JavaScript Interview questions| JavaScript Coding Questions
JavaScript Interview questions!!! Are you in a position to confidently and brilliantly prepare yourself for an interview involving JavaScript
You don’t need to look any further because our comprehensive guide on mastering JavaScript interview questions and answers has got you covered.
With this all-encompassing review, both novices and seasoned professionals alike will be able to efficiently prepare for their upcoming interviews, leaving no place for anxiety in the process.
Move forward with this trip toward professional accomplishment right now; let’s get started.
JavaScript Interview questionsand Answers:
1. What is JavaScript?
JavaScript is a popular and widely used programming language, with companies like Netflix, Walmart, and PayPal building internal applications around it.
JavaScript developers can work as front-end, back-end, or full-stack developers. JavaScript can be used to build full-blown web or mobile apps, real-time networking applications, command line tools, or games.
2. What is the difference between JavaScript and ECMO script?
ECMO script is a specification, while JavaScript is a programming language that confirms it. The organization ECMO is responsible for defining standards.
3. How to write JavaScript code?
A code editor like Visual Studio Code or Note can be downloaded from code.vscode.com. Although not necessary for JavaScript execution, having a node on a machine is beneficial for installing third-party libraries.
4. How to create a new folder and file in Visual Studio Code?
To create a new folder called JS-basics and drag it into Visual Studio Code, and add a new file named Index.html, which generates basic HTML boilerplate for JavaScript code.
Open the extensions tab and search for live server. Install and restart Visual Studio Code. Open the Explorer tab, right-click index.html, and select open with live server.
5. How to console.log. Hello world in JavaScript?
Write the same code as in the last lecture. Console.log. Hello world. This code contains a statement, a string, and a comment. The JavaScript engine will not execute the code, but it is used to document the code. For this demo, add a simple comment, explaining why the code is being written.
6. How to separate concerns in programming?
In programming, the separation of concerns is crucial for separating content from behavior. In real-world applications, we often have hundreds or thousands of JavaScript files, which are combined into a bundle and served to the client.
7. What is the integrated terminal in VS Code?
The integrated terminal in VS Code points to the same folder where we created our files, allowing us to run Node and index.js without explicitly opening a separate terminal window.
8. How to declare a variable called interest rate in JavaScript?
In JavaScript, we can declare a variable called interest rate using camel notation, where the first letter of the first word should be lowercase and the first letter of every word after should be uppercase. This convention ensures that variables are case sensitive and do not have undefined values.
9. What is the difference between a variable and a constant in JavaScript?
In JavaScript, constants are used instead of variables to avoid bugs, such as changing let to const. The default choice for real-world applications is constant, and if needed, let should be used.
10. What are the two categories of types in JavaScript?
The two categories of types in JavaScript are primitives (value types) and reference types. Primitives include strings, numbers, Booleans, undefined, and none. In JavaScript, the type of a variable can be set at runtime, unlike static languages where the type is set and cannot be changed in the future.
11. What is the type of operator in JavaScript?
The type of operator in JavaScript allows for checking the type of a variable, such as adding the name of a variable. However, the type of operator is reserved, so a variable called “type of” cannot be used.
12. How to check the type of a variable in JavaScript?
In JavaScript, the type of an operator can be used to check the type of a variable. To use the type of operator, simply add the name of the variable followed by the word “typeof.” For example, “typeof interest rate” will return the type of the variable interest rate.
13. What is an object in JavaScript?
An object in JavaScript is like an object in real life, as it can hold multiple related variables. For example, a person with two variables, name and age, can be declared as a person object and referenced instead of referencing them.
14. How to declare a person object in JavaScript?
To declare a person object in JavaScript, we start with let or const and set it to an object literal using curly braces. Between these curly braces, we add one or more key value pairs, which are the properties of this object.
For example, to change the name of a person, we can use dot notation, which adds the name of the object and the person to the object.
