Dart Interview Questions
Dart interview questions: This open-source programming language, developed by Google, is known for its fast performance and efficient compilation into JavaScript code.
Dart has become increasingly popular, particularly for developing mobile and online applications.
More firms now include Dart in their technology stacks, making its expertise highly regarded among software professionals.
Whether you’re a new or experienced developer, this Dart interview questions and answers blog provides common Dart interview questions with answers to ensure they ace that upcoming Dart interview!
So, let’s dive right in and prepare to ace that following Dart job interview!
1. What is the blueprint for creating objects in Dart?
The blueprint for creating objects in Dart includes instance variables, function names, and constructors.
2. What is the default constructor in Dart?
The default constructor in Dart is the name, which is not a written type.
3. Can you define named constructors in Dart?
You can define as many named constructors in Dart as needed.
4. What are instance variables and local variables in Dart?
Instance variables are field variables, while local variables are integer marks confined within the method.
5. What are reference variables in Dart?
In Dart, reference variables, such as s1 and s2, assign values to objects and are used to create them.
6. What is the purpose of defining es and objects in Dart?
Defining es and objects in Dart is to define properties and behaviour for each, such as colour, breed, or behaviour.
7. Can you change the default values of instance variables in JavaScript?
By assigning valid values, you can change the default values of instance variables in JavaScript.
8. What is a Growable List in Dart?
A Growable List in Dart is a list whose size automatically increases to accommodate the elements within it.
9. How do you define a variable list in Dart, and what is the persistence of the country’s dot?
You would use the list constructor to define a variable list in Dart.
For example, if you wanted to create a list of string values for country names, you would use the statement List<String> countries = [“USA”, “Canada”, “Mexico”];.
The countries dot (.) separates the elements of the list when defining it.
10. What are the different types of lists in Dart, and what is the purpose of the list constructor?
Dart has two types of lists: fixed-length lists and growable lists.
Fixed-length lists have a fixed size and cannot be changed once created, while growable lists can be resized dynamically.
The list constructor aims to create a new list with a specified length.
11. How are elements stored in a Dart list?
Elements in a Dart list are stored in sequence, each with a unique address or index that can be accessed or read.
The index starts with zero, so the first element is at index zero, the second is at index one, and so on.
12. What is the purpose of the number list and square brackets in Dart, and how are values initialised in a list?
Dart’s number list and square brackets initialise a list with specific values.
For example, List<int> numbers = [73, 64] creates a list of integers with values 73 and 64.
If you don’t provide any values, they are initialised as null.
13. How do you print out values from a list in Dart, the print statement and the list’s index?
To print out values from a list in Dart, use the print statement followed by the list’s name and the index of the element you want to print.
For example, print (numbers [0]); would print the value 73.
14. How do you delete a value from a list in Dart, and what is the use of setting a value to null?
To delete a value from a list in Dart, you access it at the specified index and set it equal to null.
For example, numbers [1] = invalid deletes the value 64 from the list.
Setting a value to null is to remove it from the list.
15. What is the for each loop in Dart, and how is it used to print out values from a list?
There is a way to print out values from a list using a lambda expression for each loop in Dart.
It uses a parameter as an integer value and is used in the method body.
The index prints out the values, and the output is the same as using a regular for loop.
16. What are the predefined options for a list in Dart, and which type of list do these operations apply to?
The predefined options for a list in Dart include the remove, add, and remove-add methods. These operations are not supported in the fixed-length list but only in the growable list.
17. What is a map in Dart, and how is it defined?
A map in Dart is an unordered collection of key-value pairs, which can be of any object type.
The key should always be unique, but the value can be repeated or have a duplicate entry.
Maps are defined by determining a variable of roots and creating it using the map constructor.
18. How are values inserted into a map in Dart?
Values are inserted into a map in Dart by using square brackets and defining the key as a string.
The key should never be repeated, but the value can have duplicate entries.
19. What collections are covered, and how do you create dark maps using lambda expressions for each loop?
The video covers the creation of ordered lists, fixed-length lists, growable lists, unordered sets, and hash maps.
To create dark maps using lambda expressions for each loop, the key is a string, and the value is an integer.
Various operations can be performed on the map, including checking if a key is present, updating the map, and deleting the key and value.
20. How do you perform a read operation on a map in Dart, and how do you print out all the values?
To perform a read operation on a map in Dart, use a print statement with the map and string key.
To print out all the values, use a for-in loop with the variable of key in the map and dot keys.
In this case, the key object is a string, and the values can be used instead of keys.
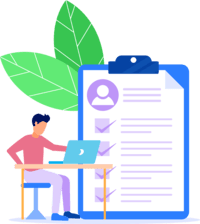
Dart Training
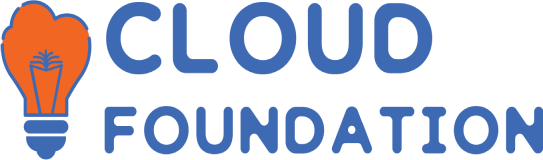
21. What are conditional expressions in Dart?
Conditional expressions in Dart are simple and effective ways to find the smallest number of two numbers.
