using NUnit in testing?
NUnit testing tools enable the simultaneous running of tests in any specified order, making for efficient test execution. They are executed via the command line using various parameters for convenient control.
3. How is NUnit structured?
NUnit is decorated as a test fixture and includes a setup method that enables us to set up Selenium WebDriver or database connectivity or open files as necessary.
Teardown methods are utilisedto perform cleanup operations such as closing Selenium WebDriver or disconnecting databases; actual test runs conduct assertion operations.
4. How do you install the Selenium dependency within your project?
Search Selenium on NuGet Package Manager and install its web driver, then follow these instructions:
5. How do you start the Selenium hub?
Execute the command “java -jar” to initiate the Selenium hub start-up process.
6. What do you need to run the Selenium test?
A Selenium hub accepts requests and executes them into client code, depending on which Selenium users have requested tests. Ideally, we would require both the Selenium driver and browser running locally on the local machine to run our Selenium tests successfully.
7. What is the purpose of the Selenium grid?
Selenium Grid allows you to test on multiple browsers and machines simultaneously.
8. How does one set up a test for a local machine?
Its Initial setup involves closing off drivers before running any tests; code should then be prepared, with users then accessing URL, the Lambda tests sample app.
9. How can the code be executed?
Once prepared for execution, right-clicking a test and clicking run test allows users to execute it. In turn, capabilities are received, the browser launches automatically, and the Selenium test completes successfully.
10. What are the first requirements for running a test on the Lambda test platform?
To run tests successfully on Lambda, your initial needs should include capabilities, usernames, and access critical properties outlined in your test scripts.
11. What properties are required to connect to the Lambda test platform?
A series of properties is necessary, including your browser version and operating system and building a name, username, and access key.
12. How are the Lambda test connectivity and local test information obtained?
Lambda test connectivity has been configured, and information on platform versions, names and operating systems has been obtained from the end unit’s test fixture.
13. What is the Lambda test platform?
The Lambda test platform is an internet-connected Selenium grid with over 2000 real desktop devices, mobile viewports and operating system combinations to facilitate parallel test execution.
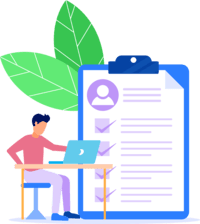
NUnit Training
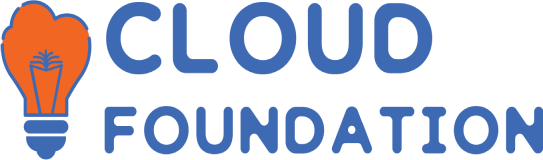
14. What browsers and operating systems will the test run on?
This test will include Chrome, Firefox, and Microsoft Edge browsers running on both a Windows 10 operating system and on both PCs running Linux operating systems.
15. How can the test be run on different environments?
To run tests under various settings, instead of hardcoding the Chrome option to an environment-dependent opportunity, this test method must return browser options based on the browser name passed so testing capabilities against each environment may utilise these capabilities.
16. How can the test be run in parallel?
The parallelisable attribute allows testing to run simultaneously using in NUnit, assign either fixture, children or self as your parallel scope by using the assembly option to set the parallelisable point of in NUnit as a similar scope for any tests in parallel content for your assembly option test run.
17. How does Lambda Test Tunnelling work?
Lambda Test Tunnelling creates an SSH-based integration tunnel between local systems and Lambda Test servers on AWS; this allows testing applications on more than 2000 browser environments on this platform.
18. What is the process of running a local machine-deployed application on the Lambda Test Platform?
Running local machine-deployed apps on the Lambda Test Platform entails starting an HTTP server on local host and deploying their respective application there.
19. What is the purpose of Lambda test tunnelling?
Lambda test tunnelling provides the means for running tests on locally deployed apps using Lambda as part of its platform for test automation and virtualisation.
20. What is the patch object design pattern?
Automated testing employs this design pattern, which permits manipulating an object without altering its state significantly.
21. What is the WebDriver manager?
WebDriver Manager is an application that downloads all required drivers for Selenium. It is an open-source tool for automating web browsers.
22. What is the test class used to automate NUnit project testing?
A test class downloads drivers onto driver managers and initiates tests via WebDriver wait in Chrome browser, invoking private methods to group logic in shorter tests.
23. What main page file is used in the page object pattern?
A main page file consists of actions, assertions and an element map containing elements’ properties and locators. A base class for all pages is constructed; its constructor passes driver parameters while initialising web driver wait timers.
24. What is the main page in the page object pattern?
A main page is an independent class for a website’s homepage that features one public action: adding a rocket with a specific name to a shopping cart.
25. What is the template method in the page object pattern?
A Template Method defines the basic structure of an action and may be overridden by subclasses to provide specific implementation details.
