Array Interview Questions
Our Array in Java interview questions blog will cover array data structures, algorithms and applications, and best practices using arrays in popular programming languages.
Array Interview Questions in Java provides job hunters and hiring managers with access to knowledge regarding a variety of interview questions and interviews.
One of the primary goals of the Java Interview Questions on Arrays blog is to offer insightful and helpful answers to frequently asked interview questions regarding arrays.
Overall, Array Coding Questions are an indispensable resource for anyone preparing to participate in an array-related interview.
By offering helpful answers to technical inquiries while emphasising professionalism and honesty as core values for interview preparation, this Array Programming Questions blog can aid job hunters and hiring managers in successfully passing an array of examinations.
1. What is an Array?
An Array is a data structure that allows you to store multiple elements of the same data type, such as a number or a data item, in consecutive memory locations.
2. What are Arrays in Java?
Arrays are Java data structures containing multiple values and homogeneous elements. They maintain type safety by storing only integers in the same variety.
3. What are the types of Arrays in Java?
There are various Arrays in Java, such as single-dimensional or one-dimensional Arrays, two-dimensional Arrays, and multi-dimensional Arrays.
4. What is the purpose of one-dimensional Arrays in Java?
One-dimensional Arrays are used for data storage in software design, such as storing a country’s population in states.
5. What is indexing in Arrays in Java?
In Java Arrays, elements are indexed based on size, with four indexes (0, length of the Array – 1) for a size of 5. Every component of an Array is accessible through this index.
6. What sorting and searching techniques are used in Arrays in Java?
Sorting and searching techniques in Arrays are essential for solving fundamental problems and storing large amounts of data in a single container.
7. How is an Array updated in Java?
To update an Array in Java, you must use the index and assign a new value.
8. How is a country’s population stored in a Java Array?
To store a country’s population in a Java Array, you can create a multi-value container by writing an integer Array named state population.
This will have five elements and be stored in a single-value container.
9. What is the purpose of using Arrays in Java?
Arrays are used in Java to efficiently store and manipulate multiple values of the same data type.
They provide a contiguous memory location for data storage, making them useful for various applications.
10. What is the difference between one-dimensional and two-dimensional Arrays in Java?
One-dimensional Arrays in Java store a single value at each index, while two-dimensional Arrays in Java store a collection of values at each index.
11. What is a stack data structure?
A stack data structure is a linear data structure that follows the Last-In-First-Out (LIFO) principle, meaning the last element added to the stack is the first to be removed.
12. What is a heap data structure?
A heap data structure is a specialised tree-based data structure that satisfies the heap property, meaning that any parent node’s value is greater than or equal to the values of its children.
Heaps are commonly used for sorting and searching algorithms.
13. How is a one-dimensional Array created in Java?
Creating a one-dimensional Array in Java is simple. You need to give a reference variable name, specify the data type, and use a new operator with the size.
14. How is the country population calculated in the Array?
The country population of an Array is calculated by adding the country population of the first element to the country population of the first element.
This ensures that all components are, by default, zero.
15. What is the difference between an Array with default initial values and an Array with default initial values?
The main difference between an Array with default initial values and an Array with default initial values is that the former can be accessed and updated individually.
At the same time, the latter can come in before performing certain operations, allowing for more flexibility in writing and rereading the data.
16. What is the use of implicit and explicit ways to update data in Java?
The assignment operator implicitly updates the data, while the write operation is explicitly converted into an update operation.
17. How do indexes allow for read, write, and update operations on one D Array?
Indexes allow for read, write, and update operations on one D Array by dividing the data into 0th, 0th, and first elements.
Accessing any element in a D Array is done by mentioning its indexes, and updating the data is done based on indexing.
18. What is an enhanced loop used for in Java?
An enhanced loop reads an Array, starting from the zero index and copying the element at the zero index into a variable called ELM.
19. What are the time-saving benefits of using indexes in data structures?
Using indexes in data structures provides time-saving benefits by reducing the development effort required to access and update data.
20. What are two-dimensional Arrays, also known as Arrays of Arrays?
Two-dimensional or Arrays of Arrays represent a matrix by dividing the data into 0th, 0th, and first elements.
21. How does accessing any element in a 2D Array work in Java?
Accessing any element in a 2D Array in Java is done by mentioning its indexes.
22. What is the world population represented as in the provided program?
The world population is represented as an Array of Arrays, where the first Arraymeans the first country, and the second Array represents the second country.
23. How is the population count for each state represented in the Array of Arrays?
The population counts are represented as integers in the Array of Arrays, with the 0th index representing a job and the first and second index representing the countries.
24. How is the data represented in an Array of Arrays at a hash code in the program?
The data is represented as an Array of Arrays, where the first index is the zero index, followed by the first and second index.