22. What are switch case statements in Dart, and how are they used?
Switch case statements in Dart execute different code blocks based on various conditions.
They replace multiple if-else statements and make the code more readable and efficient.
23. What are the different types of constructors in Dart, and what are their purposes?
Dart’s different types of constructors include default, parameterised, named, and constant constructors.
They aim to create objects, initialise state, and define custom constructor names.
24. What is a default constructor in Dart?
A default constructor in Dart is a constructor called as if it were a function.
It aims to create an object and initialise its state with default values.
25. What is a parameterised constructor in Dart, and how is it used?
A parameterised constructor in Dart contains a parameter. It creates an object and initialises its state with provided values.
26. How do you use a parameterised constructor in Dart, and what is its advantage?
To use a parameterised constructor in Dart, pass in the value of a parameter and assign it to a variable.
Its advantage is that it reduces the code to a more manageable size.
27. How is a named constructor with parameters similar to a parameterised constructor in Dart?
A named constructor with parameters in Dart is similar to a parameterised constructor as both can add parameters such as dot ID or dot name.
28. How do you create another named constructor in Dart, and what is its purpose?
To create another named constructor in Dart, use the syntax of student, dot, and my custom constructor.
It aims to create another object with a reference variable and a specific value.
29. What is a constant constructor in Dart, and how is it used?
A constant constructor in Dart creates objects and initialises instance or field variables.
It is not commonly used in Dart but is helpful for object-oriented programming.
30. Can you have default and parameterised constructors within the same class in Dart?
No, having default and parameterised constructors within the same class in Dart is impossible.
31. Can you use multiple named constructors within the same class in Dart, and can they be used simultaneously with the parameterised and default constructors?
In Dart, you can use multiple named constructors within the same class, but they cannot be used simultaneously with the parameterised and default constructors.
32. How do you define a named constructor within the of in JavaScript?
A named constructor within the of is defined by calling a constructor from the animal’s parent.
To invoke this constructor from the of, we use the super keyword followed by a dot and the name of our constructor.
33. How does inheritance with the default parameterised and named constructors work in JavaScript?
Inheritance with the default parameterised and named constructors works by calling the super no argument constructor by default if no parameters exist in the super constructor.
If the default constructor is missing in the parent, you must manually call one of the constructors in the super.
34. Can you discuss the custom exception handling in Dart?
Dart’s Custom exception handling focuses on creating a custom exception that implements the exception interface.
It defines a method to print an error message stating that the user cannot enter a negative number.
A method is also created to verify the amount, checking if it is smaller than 0.
If the amount is smaller than 0, it is thrown manually.
35. How can the application crash if the user enters a negative number in a Dart-based banking application?
The application crashes if the user enters a negative number, indicating an unhandled exception.
This crash can be mitigated by using a try block, on-deposit exception, or catch clause to handle this exception.
This allows the error message to be printed and the code executed.
The final can manage the code, regardless of the error.
36. What is the App Start keyword in Dart used for?
The App Start keyword in Dart defines a shape as an App Start.
This allows for creating objects that cannot be instantiated or made with a new expression.
37. What is the advantage of using the App Start syntax in Dart?
Unlike normal functions, the advantage of using the App Start syntax in Dart is that it does not have a method body.
38. How do you define App Start Methods in Dart?
We use the return keyword, method name, and semi-colon to define App Start Methods in Dart.
39. How do you use the App Start method?
To use the App Start method, we follow the concept of inheritance.
We extend the shape to include a draw method if we have a rectangle.
However, we must override the method within the rectangle when extending the App Start.
40. Can we define other normal functions within the App Start in Dart?
Yes, we can define other normal functions within the App Start in Dart, such as void my everyday function.
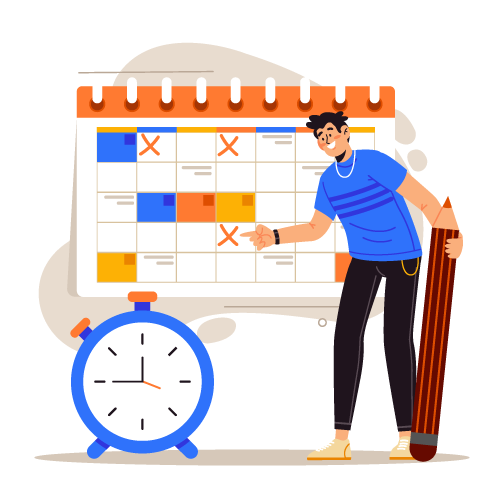
Dart Online Training
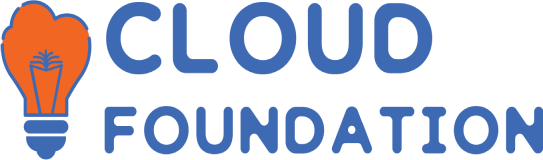
41. Is it necessary to override normal functions within the rectangle in Dart?
No, overriding normal functions within the rectangle in Dart is unnecessary.
