Java Stream Interview Questions and Answers
The Java Stream interview questions and answers help you crack the interview and secure a better future, this blog provides you, information about this technology.
Java stream is a powerful feature of the Java programming language that allows for the concise and efficient processing of large amounts of data.
It provides a way to chain together operations on data collections, making it easy to perform complex transformations and manipulations; with Java Stream, developers can write more expressive, readable, and maintainable code.
In this blog, we will explore the Java stream API interview questions.
1. What are streams used for in data processing?
Streams are used for efficiently processing data and can be used in a pipeline to improve data processing efficiency.
2. What are the three parts of a stream pipeline?
The three parts of a stream pipeline are the source (array, collection, or file), intermediate operations (transforming the stream into another stream or the same stream), and terminal operations.
3. Can streams be utilized just once?
Yes, streams can only be used once, so they must be recreated after being used.
4. What are some examples of stream pipelines?
Some examples of stream pipelines include filtering, searching, and counting.
5. How does lazy evaluation work in streams?
Lazy evaluation is a property of streams that allows users to retrieve records only when needed, reducing the number of operations required, in a stream, nothing happens until a terminal operation occurs, which is necessary for operations to occur.
6. What is the pipeline in a stream pipeline?
The pipeline in a stream pipeline specifies the operations to be performed on the source and in what order they occur, allowing JDK to reduce operations whenever possible.
7. What is the difference between streams and collections?
Streams are a data sequence that can be processed using operations, unlike arrays or collections, which are fixed in size and can be accessed randomly.
8. What is the limit terminal operation in Java?
The limit terminal operation is a terminal operation in Java that returns a subset of the stream up to a specified limit.
9. How are streams created in Java?
Streams can be created in Java using the stream of the static method, which creates a stream of strings.
10. What is the filter operation in a stream pipeline?
The filter operation in a stream pipeline filters the stream.
11. What is the purpose of streams in Java?
Streams are lazy because they don’t maintain data but efficiently display large amounts of data.
12. How do we create a list of strings using arrays in Java?
We can create a list of strings using arrays by first creating an array of strings and then converting it into a list using the Arrays.stream() method.
13. What is the arrays dot stream method in Java?
The arrays dot stream method is static in the arrays class, which takes an array as input and returns a double stream of its elements.
14. What is the peak terminal operation in Java?
The peak terminal operation is a terminal operation in Java that returns the maximum value of the stream.
15. How can you stream a collection in Java?
We can stream a collection in Java using the default collection interface method stream ().
16. What is a collection view in Java?
A collection view is a view of a collection that allows us to stream its elements.
17. How do you convert a collection to a collection view in Java?
Convert a collection to a collection view in Java using the StreamViewStream method of the StreamView class.
18. How do you stream from a file in Java?
We can stream from a file in Java using the Files.lines() method takes in a path and returns a stream of strings representing the lines from the file.
19. What is the split method in Java?
The split method splits a string into an array of substrings.
20. What is the purpose of the generate from stream method in Java?
The generate from stream method generates a sequence of values by repeatedly invoking a supplier method that returns an integer between 0 and 9.
21. What is stream dot iterate, and what are its parameters?
Stream dot iterate is a method that generates infinite streams in Java; it takes two parameters: the seed and a unit operator function, a unit operator is a function where the argument and return are the same type, and the input is two, four, six, and so on, and the output is two, four, six, a ten up to 20 even numbers.
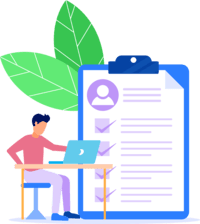
Java Stream Training
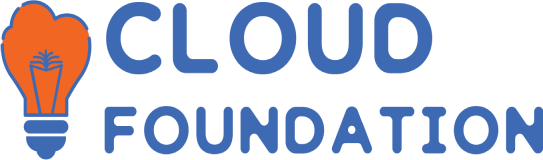
22. What are terminal operations in a stream, and what are their types?
Terminal operations are crucial parts of any stream in Java, as they can be performed without intermediate operations but not the other way around.
They include count, min and max; find any and find first; match, any match and non-match; reduce and collect; find any and find first; any and find first; and find any and find first.
23. What is the count terminal operation in Java?
The count terminal operation is a terminal operation in Java that returns the number of elements in the stream.
