Flask Interview Questions and Answers
Flask is an advanced Python microweb framework which is specifically designed to create lightweight online applications quickly and efficiently.
Starting here will get you off on the right foot when learning Flask for either newcomers or experienced developers looking to sharpen up.
Let us work together in understanding and learning Flask together!
1. What is Flask, and why is it an excellent micro framework?
Flask is a micro web framework written in Python. It makes working with back-end web applications due to its simplicity and ease of use.
2. What are some of the features of the Flask?
The Flask will include a user management system, login options, password reset email, account information, updating profile pictures, writing new posts, viewing other people’s posts, updating and deleting posts, and more.
3. What is a virtual environment, and why is it recommended to use one?
A virtual environment is a tool that helps keep dependencies required by different projects separate by creating isolated Python environments. Separating various projects into their virtual environments is recommended to avoid version conflicts.
4. How do you install Flask?
You can use pip by running the command “pip install Flask” in your terminal or command prompt to install Flask. After installing Flask, you can import it into your Python script and create a new Flask application.
5. What is a route in Flask?
A route is a URL we type into our browser to a specific page in our application. Flask uses route decorators to create routes, which are used to add additional functionality to existing functions.
6. How do you use a template in a Flask route function?
To use a template in a Flask route function, instead of returning the HTML code directly, replace the ‘render_template’ function with the name of the template file as the argument.
7. What does the app.route decorator do in Flask?
The app.route decorator in Flask is used to define the URL routes for our application. It handles all of the complicated back-end stuff and allows us to write a function that returns the information shown on our website for that specific route.
8. How do you create a new route and function for the application’s home page?
To create a new route and function for the application’s home page, you can define a new function with a decorator @app.route(‘/’) that maps to the URL ‘/’. In this function, you can write the code executed when the home page is loaded.
9. How do you run the Flask application?
To run the Flask application, navigate to the project directory in the command line and run the ‘flask run’ command. For Windows users, set the environment variable ‘flask\_app’ to the name of the Flask application file and then run the command ‘flask run’.
10. What is debug mode in Flask, and how is it used?
Debug mode in Flask is a feature that allows the server to display additional information when an error occurs. It is helpful for development and debugging purposes.
To run the application in debug mode, set the environment variable ‘FLASK\_DEBUG’ to ‘1’ and then run the application with the command ‘flask run’.
11. How can you update the HTML code in a Flask application?
To update the HTML code in a Flask application, make the changes to the HTML file, save the changes, and then reload the page in the web browser. If the changes do not occur, stop the web server and rerun it.
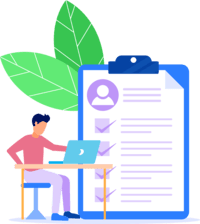
Flask Training
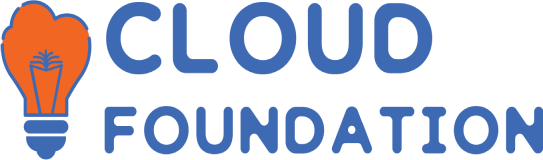
You can use if-else statements within templates to create different HTML structures based on conditions.
13. What is another way to run the Flask application directly using Python?
Another way to run the Flask application directly using Python is to use a conditional statement that checks if the module’s name is equal to ‘__main__’.
This conditional is only valid if the script is run directly with Python, not through the Flask server. To do this, add the conditional statement to the app.run() statement and run the application in debug mode.
14. What is the purpose of using templates in Flask web development?
Templates allow developers to create a more efficient and user-friendly HTML structure for various routes by reducing the amount of repeated HTML tags.
They can be used to create a minimal structure and make it easier to update and maintain the HTML code.
15. How do you create a template in Flask?
To create a template in Flask, first, create a templates directory within the project folder and call it “templates” in Sublime Text.
Then, create templates for each route by creating a new file in the templates directory with the route’s name. Replace the HTML code specific to that route in the function with the name of the template file.
16. How do you create multiple routes that handle the same function?
To create multiple routes that handle the same function, add another decorator with the name of the new route below the existing decorator and change the function name accordingly.
17. What is the advantage of using templates instead of returning HTML code directly in the function?
Using templates instead of returning HTML code directly in the function allows for easier maintenance and updating of the HTML code. It also reduces the amount of repeated HTML tags and makes the code more efficient.
18. How do you pass variables to a template in Flask?
Pass variables as an argument when rendering the template using the ‘render\_template’ function. For example, ‘return render\_template(“home.html”, posts=posts\_list)’.
19. How do you use a for loop in a Flask template?
Use the templating engine to write a for loop within curly braces and two parentheses. For example, ‘for post in posts’.
20. How do you access variables within a template using the templating engine?
Use dot access to access variables within the template. For example, ‘{{ post.title }}’ or ‘{{ post.content }}’.
21. How do you pass a list of dictionaries to a template in Flask?
Pass the list as an argument when rendering the template. For example, ‘return render\_template(“home.html”, posts=posts\_list)’.