42. Why is Dart’s syntax of using the brick keyword, labels for loops, and nested for loops highly discouraged?
Due to the code convention, Dart’s syntax of using the brick keyword, labels for loops, and nested for loops is highly discouraged.
43. How do you break out of a loop after a specific condition in Dart?
Using the break keyword, you can break out of a loop after a specific condition in Dart.
44. What is the purpose of using label loops in Dart?
Labels in loops in Dart are used to break out of a specific loop when you have nested loops.
45. Explain a name loop in Dart.
Yes, you can name loops in Dart as they are user-defined and can be named as desired.
46. What is a callable in Dart?
A callable in the Dart can be called like a function.
47. How do you convert a class into a callable in Dart?
To convert a class into a callable in Dart, you must implement the call method within the class.
This Dart platform might help you develop better multiple-choice questions and answers!
1. What is the purpose of using the “App Start” keyword in Dart?
Define a shape as an App. Start ✔️
Create objects from the App, Start
Instantiate or create new expressions
None of the above
2. What is the advantage of using App Start in Dart?
It has a method.
It cannot be instantiated or created with a new expression ✔️
Allows objects to be made from this
None of the above
3. How do you define App Start Methods in Dart?
Using the return keyword, method name, and semi-colon ✔️
Using the extend keyword
Using the new keyword
None of the above
4. What is the importance of overriding App Start methods within App Start?
Avoid creating objects from App Start.
Ensure the App Start method has a method body
To allow the method to be used within the App Start ✔️
None of the above
5. How do you write a single-line comment in Dart?
Using a pair of forward slashes ✔️
Backward slashes
Pair of asterisks
None of the above
6. How do you write a multi-line comment in Dart?
Using the forward slash and asterisk, followed by asterisk and then the forward slash ✔️
Using the forward slash and backward slash, followed by backward slash and then the forward slash
Using the forward slash twice
None of the above
7. What happens when the fourth loop tries to iterate but fails due to a false condition?
terminates, and only three hellos are displayed in the output console ✔️
Continues until a proper condition is met
Skips the iteration and moves to the next loop
Crashes the program
8. What is the primary function of an application?
The entry point of the application ✔️
The last function defined in the application
Function with the highest number of parameters
The highest value
9. What is the purpose of determining the return type as void in a function?
To indicate that the function returns no value ✔️
Show that the function returns a value
Demonstrate the role’s complexity
Indicate the function’s execution time
10. What is the default behaviour for a dot function without a return statement?
It returns null ✔️
Throws an error
Takings the last statement executed in the function
Proceed to the area of a rectangle
11. What does the course cover in Dart programming?
Syntax and properties of functions ✔️
Properties of loops
Properties of comments
Syntax and properties of variables
12. What are the standard exceptions in Dart?
Format exceptions and integer division by zero exceptions ✔️
Null reference exceptions and type mismatch exceptions
Array index out-of-bounds exceptions and unhandled exceptions
None of the above
13. What are the two objectives of using on and catch clauses in Dart?
To determine the exception type and handle type✔️
Create custom exceptions and understand the events before throwing the exception.
Test the code by dividing it by a valid integer and consistently execute the final clause.
Manage the integer division by zero exception and add a last clause to the code.
Dart is an ideal programming language for building mobile and online applications, particularly with its clean syntax, fast speed, and extensive community support.
As such, it has quickly become one of the more sought-after developer options with Dart programming interview questions.
Understanding and becoming proficient with Dart can dramatically boost career opportunities and professional advancement.
We hope this blog has provided valuable lessons about its core principles and anticipated interview questions you might face in a Dart interview through theDart language interview questions.
So, step forth with confidence and attack your Dart interview!
Best of Luck!!! Thank You!!!
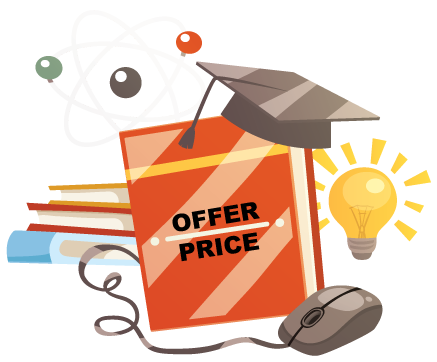
Dart Course Price
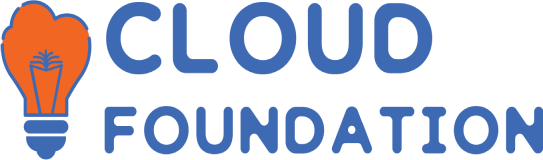
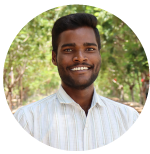
Shekar
Author