JAVA Concurrency Interview Questions
JAVA Concurrency Interview Questions!!! Your progress toward mastery of Java concurrency is commendable.
Our JAVA Concurrency questions blog will provide you the tools you need to understand complicated topics and excel in any interview that pertains to concurrent programming, whether you’re preparing for an exam, interview or just want a better understanding of the subject.
You can now overcome your fears and uncertainties and become a fully proficient Java programmer by delving into Java concurrency programming. Shall we begin?
JAVA Concurrency Interview Questions and Answers:
1. What are the concurrent programming patterns used in Java?
The concurrent programming patterns in Java include using threads, synchronous keywords, atomic objects, semaphores, and futures.
2. What is multithreading in Java?
Multithreading in Java is a concept where two lambdas are executed in separate threads. The first supplier is executed in one thread, and when it finishes, another thread is created to run the following function.
3. What is the role of the get method in multithreading in Java?
The get method is used to obtain the result of the complete chain of two lambdas run in separated threads.
4. Why is it important for software engineers to understand multithreading in Java?
Multithreading is essential for software engineers as it is important for day-to-day work and interview preparation for SD1 and SD2 positions. It is also crucial for system design interviews and often overlooked by many SD1 freshers.
5. What is the difference between a thread and a process in Java?
A thread is a lightweight process that runs within a program, and switching between threads is less expensive than switching between two processes.
Threads share the same address space, and the cost of communication between threads is lower. A process, on the other hand, is a separate instance of a program that runs in memory.
6. What is multitasking within a program in Java?
Multitasking within a program involves multiple threads running within the same program, which can be process-based or thread-based. Thread-based multitasking is used when multiple features or functions occur within a single program.
7. Why is thread-based multitasking less expensive than process-based multitasking?
Thread-based multitasking is less expensive than process-based multitasking because threads share the same address space, and the cost of communication between threads is lower. Switching between threads is also less expensive than switching between two processes.
8. What is multitasking in human behaviour and why is it important?
Multitasking is essential in human behaviour as it allows developers to focus on other tasks while waiting for a particular task to complete. It helps in utilizing idle time effectively and is crucial in both service-based and product-based companies.
9. What is the difference between multitasking in human behaviour and process-based multitasking?
In human behaviour, multitasking allows individuals to focus on other tasks while waiting for a particular task to complete, such as reading a book or participating in meetings.
In contrast, process-based multitasking allows developers to focus on other tasks while waiting for a process to complete, such as packing bags or preparing for an office meeting.
10. What is multitasking in a single-thread environment?
In a single-thread environment, only one task can be performed at a time, and the main function executes sequentially. When the main thread stops executing, the program flows to the program stop.
11. What is a thread in Java, and what is the main thread?
A thread is an independent sequential path of execution within a program, and a main thread is also a thread. In a single-thread environment, the main thread is the only thread running, and when it stops executing, the program flows to the program stop.
12. How can developers create more threads on top of the main thread in Java?
To create more threads on top of the main thread, one must create them manually. However, if multiple threads are needed, they can run concurrently within a program at runtime.
13. What are the important concepts related to multithreading in Java?
The important concepts related to multithreading in Java include creating threads, providing code for execution, accessing common data and code, synchronization, and transitioning between thread states.
14. How can developers create a custom thread in Java?
To create a custom thread in Java, one must do so from the main thread itself, spawning all other threads called child threads.
15. What are user threads and demon threads in Java?
User threads are the ones that execute tasks, while demon threads are the mercy of the runtime system. When there are no user threads running, the program will continue running, regardless of whether the demon threads are running.
16. How can you create a demon thread in Java?
To create a demon thread, you must pass through the method setDaemon() before starting the thread. This method is similar to creating a thread but more complex.
17. What are the two ways to create a user thread in Java?
The first way is to create a class called Thread1 and extend it, then override the run() method. The second way is to implement the Runnable interface or extend the Thread class.
18. What is the difference between creating a thread by extending the Thread class and implementing the Runnable interface?
When you extend the Thread class, you create a new thread class and override the run() method. When you implement the Runnable interface, you create a new class that implements the Runnable interface and provide the code to be executed in the run() method of that class.