22. What is template inheritance in Flask web development?
Template inheritance is a way to avoid repeating code in templates by creating a base template and extending it in child templates. It allows you to keep common elements in the base template and override them with unique content in child templates.
23. How do you create a block within a body tag in Flask?
You can use the block keyword to create a block within a body tag and then create another code block afterwards. The content section is then overridden with unique elements from each page.
24. How do you create a layout template in Flask?
To create a layout template in Flask, create a new file in the “templates” directory with the name “layout.html”. Pick out all repeated sections between your templates and copy the code from one of them into the layout template.
Delete the unique part within the body tag and extend the layout template in child templates using the “extends” keyword.
25. How do you create a block within a body tag in Flask?
You can use the block keyword to create a block within a body tag and then create another code block afterwards. The content section is then overridden with unique elements from each page.
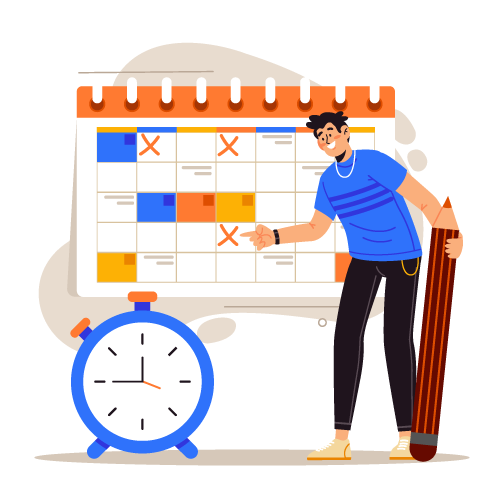
Flask Online Training
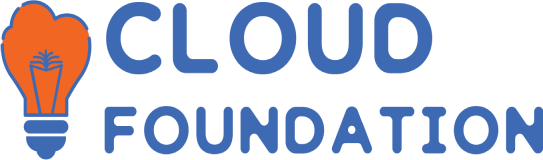
26. How do you create a block within a body tag in Flask?
You can use the block keyword to create a block within a body tag and then create another code block afterwards. The content section is then overridden with unique elements from each page.
Test your knowledge with our fun and challenging multiple choice questions!
1. What command will allow you to run your Flask application?
a) python startapp
b)
c) python app.py
d) flask start
2.Which IP address and port number combo does Flask serve your app by default?
a)
b) 192.168.1.1 and port 8000
c) 0.0.0.0 and port 3000
d) 10.0.0.1 and port 8080
3. To install Flask, what package manager is required?
a) npm
b)
c) yarn
d) gem
4. How do you create multiple routes handled by the same function in flask?
a)
b) Creating a multi-threaded context
c) Defining an abstract route method
d) Using a conditional switch statement
5. In Flask, what function is called to return more complex HTML code to our web page?
a) compose_layout
b) draw_html
c) distribute_template
d)
6. Which snippet of code is copied into location of dummy post data when creating blocks in Flask?
a) Global styles from main.css
b) Start script from server-config file
c) Head section from base.html template
d)
7. What purpose does the Flask command ‘URL for’ serve?
a) Estimating server load
b) Debugging URL endpoints
c) Calculating response times
d)
8. What feature makes it easy to manage and separate blog post presentations?
a) Database normalization
b) JavaScript Engines
c)
d) CSS Transitions
9. Which HTML structure mainly remains intact even after extending Flask templates?
a) Internal script references
b)
c) Client-side validation tags
d) Database connections script
10. What needs to be done to apply global styles from a file named ‘main.css’ in a Flask project?
a) Embed the CSS directly in each HTML header
b) Send the CSS styles through an email
c)
d) Hardcode the styles into the layers of the firewall
Conclusion
This blog covered some of the most frequently asked Flask Python Interview Questions ranging from basics, routing templates and deployment. These Interview Questions on Flask Pytton should help you grasp Flask better as a framework and prepare you for interviews involving Flask.
Hopefully, this blog can assist your preparations for an interview and enjoy Flask coding!
Good luck in your coding career and happy coding with Flask!
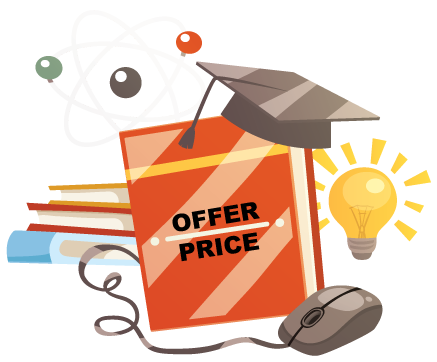
Flask Course Price
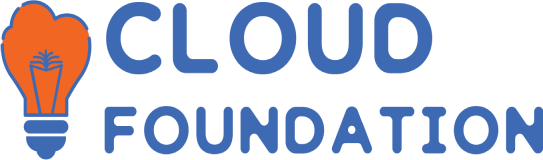
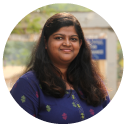
Ankita
Author