15. What is the difference between dot notation and bracket notation in JavaScript?
In JavaScript, dot notation uses the name of the object and the person to determine the name of the target property. Bracket notation uses square brackets and a string to determine the name of the target property.
Bracket notation is useful when the user is selecting the name of the target property at runtime, as it can change at runtime.
16. How to use dot notation for in JavaScript?
In JavaScript, dot notation can be used to access the properties of an object, such as age and name. It is cleaner and easier to use.
17. What are arrays in JavaScript?
In JavaScript, arrays are data structures used to represent lists of objects, such as products in a shopping cart or callers the user has selected. These arrays can be initialized and set to an empty array using square brackets, which indicate an empty array.
The length and type of objects in an array can change at runtime, and they can store different types.
18. How to access the length property of an array in JavaScript?
The length property in JavaScript returns the number of items or elements in an array. This property is useful for operations on arrays.
19. What are functions in JavaScript?
Functions in JavaScript are sets of statements that either perform a task or calculate and return a value.
20. What is a parameter in a function?
A parameter in a function is the actual value that is supplied for that parameter. It is an input to the function.
21. What is the difference between a parameter and an argument?
A parameter is the actual value that is supplied for that parameter. An argument is a value that is passed to a function when it is called.
22. What can be passed as an argument to a function?
An argument can be passed to a function as a value.
23. What is the square function in JavaScript?
The square function in JavaScript takes a parameter and calculates the square of that number.
24. How to use the square function?
The square function can be used to initialize a variable, like the number, and display it on the console. It can also be used to pass the square of two to console.log.
25. What are real-world applications?
Real-world applications are collections of hundreds or thousands of functions working together to provide the functionality of that application.
26. How to install JavaScript?
JavaScript can be installed on all web browsers, making it accessible on various devices and operating systems.
27. How to set up my development environment for JavaScript?
To set up your development environment for JavaScript, you can download a code editor like sublime text, Visual Studio Code, or Atom, and create an HTML file with script tags. Open the file in a web browser, and the JavaScript console will appear.
28. What are some popular JavaScript editors?
Some popular JavaScript editors include FreeCodeCamp.org, CodePen.io, and Scrimba.com.
29. What are comments in JavaScript?
Comments in JavaScript are lines of code that JavaScript intentionally ignores, creating notes for yourself and others.
30. What are the seven data types in JavaScript?
The seven data types in JavaScript are strings, numbers, undefined, null, booleans, symbols, and objects.
31. What is the difference between undefined and null?
Undefined means something that hasn’t been defined, while null means nothing.
32. What is a variable in JavaScript?
A variable in JavaScript is a label that allows computers to store and manipulate data dynamically.
33. How to declare a variable in JavaScript?
There are three ways to declare a variable in JavaScript: var, let, and const. var is used throughout the entire program, while let is used within the scope of where it is declared. Const is a variable that should never change and can be changed without an error.
34. What is the difference between clearing variables and assigning variables?
In JavaScript, lines are usually terminated with a semicolon, which is not required but recommended for clarity. For example, declaring a variable as var a and assigning it to var b using the equal sign is a common way to assign values.
35. How to use the console.log function in JavaScript?
The console.log function allows you to see variables at various points in your program.
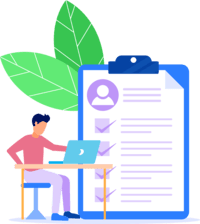
JavaScript Training
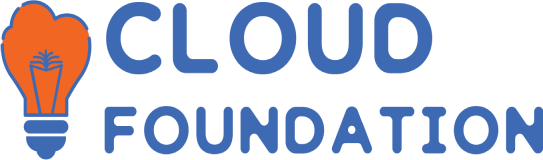
36. What is the difference between function names and variable names in JavaScript?
Function names and variable names are case sensitive, meaning capitalization matters.
37. What are variables uninitialized in JavaScript?
Variables in JavaScript are uninitialized, meaning their value is undefined.