19. What is the order of execution between the main thread and a child thread in Java?
The parent-child relationship between the two threads determines the order of execution. The main thread starts first, and the child thread starts when you call the start() method.
20. What are the different ways to create a thread in Java?
There are three ways to create a thread in Javpassing a Runnable interface, extending the Thread class, or implementing the Runnable interface.
21. What is the role of the target object in the thread class?
The target object in the thread class is of type Runnable interface. When the thread is started, it checks if the target object is null. If it is null, the thread does not enter the loop, and the run method is not called. To call the run method, you need to ensure that the target object is not null and properly implemented.
22. What is the difference between passing a Runnable interface and extending the Thread class to create a thread?
When you pass a Runnable interface to create a thread, you create a new thread and send the target (your Runnable instance) to it.
The thread then executes your instance’s run method. When you extend the Thread class and provide your own implementation of the run method, you order the thread to send it to the set.
23. Why is it not possible to extend multiple classes in Java?
Java does not support extending multiple classes. However, if you want to implement other interfaces apart from Runnable, you can do so by implementing the Runnable interface instead of extending the Thread class.
24. Which way is the best way to create a thread in Java?
Implementing the Runnable interface is the best way to create a thread in Java as it allows for multiple implementations for a particular class and does not extend multiple classes.
25. How can you create a thread using lambda and pass the implementation of the run method as a parameter in Java?
In Java, you can create a thread by using lambda and passing the implementation of the run method as a parameter.
The compiler then creates a class that implements the Runnable interface inside the run method, passing the code and instance shaded object of that particular class. This creates a thread, which is then executed by the JVM of that particular thread.
26. What happens when you create a thread by passing a Runnable object in Java?
When creating a thread by passing a Runnable object in Java, the current thread or main thread is created, and a thread object is created.
The Runnable object is passed as a parameter, and the thread name is passed. The start method of that thread returns immediately, but there is a time gap between when the run method of that thread is executed. Once the run method is executed, a new thread begins, and the current thread is executed.
27. What happens when you create an object of a class that extends the Thread class in Java?
When creating an object of a class that extends the Thread class in Java, the start method returns immediately, but after a certain point of time, the run method is executed by the JVM. Once the start method is called, two threads are created – the current thread and the new thread.
28. Why is synchronization important in Java programming?
In Java programming, synchronization is important as threads share the same memory space and can also share objects. This can be beneficial in critical situations where only one thread has access to a shared resource, such as in a movie ticket booking application.
29. How can you create a critical situation in Java?
To create a critical situation in Java, you can create a Stack class and an array inside it. You need to pass the constructor capacity to determine the capacity of this particular stack. The stack top index will be set to minus one, and two methods will be used: push and pop.
30. What is the role of synchronized locks in Java?
In Java, synchronized locks are used to ensure that only one thread can access a shared resource at a time. This is achieved by creating a synchronized block within curly braces, which is a critical section of the code.
The synchronized keyword declares the block as a critical section, preventing multiple threads from accessing it.
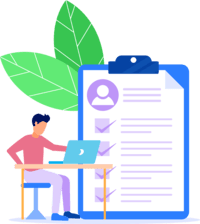
JAVA Concurrency Training
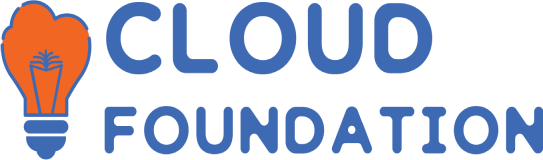
31. How does the synchronized block determine which thread will access it in Java?
In Java, every object can use a synchronized lock, and a primitive int can be used as a lock right. The synchronized block asks for the lock to determine which thread will access it.
When a thread enters the synchronized block, it acquires the lock and holds it until it exits the block. This ensures that only one thread can access the shared resource at a time.
32. In Java, how can objects be used as locks to ensure consistency when multiple threads are accessing the same method?
Objects can be created and passed as locks to ensure that the thread holding the lock is the only one that can access the method. This is particularly important when multiple threads are trying to access the same method.
