Go Interview Questions
Go Interview Questions can help you ace any Go Interview by covering various topics from complex ideas to elementary grammar.
Google introduced its open-source programming language Go (Golang) back in 2007.
Google created Go in 2009 in an attempt to integrate all of the best aspects from various languages like C, C++ and Java into one cohesive system.
Preparing for a Go interview may seem intimidating, no matter your programming experience level. To assist with this daunting challenge, we have assembled a list of frequently askedGo Interview Questions and Answers.
1. What is Go, and what are its use cases?
Go is a programming language created by Google in 2007, designed to simplify writing multi-threaded concurrent applications for modern performance infrastructure.
It is best used for writing high-performance applications that run on cloud platforms and require scalability and resource efficiency.
2. Why was Go created?
Go was created to address the need for a language using modern performance infrastructure, such as multicore processors and cloud computing. It was designed to be simpler and more efficient than existing languages.
3. What are Go packages, and how are they used?
Go packages are collections of Go source code files organised into a single directory tree. They allow developers to modularise their code and reuse it in other projects.
4. How do you start using Go in Visual Studio Code?
To start using Go in Visual Studio Code, you must download and install the Go compiler and an integrated development environment (IDE) like Visual Studio Code.
You should also install a Go extension for better syntax highlighting, navigation, and troubleshooting.
5. How do you create a project and initialise it?
To create and initialise a Go project, you must create a project folder, open a terminal in the project directory, and run the command “go mod init<module name>”. This will make a go. The mod file that describes the project and its dependencies.
6. What is the role of the primary function in a Go application?
The primary function is the entry point of a Go application. It is the first function that gets executed when the program starts running.
7. What is the difference between Go’s “print” and “print ln” functions?
The “print” function in Go prints the output to the console without adding a newline character at the end. On the other hand, the “print ln” function prints the output with a newline character at the end.
8. How do you create variables in Go?
In Go, variables are created using the `var` keyword and the variable name in camel case syntax. To fix an undeclared name error, assign a value to the variable and print it using the `print` or `println` function.
9. How do you track the number of remaining tickets in Go?
Create a variable called `remaining tickets` with an initial value of 50 to track the remaining tickets. Decrease the value each time a ticket is booked.
10. How do you format and print output using `print` in Go?
Use the `%` placeholder in the text string and annotate it with a letter indicating the variable type to be printed. Reference the variable with a comma and its name. Add a new line using backslash n. Reverse the order of placeholders when using multiple placeholders.
11. Why does it require variable types to be defined?
Go requires defining variable types to make the code more robust and prevent errors from being assigned incorrectly.
12. What are the advantages of Go’s error handling?
Go’s error handling allows errors to be detected and highlighted before running the application, preventing errors from being discovered during code execution.
13. How do you define variables and assign them values directly in Go?
Use the variable name followed by the equal sign and the value to create and assign a variable a value in one line.
14. How do you read user input in Go?
Use the `scan` function from the `fmt` package to read user input and save it as a variable.
15. How do you create and define an array in Go?
An array in Go is defined by its size, type of elements, and actual value. The size and type of elements are combined to form the array type.
16. How do you add new elements to an array in Go?
You can add new elements to an array in Go by using indexes to assign values to specific positions.
17. What is the difference between an array and a slice in Go?
An array is a fixed-size data structure, while a slice is a dynamic, resizable data structure that uses a variety under the hood.
18. How do you define and create a slice in Go?
A slice is defined without a size definition, and new elements can be added using the same syntax as in an array.
The slice has brackets around its value and a length of one, automatically expanding as new elements are added.
19. What is the purpose of the loop in the given Go application?
The loop in the Go application is used to simulate booking multiple times for different users, allowing the user information and bookings to be printed out for each user.
20. What is the goal of the code snippet?
The code snippet aims to create a slice of first names from the bookings list and check if the remaining tickets are available for booking.
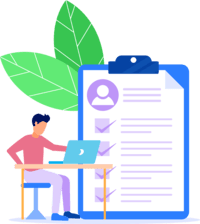
Go Training
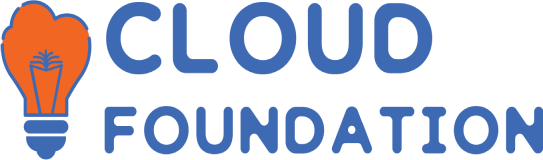
21. What is the purpose of the continued instruction in the application?
The continue instruction is used to skip the current iteration of the for loop and move on to the next one, allowing the user to enter their first name again if they want to book more tickets than remaining.
22. How can the application be made more user-friendly?
The application can be more user-friendly by allowing users to try again with a corrected number of tickets instead of ending the program if they input more tickets than remaining.