38. How to fix the undefined value of a variable in JavaScript?
To fix the undefined value of a variable in JavaScript, set it to five, set B to 10, and set C to a string.
39. How to use proper camel case and title case over variables in JavaScript?
It is important to use proper camel case and title case over variables in JavaScript to ensure they are correctly defined and assigned.
40. What are some operations that can be performed in JavaScript?
JavaScript allows users to perform various operations, such as adding, subtraction, multiplication, division, quotient, remainder, and division equal.
41. What are some shortcuts that can be used to accomplish variable assignments in JavaScript?
Shortcuts like plus equals and minus equals can be used to accomplish the same task as adding or subtracting a number from a variable.
42. How to use the minus equals operator in JavaScript?
Dividing a variable by a new number and assigning the answer to the variable can be done using the minus equals operator in JavaScript.
43. What are some operations that can be performed in JavaScript on numbers?
JavaScript offers functions for performing addition, subtraction, multiplication, division, and quotient on numbers.
44. What are string literals in JavaScript?
String literals are characters surrounded by quotation marks, which can be single, double, or back text. They are created using JavaScript and can be accessed using the plus operator.
45. How to escape a quote in a string in JavaScript?
To escape a quote in a string in JavaScript, a backslash is used before each quotation mark.
46. How to use back text in a string in JavaScript?
Back text can be used in a string in JavaScript to use single quotes and double quotes within the string.
47. How to execute single quotes and backslashes in a string in JavaScript?
Executing single quotes and backslashes in a string in JavaScript is done by placing two backslashes before the backslash.
48. What are some escape characters in JavaScript?
Some escape characters in JavaScript include new line characters, carriage returns, tabs, backspaces, and form feeds.
49. How to create a multi-line string in JavaScript?
A multi-line string in JavaScript can be created by adding a backslash, tab, and backslash to the first, second, and third lines.
50. What is the RSTR (Raw String) in JavaScript?
The RSTR R string in JavaScript is a long string that contains the phrases “I come first” and “I come second.”
51. How to concatenate strings with variables in JavaScript?
Concatenating strings with variables in JavaScript can be done using the plus equal operator, which adds the variable to the end of the string.
52. What is a versatile tool for concatenating strings and variables in JavaScript?
The RSTR R string is a versatile tool for concatenating strings and variables in JavaScript. It allows for the creation of long strings and can be used to create more complex and meaningful sentences.
53. What is JavaScript used for?
JavaScript is a powerful tool for finding the length of a string.
54. How can the length of a string be calculated in JavaScript?
By adding a first name and using the dot length property, the length can be calculated. For example, the first name dot length will return three, while the last name dot length will return eight.
55. What is zero-based indexing?
Modern programming languages like JavaScript start counting at zero, which is called zero-based indexing. This means that the first letter of the first name will be equal to a.
56. Can strings be altered once created in JavaScript?
Strings are immutable, meaning they cannot be altered once created. However, they can be changed, just the individual characters of a string literal.
57. How can a noun, adjective, verb, and adverb be added to a sentence using the plus equals function in JavaScript?
To add a noun, adjective, verb, and adverb to the sentence, use the plus equals function. For example, to add a noun, adjective, verb, and adverb, use the plus equals function.
58. What is an array in JavaScript?
An array in JavaScript allows you to store several pieces of data in one place, such as an R array. They start with a bracket and end with a comma, and every element is separated by a comma.
59. What are nested arrays or multi-dimensional arrays in JavaScript?
Nested arrays or multi-dimensional arrays can be created by combining elements from one array with another, creating nested arrays. For example, the first element in an array will be an array with a string and a number.
60. What is bracket notation in Python used for?
In Python, bracket notation is used to find specific indexes and strings in arrays.
61. What is double bracket notation used for in Python?
Double bracket notation can be used to select an element in a multi-dimensional or array of arrays. To access an array of arrays or an element within an array, use double bracket notation.