33. Why are two separate locks needed for push and pop methods?
Two separate locks are needed for push and pop methods to allow two threads to simultaneously run the push and pop methods without having to use different locks for each method. However, to ensure consistency, the same lock should be used for both the push and pop blocks.
34. What happens when a thread is executing the push method and another thread tries to call the pop method?
The push method will not allow the other thread to call the pop method, and the pop method will not allow the thread executing the push method to call it. This ensures that when a thread is executing the push method, no other thread will be able to execute it.
35. What is the difference between synchronized blocks and synchronized methods?
Synchronized blocks make code blocks synchronized, either by enclosing the entire method in a block or by using a synchronized block for specific code within the method.
When using a synchronized block, an explicit lock is required, and the compiler uses the instance of the current object as the lock. Synchronized methods, on the other hand, synchronize the entire method, and the lock used is the instance of the current object.
36. What happens when multiple synchronized methods are declared in a class?
When multiple synchronized methods are declared in a class, the compiler uses the instance of the current object as the lock. This means that only one thread can execute all synchronized methods at a time, even if they have nothing common in between.
37. What is the lock used for in static synchronize methods?
In static synchronize methods, the lock is the class itself, while in non-static methods, the current object reference is used as the lock.
38. Why is it important to understand the concept of synchronize blocks and synchronize methods?
Understanding the importance of synchronize blocks and synchronize methods is crucial for efficient code execution and maintainability in a class environment.
It helps prevent inconsistent state changes between threads and ensures that only one thread can access shared resources at a time.
39. What is the role of synchronization in Java when multiple threads are trying to access a shared resource?
Synchronization is used in Java to ensure that only one thread can access a shared resource at a time.
When a thread enters a synchronized method, it acquires the object lock associated with the object, preventing other threads from accessing the shared resource until the first thread exits the synchronized method and releases the lock.
40. What is the difference between synchronization of static methods and synchronization of instance methods in Java?
Synchronization of static methods is independent from the synchronization of instance methods.
When a thread acquires the lock of a class to execute a static synchronized method, it does not affect any thread acquiring the lock of any object of the class to execute a synchronized instance method.
41. What is the concept of a raised condition in Java?
A raised condition occurs when two or more threads simultaneously update the same value, leaving the value in an undefined and advanced state.
42. What is thread safety in Java?
Thread safety is a term used to describe the design of classes that ensure the state of the object is always consistent even when objects are being used concurrently by multiple other threads.
43. What is the role of the volatile keyword in Java?
The volatile keyword is used in Java to ensure that threads read and write shared variables consistently.
It prevents threads from reading the value of a shared variable from their local cache and ensures that the most up-to-date value is read from the main memory.
44. What is the singleton design pattern in Java?
The singleton design pattern is a design pattern used in Java to ensure that only one instance of a class can be created.
It is achieved by making the constructor of the class private and providing a static method to get the instance of the class. The static method checks if an instance of the class exists and creates it if it does not.
45. Why is the flag instance declared volatile in the singleton design pattern?
The flag instance is declared volatile in the singleton design pattern to ensure that it can be directly updated in the main memory and read by the thread from the main memory.
This prevents multiple threads from creating multiple instances of the singleton class, ensuring that only one instance exists.
46. What is the problem with dividing threads into two groups in multithreaded environments?
The problem is that one group may be trying to push items to a queue while the other group is trying to remove items from the queue, which can lead to inconsistent state and potential errors.
47. What is the singleton design pattern and why is it important?
The singleton design pattern ensures that only one instance of a class can be created. It is important because it helps maintain consistency and prevent potential issues that can arise from having multiple instances of a class.
48. What are the two methods being implemented in the code?
The two methods being implemented in the code are public Boolean add and public int remove.
49. Why do we need to use synchronization when working with the queue object?
We need to use synchronization to prevent multiple threads from accessing the queue object inconsistently and potentially causing errors.
50. What should be placed inside a synchronized block in the remove method?
The remove code should be placed inside a synchronized block to prevent inconsistent state and ensure that only one thread can access the queue object at a time.
