Java Streams Tutorial
Introduction to Java Streams
Today’s software development landscape requires excellent performance, flexibility, and ease of use for huge data processing.
As businesses analyse growing datasets, scalable and efficient solutions are needed. Developers may write cleaner, more readable code while preserving performance with Java Streams data processing technology.
Java Streams simplifies collection and massive dataset management with declarative data processing, making it crucial for designing robust, high-performance applications.
What is Java Streams?
Java Streams offer a contemporary, functional method of handling data sets. Streams ease difficult data manipulation chores by allowing declarative reduction, mapping, and filtering operations.
Their support of lazy evaluation and parallel processing helps to effectively handle big datasets.
Using Streams, developers may create easily understood, high-performance, cleaner, more compact code.
Stream
A stream is an abstraction that focuses on the overall picture rather than each individual part.
This explains the concept of a stream in programming, starting with a collection of elements such as arrays, sets, lists, maps, and more.
Later introduces the abstraction phase, which involves telling the logic what it wants without implementing every detail.
Instead of defining and controlling every aspect of the logic itself, It focuses on the stream itself and all available methods.
When a stream is invoked on a list or collection, it can have methods such as filtering down elements, mapping for transformations, and reducing.
These methods are essential for working with the stream and its implementation. The intermediate steps in implementing a stream include filtering down elements, mapping for transformations, and reducing.
These steps help ensure that the code is executed correctly and efficiently.
Process of working with Java streams in Python
The Java streams API was introduced in Java 8 and provides a functional approach to processing bounded streams of objects.
It is designed to take advantage of Java lambda expressions, which were also added in Java.
A stream object is obtained from the list, and for each stream object, a method is called. The method starts the iteration of the elements in the list, and for each element in the list, it executes a Java lambda expression.
The Java streams API is designed to print out the value of each element, which is used in the examples.
For those new to Java lambda expressions, the tutorials in both textual and video form can be found.
The Java streams API is a component that is capable of internal iteration of its elements and provides functionality for the creation of bounded streams of objects.
By using the Java streams API, developers can create more efficient and effective applications, while also leveraging the power of Java lambda expressions.
Java streams provide functionality for processing its elements during iteration. To use a Java stream, follow these steps: obtain a stream, call zero or more streams, and call non-terminal operations on the stream.
Examples of non-terminal operations include calling zero or more and calling a terminal.
Non-terminal operations are not called, and the stream object is configured for later use. When calling a non-terminal operation, it is just a terminal operation, not starting the iteration of the elements.
When configuring the stream for later use, the iteration of the elements is started, and non-elements are started.
These non-terminal operations will kick in, while terminal operations start the internal processing of the elements.
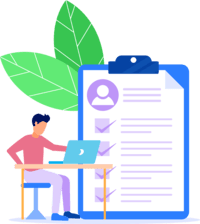
Java Streams Training
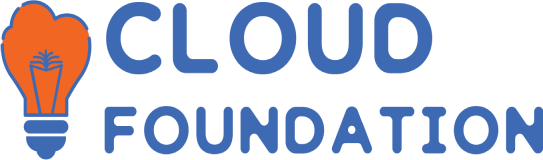
The process of working with streams in Python. It begins by creating a new file and then working with it.
The first step is to create a list array with various elements. Then, uses a list of strings to define names for each element.
The names are then assigned to the list dot and off. This is repeated for each element in the list.
Then uses a collection to invoke the function. For example, to work with a stream named “Zara”, It uses a collection of strings called “Alex”. The function is then used to create a new stream named “Zara” from the original list.
Then uses the “work with streams” method to create a new stream named “Alex” from the original list.
The “work with streams” method is then used to create a new stream named “Alex” from the original list.
Method Map in Java streams
The use of a method map in Python to achieve the desired output of all the names of persons.
The map method is intermediate, meaning it returns a stream after applying a function to all elements.
The convenient aspect is that the stream returned doesn’t have to be of the same data type as the original stream.
For example, demonstrating how to apply a function to all elements and then applying a lambda expression to each element.
The name of each person is then returned, and the output can be saved or output to the console.
It emphasizes the convenience of using the method map in Python, rather than focusing on the original stream or filtering or sorting.
Flat Map
A Python program that uses a flat map to retrieve employee names and data from a stream of companies.
The program uses nested data structures and a getter function to sort the data and filter it based on the company’s start and end.
The program then sorts the data again and filters the results based on the company’s start and end.
The program also saves the data to a string and joins the collection. The output is then output to the console.
The program also performs some operations, such as assigning the data to a variable and displaying the results.
The program is tested and successful, with some errors being reported. The program also includes a few lines of code and a few operations to display the results.
The program is designed to be efficient and efficient, allowing for efficient data collection and analysis.
The program is designed to be user-friendly and easy to use, making it a valuable tool for data analysis and visualization.
Advantages of using Java Streams
Streams of programming are a popular choice for developers due to their ability to produce more concise code, produce shorter methods, and work well with Lambda functions.
However, they may be less efficient in debugging and may be more cumbersome in certain cases.