62. What is the push function in Python?
The push function in Python is used to append data to the end of an array.
63. How can the pop function be used in Python?
The pop function in Python removes an item from an array.
64. What is the shift function in Python?
The shift function in Python is similar to the pop function, but it removes the first element of the array instead of the final one.
65. What is the purpose of functions in JavaScript?
Functions in JavaScript allow us to create reusable code by setting up a function with a word function, function name, parentheses, and curly brackets.
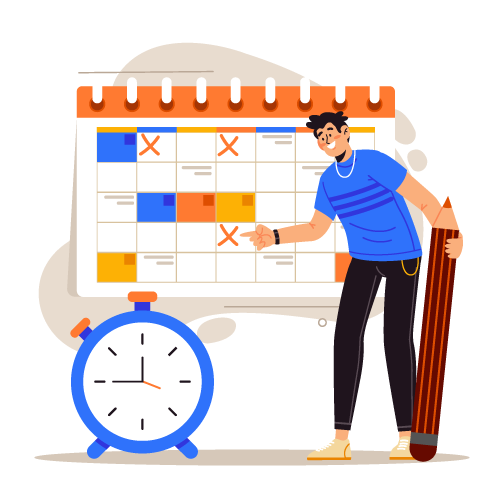
JavaScript Training
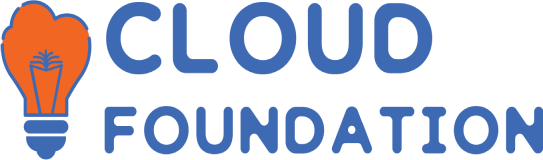
66. What are parameters in JavaScript?
Parameters are variables that act as placeholders for values to be input to a function within the function.
67. How can a function with ARBs be created in JavaScript?
To create a function similar to that function, we can declare a variable called myglobal outside of a function block and set it to 10. This variable can be seen everywhere in the code, even in the function Fun2.
68. What is the concept of scope in JavaScript?
Scope in JavaScript refers to the visibility of variables.
69. What is global scope in JavaScript?
Global scope in JavaScript refers to the visibility of variables defined outside of a function block.
70. How can the type of myglobal be set in JavaScript?
If the type of myglobal does not equal undefined, it will not be undefined. Since this variable is in global scope, the program knows about it.
71. How can a variable be scoped to a function in JavaScript?
If the type of oops global equals undefined, it can be set without using the var keyword. This allows the variable to be scoped to the function, unlike when using the var keyword.
72. How can functions in JavaScript be created with variables and parameters?
Functions in JavaScript can be created with variables and parameters, and using var keywords to set variables without using the var keyword.
73. What is the purpose of using the var keyword in declaring variables?
The var keyword is used to declare variables within a function, allowing them to be accessed anywhere in the program.
74. What happens if the var keyword is not used when declaring a variable?
If the var keyword is not used when declaring a variable, it becomes global automatically, allowing access to the variable anywhere in the program.
75. How can you access a variable outside of a function in JavaScript?
To access a variable outside of a function in JavaScript, you need to delete the variable, ensuring that it is only visible within the function.
76. What is an abstract data structure in computer science?
An abstract data structure is a data structure defined by its behavior, rather than its implementation.
77. What is a queue in computer science?
A queue is an abstract data structure where items are kept in order.
78. How can you simulate a queue using the next inline function in JavaScript?
The next inline function can simulate a queue in JavaScript by adding an item to an array and returning the first item on the list.
79. What is the JSON.stringify function in JavaScript?
The JSON.stringify function is used to convert an array into a string that can be easily printed out.
80. How can you create a new JSON string from an original array using the next inline function?
The next inline function can be used to create a new JSON string from an original array in JavaScript.
81. What is a Boolean data type in JavaScript?
Booleans are data types in JavaScript with only two values: true or false.
82. What is the purpose of using if statements in JavaScript?
The if statement is used to make decisions in code, executing the code in curly braces under certain conditions defined in parentheses.