51. What should we do if the queue is full and a thread wants to add an item?
We can apply the thread into a queue to potentially unblock it when a space becomes available.
52. What should we do if the queue is empty and a thread wants to remove an item?
We must wait for a condition to be true before removing an item from the queue to avoid an exception.
53. Why do threads need to wait before removing an item from the queue?
Threads need to wait before removing an item from the queue to prevent an interrupted exception and ensure that other threads can access the critical section of the queue without interruption.
54. What happens when an added thread gains access to the queue lock and finds that the queue is full?
Two added threads can enter a waiting state when the queue is full, and both threads try to acquire the lock when an item is removed.
This can lead to a situation where both threads try to acquire the lock at the same time, resulting in unnecessary contention.
55. How can the problem of two added threads fighting for the lock be solved?
Using a `while` loop in both places where the added thread checks for room to add items and checks the wait set can solve the problem. This ensures that the added thread does not add items to the queue when there is no room and maintains consistency.
56. What are the different thread states in Java?
In Java, a thread can be in the new state, ready to run state, dead state, sleeping state, blocked for I/O state, or join completion state.
The new state is when the thread is created, the ready to run state is when the thread is waiting for the JVM to assign it to a processor, the dead state is when the thread has finished execution, the sleeping state is when the thread is waiting for some condition to be met, and the blocked for I/O state is when the thread is waiting for some external event to occur.
The join completion state is when a thread is waiting for another thread to complete its execution.
57. What state does a thread enter when it sleeps?
A thread enters the waiting for notification state when it sleeps.
58. What state does a thread transition into when it gets notified?
A thread transitions into the block for lock acquisition state when it gets notified.
59. How can you determine the current state of a thread in Java?
You can determine the current state of a thread in Java by using the `Thread.State` constant and calling the `Thread.getState()` method.
60. What is the difference between the sleep and wait methods in Java?
The sleep method puts a thread to sleep for a specified time, while the wait method makes a thread wait until it is notified by another thread.
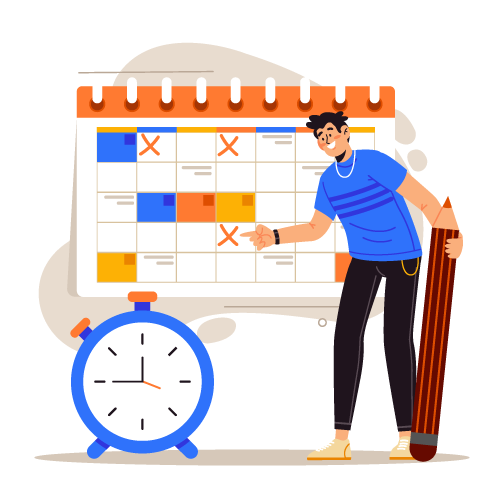
JAVA Concurrency Training
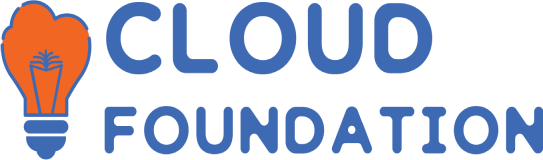
61. What happens when a thread is notified in Java?
A thread transitions into the block for lock acquisition state when it is notified, but it must compete for the lock before it can start executing.
62. What is the purpose of the yield method in Java?
The yield method is an advisory method that suggests the JVM transition a thread from the running state to the ready-to-run state. It is up to the JVM to decide whether to follow the suggestion.
63. What happens when a thread acquires a lock and calls the wait method?
The thread transitions from the blocked state to the wait for lock acquisition state, but it cannot directly start executing until it acquires the lock.
64. What are the three conditions that can wake up a thread in the wait for no difficulties in state?
A thread in the wait for no difficulties in state can be awakened by the occurrence of three incidents: time lapses, interrupted, and another thread interrupting the waiting thread.
65. What state does a thread transition to when it is notified by another thread in Java?
A thread transitions to the block for lock acquisition state when it is notified by another thread.
66. How do you add a thread to the wait set in Java?
You can add a thread to the wait set by calling the wait method on an object and not passing any time parameter.