Despite these drawbacks, streams of programming are generally preferred for their ability to provide a more comprehensible and efficient toolbox.
Overall, both streams and imperative programming offer advantages and disadvantages, but the choice depends on the specific needs and goals of the developer.
The stream way is easy to understand, but it may not be intuitively intuitive. The stream way is also used to filter out all businesses starting with an A run flat map.
The main advantage of streams of programming is that they produce more concise and concise code.
They are often used in conjunction with Lambda functions, which provide a very efficient toolbox.
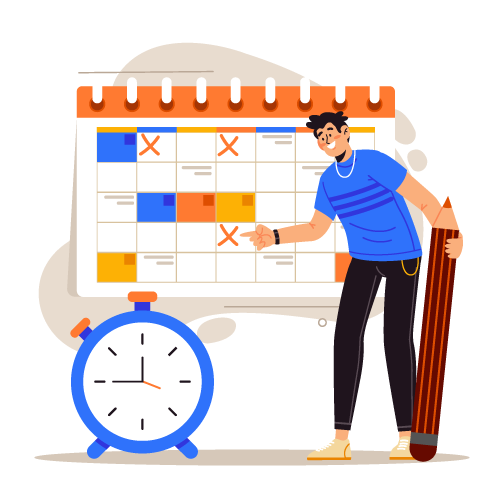
Java Streams Online Training
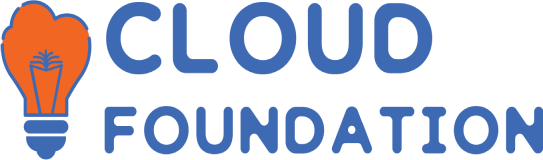
Overview of Python’s various methods and techniques for manipulating data
The Python program that can be invoked from a list to extract data from a stream. The program can then use the built-in ‘streams’ function to extract names, streams, and values from the list.
The program can then use the ‘look’ function to specify the data type and generate a stream of strings.
The ‘look’ function can also be used to generate a list of names, stream, or values. The program can also use the ‘builder’ function to generate a list of values from the list.
The program can then use the ‘methods’ function to extract the values from the list. The program can also use the’ stream’ function to extract the values from the list.
The program can also use the ‘methods’ function to extract the values from the list
The various methods and techniques used in Python to manipulate data. It demonstrates how to use these methods in a concise manner, demonstrating how to concatenate and manipulate streams.
The methods include the use of a map filter, the collection of limits, the sorting of limits, the take while distinct, the sorted and limit functions, the flat map, and the dot operator.
The use of the dot operator, which is used to concatenate multiple streams together. The use of the map filter allows for the collection of limits, the sorting of limits, and the take while distinct functions.
The flat map allows for the concatenation of multiple streams, while the dot operator allows for the dot operation.
The use of the dot operator and the collection of limits. The dot operator is used to concatenate multiple streams, while the collect operator is used to sort and collect the results.
Functional programming language in java
A functional programming language in Java, which involves the use of a map function to take a person and return a person.
The function is implemented using a lambda expression and returns a new person if the input is a person. The method is public static and takes a person as an argument.
The function is then used to create a new person if the input is a person. The method reference is then passed to the map function, which then returns a new person.
The function is useful for those who have never done functional programming with Java. However, if you haven’t done so, this may not make sense to you.
The function is a map function that takes a person and returns a person. The input is a person, and the output is a new person.
The method reference is then passed to the map function, which then returns something. The function is effective because it takes a person and returns something.
If the method reference is removed, the function can be used to pass it to the map function. It highlights the importance of understanding and using functional programming in Java.
By implementing a map function and passing it to the map function, the code becomes more efficient and effective.
Conclusion
Java Streams offer, in essence, a strong and quick method for declarative data processing. They enable actions like filtering, mapping, and succinct reduction of codes, hence simplifying them.
Streams increase performance and readability with tools such lazy evaluation and parallel processing.
Still, one should be aware of possible performance flaws and adverse effects. Java Streams improve efficiency generally and simplify and expressively handle data manipulation chores.
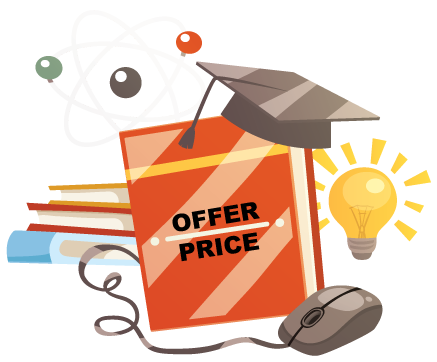
Java Streams Course Price
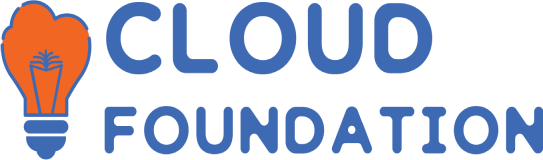
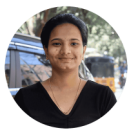
G. Madhavi
Author