83. What operators does the equality function use?
The equality function uses the equality operator, double equal sign, and strict equality operator, triple equal sign.
84. What does the equality operator with two equal signs do?
The equality operator with two equal signs performs type conversion, converting the string into a number.
85. What does the strict equality operator with three equal signs do?
The strict equality operator with three equal signs evaluates false, as it does not perform type conversion.
86. How can you use nested if statements to check if two things are true at the same time?
You can use the and operator and two ampersands to simplify the process.
87. What is the purpose of the else if statement?
The else if statement allows for an alternate block of code to be executed when the condition is not true.
88. What is the importance of ordering when using else if statements?
Order is crucial when using else if statements, as the order of the conditions is important.
89. Can if and else if statements be chained to fulfill specific conditions?
Yes, if and else if statements can be chained to fulfill specific conditions.
90. What is the purpose of using nested if statements and the and operator to check if a value is less than or equal to certain conditions?
Nested if statements and the and operator can simplify the process of checking if a value is less than or equal to certain conditions.
91. What is a function in programming?
A function is a block of code that performs a specific task and can be called by other parts of a program.
92. What is a switch statement?
A switch statement is a control structure in programming that tests the value of a variable based on a set of cases.
93. What is the importance of checking spelling errors before proceeding with a test?
Checking spelling errors before proceeding with a test is important to ensure that the test is accurate and produces the correct results.
94. What is the default option in a switch statement?
The default option in a switch statement is similar to an else and if else statement. It is the value that is executed if none of the other cases match the input value.
95. How can a switch statement be easier to write and understand than a chain of if-else statements?
A switch statement can be easier to write and understand than a chain of if-else statements because it allows for all the possible cases to be defined in one place, making it easier to see the logic and structure of the program.
96. What is blackjack card counting?
Blackjack card counting is a strategy used in the game of blackjack to count the number of high cards in the deck and make informed decisions about whether to hold or bet.
97. How can a function be modified to return early from a function with a return statement?
A function can be modified to return early from a function with a return statement by using the return keyword within the function to exit the function and return a value.
98. Can a switch statement test the value of a variable based on the input value?
Yes, a switch statement can test the value of a variable based on the input value by using if statements and comparing the input value to different cases.
99. What are the data types that objects can hold in JavaScript?
Objects can hold a variety of data types in JavaScript, including strings, numbers, arrays, and any other data type.
100. What is the purpose of objects in JavaScript?
Objects are used to group related data together and provide methods to interact with that data in JavaScript.
To summarize, JavaScript is a sophisticated and adaptable programming language that is commonly used in web development and other applications.
It is noted for its simplicity and use, making it a popular choice among developers. JavaScript has become an essential component of online development, its compatibility with all modern web browsers and ability to generate dynamic web sites.
Its popularity and adaptability have also resulted in its extension beyond web development, making it a great tool for a variety of applications.
I hope you will crack the interview.
All the Best!!!
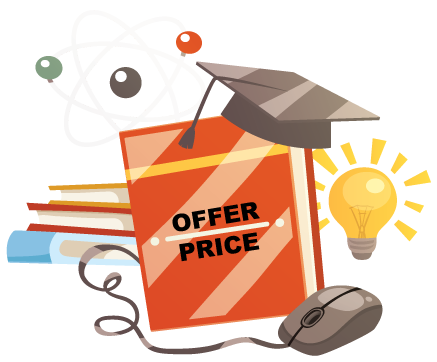
JavaScript Course Price
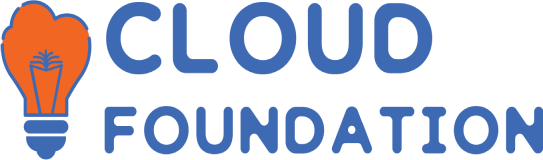
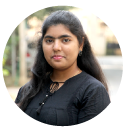
Saniya
Author