67. What is interruption in Java, and how does it affect a waiting thread?
Interruption is when another thread interrupts a waiting thread, causing an interrupted exception when the awakened thread finally gets a chance to run. The code invoking the wait method should be prepared to handle this exception.
68. What is the thread.join concept in JavaScript, and how does it work?
The thread.join concept in JavaScript allows threads to execute concurrently but ensures that the main thread does not continue until all child threads have completed their tasks.
This is achieved by creating a new thread, passing a runnable, and executing the main thread. The main thread is blocked until all child threads have completed, ensuring that all tasks are finished before the main thread continues to execute.
69. What is thread joining in Java programming?
Thread joining is a crucial aspect of Java programming that determines the scheduling of threads. It is the mechanism by which a thread waits for another thread to finish its execution before continuing.
70. What is thread priority in Java, and how is it used in thread scheduling?
Thread priority is an important concept in Java that the thread scheduler can use to determine which thread gets a chance to execute.
The thread scheduler gives CPU time to the thread with the highest priority in the ready queue, but there is no guarantee that it will be followed.
Thread scheduling strategies in JVM implementation usually involve the priority-pre-emptive multitasking (PPM) scheduling algorithm, where a thread with a higher priority than the current running thread moves to the ready-to-run state, causing the current running thread to be pre-empted and moved to the ready-to-run state as well.
71. What is the problem with relying heavily on thread priority in Java thread scheduling?
The heavy reliance on thread priority can make a program unportable across platforms due to host platform dependence.
72. What is the time slice scheduling algorithm, and how does it affect thread scheduling?
The time slice scheduling algorithm allows the running thread to run for a fixed length of time after which it moves to the ready-to-run state waiting to be executed again.
This implementation and platform dependence make how threads are scheduled unpredictable from platform to platform.
73. What are new blocks in Java threading, and how do they lead to deadlock situations?
New blocks are paradoxical situations where a thread has a lock and requires the lock of another thread but cannot relinquish its own lock.
This occurs when both threads are waiting for each other to relinquish a lock, leading to deadlock situations.
74. How can a deadlock situation be created in code?
A deadlock situation can be created by having two threads, each with a lock on a different object, and both threads trying to acquire the other’s lock.
This creates a situation where both threads are waiting for each other to relinquish their locks, leading to a deadlock.
75. How can a deadlock situation be cleared?
To clear a deadlock situation, the order in which all threads acquire the lock should always be the same. This can be done by changing the code to ensure that one thread always acquires the lock before the other.
76. What is the importance of understanding how to create a deadlock situation in Java multi-threading?
Understanding how to create a deadlock situation is crucial for successful programming and job interviews in the field of Java multi-threading.
77. What was the difference between single tasking and multi-threading in Java?
In single tasking, a single CPU could handle one task at a time, and the illusion of parallel operation was created by the operating system and CPU working together to switch between tasks.
In contrast, multi-threading allows multiple threads to execute at the same time, leading to improved performance and faster response times in dealing with large amounts of data.
78. What are the challenges of multi-threading in Java?
Multi-threading in Java comes with its challenges, such as managing memory state and avoiding synchronization issues.
If both threads manage the same memory, it can lead to deadlock, race conditions, congestion, and other issues.
79. How can threads be created in Java?
Threads can be created in Java using the thread class and the runnable interface. To create a new thread, create an object of the thread class and use object thread.start.
To implement the run method, create a thread that extends the thread using inheritance concepts or create a new thread with object thread two equal to the new thread and use the runnable interface to create the threads.
80. What is the difference between extending the thread class and using the runnable interface to create threads?
Extending the thread class using inheritance concepts is not recommended as it is not efficient. Instead, create a new thread with object thread two equal to the new thread and use the runnable interface to create the threads.
81. What is the difference between a demon thread and a regular thread in Java?
A demon thread is a background thread that runs without the main thread waiting for it to finish. To set a thread as a demon thread, set the object thread dot three dot set demon and pass it as true.
82. What is the difference between single-threaded and multi-threaded programming in terms of performance?
Single-threaded programming uses a single CPU to handle one task at a time, while multi-threaded programming allows multiple threads to execute at the same time, leading to improved performance and faster response times in dealing with large amounts of data.