24. What is the role of the accumulator in stream processing?
The accumulator combines the current result with the current value in the stream. It takes in two arguments and returns the result of adding them.
25. What is the result of using the reduce method with a stream of strings?
There are three possible results: an empty stream, one element in the stream, or multiple elements.
26. What are the two versions of collecting data from streams?
There are two versions of collecting data from streams: one without a filter and one with a filter, the second version uses an identity, a by-function accumulator, and a binary operator, a combiner.
27. What is the purpose of the combiner in the second version of collecting data from streams?
The combiner, a binary operator, takes in n1 and n2 and returns the result of the addition, it is beneficial in parallel streams.
28. What is a mutable reduction, collect?
A mutable reduction, collect, uses the same object while accumulating, making it more efficient than regular reductions; it allows data to be extracted from streams and into other forms, maps, lists, and sets.
29. What is the purpose of passing predefined API collectors in Java?
The purpose of passing predefined API collectors in Java is to create a map between different objects; these collectors generate a key and a value from the dessert name to the number of characters in the name.
30 How does a two-map work in Java?
In Java, a two-map is used to create a key and a value based on the number of characters, it takes two functions: creating the key and the value.
The key is the string cake, biscuits, or apple tart, and the value is the string itself.
31. How is a tree map created in Java?
A tree map is created in Java by using the last argument to create a new key, which is the string cake, biscuits, apple tart, and cake; the merge function adds the length of the key twice, resulting in eight values for the cake, the method reference tree map colon is also used to sort the keys automatically.
32. What is the purpose of the grouping by method in the collector’s class?
The grouping by method in the collector’s class is a terminal operation that groups elements into a map using a function that determines the keys, each value is a list of entries matching that key, which can be changed to a set.
33. What does Java provide the primitive stream classes?
Java provides an int stream for the primitive’s int, short, byte, and char; a double stream for the primitive types double and float; and a long stream for the primitive types long.
34. What are the three arrays that can be streamed in Java?
The three arrays streamed in Java are an integer array, a double array, and a long array.
35. What are the specific ways of dealing with numerical data in primitive streams?
Some specialised methods for working with numeric data in primitive streams include min, max, sum, and average.
36. What is the merge function used for in Java?
The merge function in Java handles duplicate keys in the mapping and ensures that the values are sorted automatically.
37. What does the get max method do?
The get max method returns the most immense int value.
38. What is the purpose of the get count method?
The get count method helps determine if a stream is empty.
39. What is the “group by” method in Java streams?
The “group by” method in Java streams is a way to group data based on a specific field and extract the data from the resulting map.
40. How can you modify methods within a stream using the dot stream method?
To modify methods within a stream using the dot stream method, one would use “people dot and stream” and pass a lambda expression to perform the desired operation on each stream element.
41. What are the primitive streams?
The primitive streams introduce functional interfaces like double, int, and long consumer types.
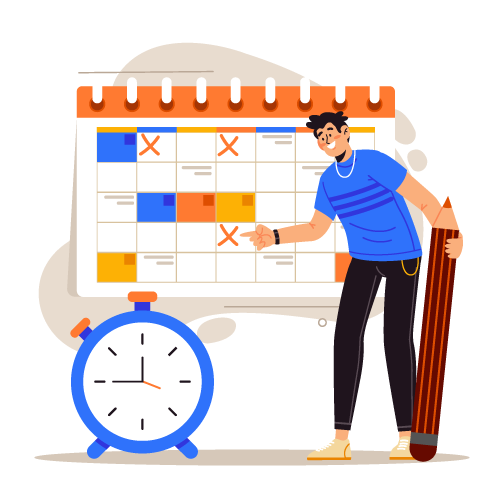
Java Stream Online Training
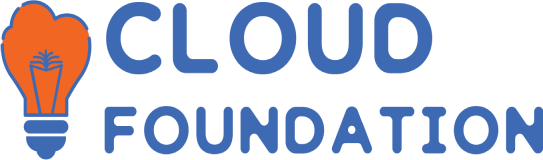
42. What are the double predicate, int predicate, and extended predicate?
The primitive streams introduced new functional interfaces, the double predicate, int predicate, and extended predicate.
43. What is the test in the double predicate, int predicate, and extended predicate?
The test in the double predicate, int predicate, and the extended predicate is a functional method for a double predicate, the int predicate defining a helpful process, and the extended predicate having a test method.