23. What is the importance of user input validation in applications?
User input validation is crucial in applications to ensure they don’t crash when users input incorrect data. Developers must check whether user input contains valid information and unexpected values, such as the length of first and last names, email addresses, and user tickets.
24. What validation checks are performed on user input in the application?
The application checks the validity of the first name and last name (with at least two characters), email address (containing the “@” symbol), and user ticket number (positive number less than remaining tickets).
25. How are multiple conditions chained in the application?
The multiple conditions are chained using the `or` operator instead of the `and` operator.
26. What is the importance of using descriptive function names and encapsulating code blocks into functions?
Using descriptive function names and encapsulating code blocks into functions helps maintain a clean and organised application, makes the code more efficient, and avoids errors.
27. How does the switch statement in programming work?
The switch statement is similar to if-else statements, allowing for different application logic based on the selected value. It controls the application flow and executes other logic based on the variable value passed into the switch.
28. What is the difference between a function and a switch statement in programming?
A function is a block of code that can be called and executed when needed, while a switch statement is a way to manage different logic based on the value of a variable.
A function can be encapsulated into a clean and organised code block. At the same time, a switch statement is used to control the application flow and execute different logic based on a selected value.
29. What is the importance of user input validation in applications?
User input validation is crucial in applications to ensure that the user input is valid and unexpected values are handled appropriately. It helps prevent errors and maintain the integrity of data in the application.
30. How can you fix the error caused by a function not recognised by the primary function in Go?
In Go, functions can return multiple values, so you need to modify the function to return the three input parameters instead of assigning them to variables within the function.
31. How are the functions organised in the booking application?
The booking application is organised into three functions: validate_name, validate_email, and validate_ticket_number.
The code for getting user input is extracted and saved into a separate function called get_user_input. The function for booking the application is placed in its function called book_ticket.
32. How can you reduce repetition in the code?
You can define package-level variables outside of all functions, allowing access to them by all tasks in the package. However, you cannot create package-level variables without errors using the provided syntax.
33. Why is defining variables locally in functions essential instead of the primary function?
Defining variables locally in functions helps avoid cluttering the primary function and ensures that logic is encapsulated in smaller functions.
34. How can functions be reused in multiple applications in Go?
Functions can be reused in multiple applications, allowing for the same block of code to be used for various conferences, ensuring consistency and efficiency.
35. How can you organise the code into multiple packages?
Go is organised into packages, which can be one or several files. You can create separate files for each conference booking or share code shared across multiple files.
To use a function from another package, you need to explicitly import that package and use the package name dot function name.
36. How can you export variables from a package?
You can export variables by capitalising their names in the package.
37. Why is exporting functions and variables from a package essential?
Exporting functions and variables from a package makes accessing other application parts easier, promoting code reuse and modularity.
38. Why is defining variables on a package level necessary instead of within a for loop?
Variables defined within a for loop only exist and cannot be used outside. Defining variables on a package level allows multiple functions to access them directly. Global variables can be created using a capitalised variable name and variable scope.
39. Why is a map used instead of a slice to save email addresses and ticket numbers for each user in the bookings list?
A map allows for saving multiple key-value pairs per user, with keys being strings and values being integers. This is useful for storing email addresses and ticket numbers associated with a unique user.
40. How is a map created and initialised in Go?
An empty map is created using the built-in function “make”, which is then used to add all user data as key-value pairs.
To add data, use the name of the map and square brackets, and convert any necessary data types to match the map’s requirements.
41. How is a map added to the bookings list, which is currently a slice of strings?
The bookings list is converted into a slice of maps using the map type instead of the string type using the “make” function. Each user data map is then added to the bookings list.
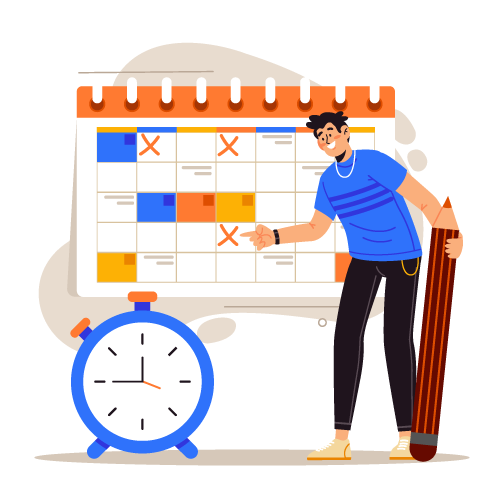
Go Online Training
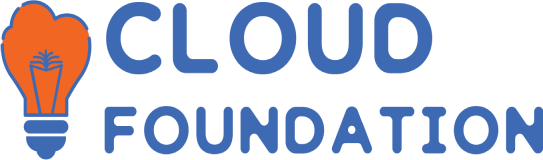
42. What is the syntax for creating and setting values in a struct?
A struct is created using the keyword “struct” and a block with curly braces. The block defines keys or fields with their desired data types.