83. What are some common issues that can arise in multi-threaded programming?
Some common issues that can arise in multi-threaded programming include managing memory state, avoiding synchronization issues, deadlock, race conditions, and congestion.
Developers must ensure that managing memory state and avoiding synchronization issues is done correctly to avoid problems like data inconsistency and deadlocks.
84. What changes are made to the code to handle data internally in multi-threading applications?
The code is modified to remove the existing thread and create new threads with their own runnable objects.
85. What is the Java memory model, and how does it handle data internally for multi-threading applications?
The Java memory model is a concept that handles data internally for multi-threading applications using a single thread and a shared object.
It consists of local variables and code for each thread, which are not shared among multiple threads, and a shared object that can lead to problems when handling shared objects.
86. What are the local variables in the Java memory model, and where are they stored?
Local variables in the Java memory model are variables that are not shared among multiple threads. They are stored in the respective thread stack, with local one, local two, and local three being local variables for each thread.
87. What is the problem of visibility in multi-threading applications, and how can it be resolved?
The visibility problem is a common issue in multi-threading applications where both threads are dealing with the same object, and the first thread updates the data for that object but leaves it in caching.
This can cause the second thread to get the old value instead of the updated value. The volatile keyword or the synchronized keyword can be used to resolve this problem.
88. What is the difference between the volatile keyword and the synchronized keyword in Java?
The volatile keyword is used to set the flag value from false to true and ensure that the while loop is over and the statement is completed.
It is useful for handling variables that need to be updated frequently and do not require complex locking mechanisms.
The synchronized keyword, on the other hand, can be used for both methods and provides a more robust locking mechanism, ensuring that only one thread can access the shared object at a time.
89. When should the volatile keyword be used in Java, and when should the synchronized keyword be used?
The volatile keyword should be used when handling variables that need to be updated frequently and do not require complex locking mechanisms.
The synchronized keyword should be used when handling shared objects that require more robust locking mechanisms and when ensuring that only one thread can access the shared object at a time.
90. What is the role of the CPU architecture in multi-threading applications?
The CPU architecture plays a crucial role in multi-threading applications as it deals with multicore processors, which handle multiple threads simultaneously.
It also ensures that data flows from the main memory to the caching layer, registers, and finally back to the main memory, and that handling multiple threads in multiple CPUs can be complicated due to the visibility problem.
To summarize, Java Concurrency is an important component of Java programming that enables developers to design fast and scalable programs by employing several threads to accomplish tasks simultaneously.
The Java Memory Model, volatile keywords, and synchronized blocks are some of the tools and features provided by Java to help manage shared data and minimize synchronization concerns in multi-threaded situations.
Understanding these principles and their implementations is critical for efficiently managing multiple threads and ensuring that applications are fast, responsive, and free of data inconsistencies and deadlocks.
Mastering Java Concurrency allows developers to create powerful and complicated systems that can manage massive volumes of data and high degrees of concurrency, making them significant assets in today’s technology market.
I hope you will be shine in your next interview.
All the Best!!!
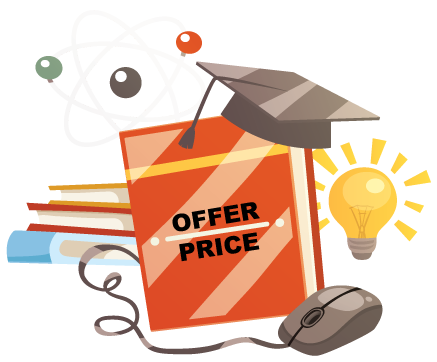
JAVA Concurrency Course Price
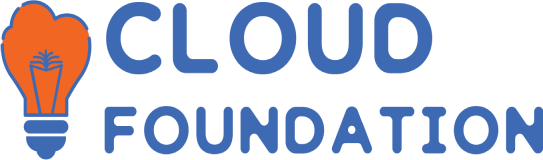
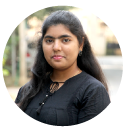
Saniya
Author