26. What is the map’s purpose in version 2 of the page object pattern?
In version 2, the map serves multiple functions. It allows changes to be locked down more securely as changes come along while at the same time dynamically building an XPath or CSS path and returning elements.
27. What is the data object called purchase info, and what does it hold?
When filling in fields for billing information such as first and last name of billing entities, company, address information, and Metadata fields on forms, it stores all this essential data in one convenient spot for future reference.
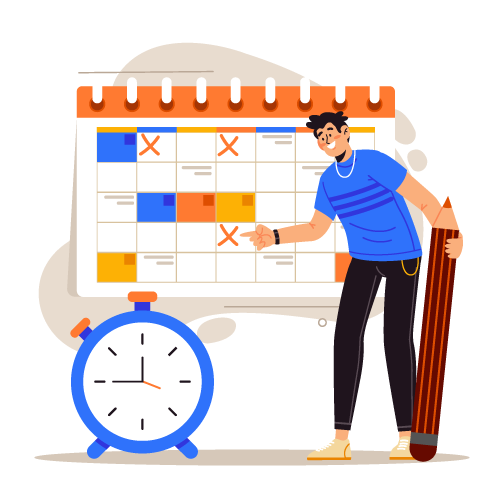
NUnit Online Training
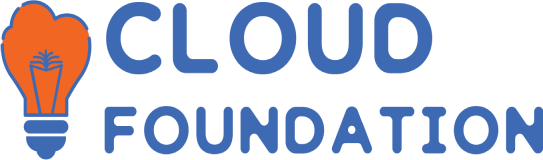
28. How are web pages used in the test?
The test uses web pages by way of composition; these private variables are then initialised using either class initialise, test initialise, or constructor initialise methods.
29. What is the domain-specific language (DSL) used in the tests?
DSL makes tests more readable and manageable by simplifying and comprehending all actions on each page and their details.
30. How is the main page marked as static and partial?
The main page is marked as static and partial using “static” and “partial” keywords; all methods have also been marked partial.
31. What are static assertions?
Static assertions can be directly called from their class as part of it and used to check program state from any method or source; they verify potential errors by testing these assertions against theircondition and can also help verify correct functionality within any test suite.
32. What is not an advantage of using NUnit within a test?
a) Run tests in parallel sequence and order specified,
b) Run tests from the command line with different parameters
c) Perform pass/fail operations with assertions
d) Run tests on any testing platform and within the Lambda test platform
33. What is the output of running a Selenium test using the NUnit framework on local machines and the Lambda test platform?
a) Chrome, version 87.0, and Windows 10 as the operating system.
b) Chrome, version 87.0, and Mac OS as the operating system.
c) Firefox, version 78.0, and Windows 10 as the operating system.
d) All of the above
34. Which of the following is a capability value that can be accessed using the Lambda test capability generator?
a) Chrome version
b) Firefox version
c) Safari version
d) Mac OS version
35. Which is the correct way to run tests on the Lambda test platform more straightforwardly?
A) Use Selenium’s parallel test execution with NUnit parallelisable attribute
B) Use Lambda Test tunnelling for cross-browser cross-platform testing
C) Use a locally hosted application for testing
D) Use a different testing framework for parallel testing
36. How many capabilities can be passed within the remote web driver to connect to the Lambda test platform?
A) One capability
B) Two capabilities
C) Three capabilities
D) Four capabilities
37. Which of the following is the correct way to change the exception from Chrome options to the capability in the test?
A) Replace the existing code with the code “capability = get browser options;”
B) Replace the existing code with the code “capability = get capabilities ();”
C) Create an if condition where the additional capability option needs to be passed
D) Create a new test fixture for Chrome
38. Which is the correct way to run a locally hosted application onto the Lambda Test Platform using Lambda Test Tunnelling?
A) Start an HTTP server and deploy the application on the local machine
B) Download the Lambda Test Tunnelling exe and run the command from the local machine
C) Use a different testing framework for parallel testing
D) Use a different capability for the remote web driver
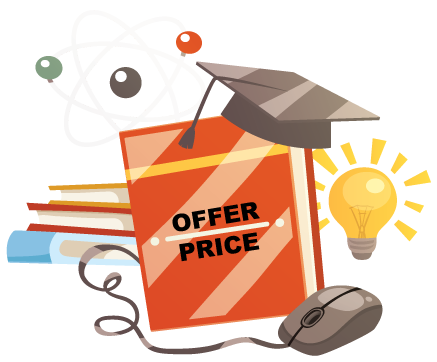
NUnit Course Price
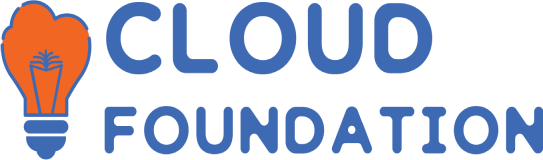
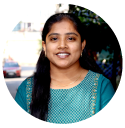
Srujana
Author