44. What are the binary operator versions for double, int, and long?
The binary operator versions for double, int, and long are applied as double, int, and use as long.
45. What is the Calculate average method?
The Calculate average method is an optional example that calculates an average by dividing the sum by the array’s length; if the option is empty, the get method will execute, and if something is present, the consumer will be completed.
46. What is the map method for streams of predators?
The map method for streams of predators converts a stream of strings into uppercase using a function with input and return type r; the terminal operation outputs the results.
47. What is the map to double method?
The map-to-double method takes a two-double function, applies it as a double, and returns it, and it is used to convert a string stream to a dual stream.
48. How can Java streams allow us to define a list, iterate over it, and apply an if statement?
Java streams allow us to define a list, loop through it, perform an if condition and print using various methods and parameters, it works well with collections and will enable us to output valid values.
49. What is the map-to-long method?
The map-to-long method combines a two-long function and a function interface and converts an extended stream to a long stream.
50. What is the difference between “people dot and then reverse” and “people dot and then comparing”?
“People dot and then reverse” reverses the order of the list, while “people dot and then comparing” uses a comparator to compare the data and sort it in a specific way.
51. How can you find the person with the maximum age using the dot stream method?
To find the person with the maximum age using the dot stream method, one would use “people dot and then stream” and pass a comparator to compare the actual field, such as age.
They would use “max” to find and extract the maximum value of a variable.
52. What is the merge function used for in Java?
The merge function in Java handles duplicate keys in the mapping and ensures that the values are sorted automatically.
53. What is the “map” method in Java streams?
The “map” method in Java streams is a way to transform each stream element into a new value, such as by extracting a specific field or performing a calculation.
54. What is the map to int method?
The map to int method takes a two-int function, giving back an int primitive; it converts a stream of int to a stream of int.
55. What are the most important considerations when using parallel Java streams?
When using parallel streams in Java, the order of thread completion determines the final result.
56. What is the functional method in Java?
The functional method is a method that takes a single parameter and returns a void.
57. What are the RL’s get-takes in Java?
The RL’s get takes in a double supplier, and its functional method is got as double, which returns a double primitive.
58. What are parallel streams in Java?
Parallel streams in Java can process elements in a stream concurrently, and Java achieves this by splitting the stream into sub-streams and performing pipeline operations.
59. What is the difference between sequential and parallel streams in Java?
A sequential stream sums up a stream of numbers, while a parallel stream takes two sub-streams of the same stream and performs additions similarly.
60. What is the dot stream method in Java programming?
The dot stream method in Java programming is a way to perform operations on data collection, such as sorting or filtering, using a stream of objects.
61. What is the clarity approach in Java programming?
The clarity approach in Java programming allows more flexibility in defining steps and implementing specific operations, and one can achieve desired results without depicting implementations using filters and sorting.
Java streams are a powerful feature of the Java programming language that allows for efficient processing of large amounts of data, it is a powerful tool in Java 8 that allows developers to write more concise and efficient code.
They provide a way to process collections in a functional, parallel, and lazy manner, using Java streams, developers can avoid creating unnecessary copies of collections, reduce memory usage, and improve performance.
In this interview question and answer, we covered various Java stream interview questions essential for developers working with Java 8 and beyond.
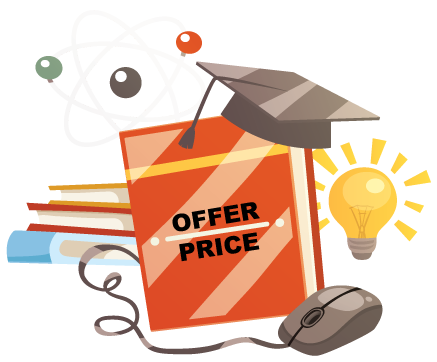
Java Stream Course Price
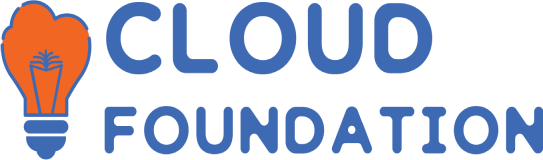
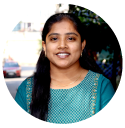
Srujana
Author