To develop and set values, use the name of the struct and the dot syntax and assign the desired values to their respective fields.
43. How does using a struct simplify extracting first name values from the bookings list?
Each booking element in the bookings list is treated as a map with key-value pairs for first name, last name, email, and number of tickets.
Using a struct, the first name value can be accessed directly using the dot syntax, simplifying extracting the first name value from the map.
44. How do you use a variable in a welcome message in Go?
To use a variable in a welcome message, define the variable at the beginning of the function and replace the print statement with the variable name.
45. What is the advantage of using a struct instead of a map for organising user data?
A struct provides a predefined structure for user data, making the code cleaner and less messy. It also allows for easier identification of errors in the code by offering suggestions for property names.
46. What is the importance of concurrency in applications?
Concurrency is essential in applications to handle time-consuming processes like ticket generation and sending without interrupting the main application flow.
It helps improve performance and efficiency in applications managing multiple bookings of multiple users.
47. What does it offer for coding concurrent applications?
Go offers the advantage of simplicity in coding concurrent applications. It allows developers to create separate threads for each code line, abstracting the entire thread creation process and improving overall performance.
48. What is a go routine in Go?
A go routine is an abstraction of an operating system thread in Go. It is lighter, cheaper to create, and takes less memory space compared to operating system threads in other languages like Java. This allows for quickly creating and using thousands or tens of thousands of threads.
49. What is the role of channels in Go?
Channels are a built-in functionality in Go that allows easy and safe communication between Go routines, helping to handle concurrency issues.
They enable the sending and receiving of data between go routines synchronised and error-free.
50. What is the benefit of using Go for coding concurrent applications?
Go simplifies coding concurrent applications, allowing developers to create separate threads for each code line without complex configuration, improving overall performance and efficiency.
Test your knowledge with our multiple-choice questions section and check your understanding of Go concepts!
1. Which programming language’s simplicity is the Go language compared to?
a) PHP
b)
c) Java
d) Ruby
2. Go is stated to be best used for what type of applications?
a)
b) Basic scripting and automation in a local environment
c) Calculations-heavy scientific computing
d) Standalone desktop applications with GUI
3. What command initiates the go application into a module or project?
a) go start booking app
b)
c) create a booking app
d) run the booking app
4. What is given as a valid operation concerning constants in Go?
a) Changing the value of a constant during runtime
b)
c) Declaring a constant with a specific type only
d) Constants cannot be used or referenced in the application
5. Why was Go created?
a) Facilitate easier web development for newcomers
b) Replace older programming languages like Java
c) Create a programming language specifically for system-level programming
d)
6. What is a convention for naming variables in the given language?
a) snakeCase
b)
c) PascalCase
d) kebab-case
7. What command is used to execute a Go application?
a) compile main.go
b) go start main.go
c) execute main.go
d)
8. What is a valid operation concerning constants in Go?
a) Changing the value of a constant during runtime
b)
c) Declaring a constant with a specific type only
d) Constants cannot be used or referenced in the application
9. What function from the format package is mentioned to output the value of the conference tickets?
a)
b) Println
c) Fprint
d) Sprint
10.What functions named in Go that control application flow based on conditions?
a) When and unless expressions
b) Condition and given formula
c)
d) Elif and otherwise operators
Hope our comprehensive Go Interview Questions and Answers guide has equipped and encouraged you to succeed at any future interviews.
Acquainting yourself with Go’s core concepts, grammar constructions and industry standards will set you apart from other applicants and demonstrate your knowledge.
Thank you for stopping by our blog as a source to prepare for a Go Interview.
Best wishes on all future interviews and don’t stop studying and practicing!
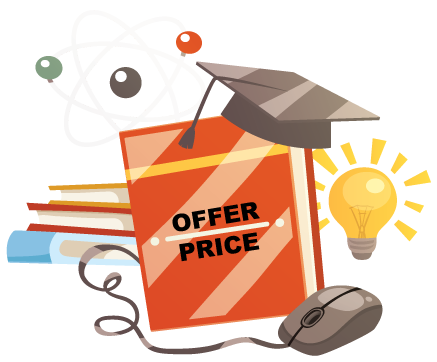
Go Course Price
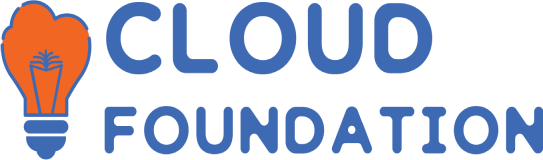
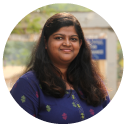
Ankita
Author