The data is indexed for each element, starting with 0 and then moving on to the followingaspects.
25. What is a country’s population in the Array?
A country population refers to a country’s population, which is stored as an Array with a default initial value of 0.
The population can be updated after performing certain operations.
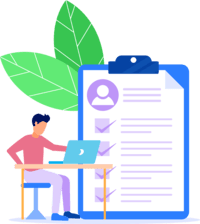
Array Training
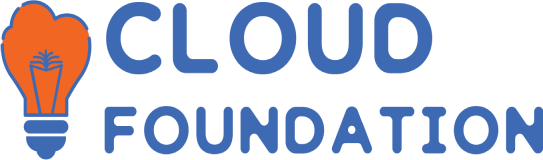
An example of a new class that uses 2D Arrays in Java is a class that represents the world population.
27. How does the program calculate the world population?
The world population is calculated by adding the world population to the size and the world population dot length.
28. What is the concept of writing and updating operations in programming?
Write and update operations in programming refer to the ability to modify and manipulate data within a program.
This can include creating, editing, or deleting data structures such as Arrays.
29. What is an example of a write operation in programming?
An example of a write operation in programming could be updating the value of an Array element, such as changing the value of a world population from 1 and 2 to 3 and 4.
30. What is an example of a read operation in programming?
An example of a read operation in programming could be retrieving the value of an Array element, such as reading the value of a world population Array and returning all sevens.
31. What is the concept of two-dimensional Arrays?
Two-dimensional Arrays, or Arrays of Arrays, are data structures that store multiple Arrays within a single Array.
They help keep and manipulate data with a two-dimensional structure like a grid or table.
32. What is the thumb loop?
The thumb loop is a programming technique that uses a loop to iterate through data using the thumb as an index.
It is a simple and efficient way to iterate through Arrays and other data structures.
33. What is the use of nested for loops in 2D Arrays?
Nested loops iterate through the elements of a 2D Array. They can perform operations on each component, such as adding or updating values.
34. What is the addition problem using two 2D Arrays?
The addition problem using two 2D Arrays involves adding the values of two matrices, A and B, to create a new matrix, C.
The matrices are represented as Arrays of Arrays, and the loop runs until C’s length is reached, adding elements to A and B.
35. What is the concept of Arrays?
Arrays are a collection of elements of the same data type stored in a contiguous memory block.
They are used to solve various problems and are a fundamental data structure in computer science.
36. What are the different topics on Arrays?
An Array, differentiating between Python lists and Arrays, creating a race in Python, accessing Array elements, and performing basic operations on Arrays like finding the length, adding and removing elements, Array concatenation, slicing, and looping through an Array.
37. What is the main difference between Python lists and Arrays?
The main difference between Python lists and Arrays is their storage methods.
Arrays store single data type values, while lists can store any values.
For example, an Array of integer values will only contain integers, while a list can store integers, floats, cares, and strings.
38. How do slicing and looping operations work for lists and Arrays?
Operations like slicing and looping are similar for lists and Arrays, but Arrays allow easier operations like multiplying or dividing values.
39. How does using 2D Arrays simplify data structures and improve the efficiency of software solutions in Java?
The use of 2D Arrays simplifies data structures. It improves the efficiency of software solutions in Java by dividing the data into 0th, 0th, and first elements, allowing for easy data access and update.
40. How can Arrays be created in Python?
In Python, to create Arrays, you must import the Array module.
This module has all the necessary functions for creating and performing various operations on Arrays. You can import this module by its original name or using an alias name.
41. How important is understanding the difference between lists and Arrays?
The session concludes by discussing the importance of understanding the difference between these two types of data structures.
It is crucial to know when to use lists and when to use Arrays to solve specific problems efficiently.
42. What is the use of enhanced loops in 2D Arrays?
Enhanced loops, also known as for-each loop, are a more concise way to iterate through the elements of a 2D Array.
They can perform operations on each Array element, such as adding or updating values.
43. What is the most commonly used method to import an Array module?
The first method to import an Array module is the most commonly used, executed without errors.
44. How can Arrays be created?
To create Arrays, use the second method, which imports the Array module as ARR, an alias name for the module.
The data type can be or any other choice.
45. What is the third method to import Array elements?
The third method, using the star from Array import star, imports all elements in the Array module.
This method specifies the constructor name, type code, and value list.
46. How are Array elements accessed?
Array elements can be accessed using index values, which hold unique aspects.
Indexing starts from zero and moves towards the right, with negative indexing beginning from the right and moving towards the left.
47. How can Array elements be accessed using negative index values?
To access Array elements using negative index values, create a heading in the JupyterNotebook and prefix text with the number of hashes based on the desired heading level.
Negative indexing returns the element at the position specified by the number of elements from the end of the Array.
48. How important is understanding the traversal order and indexing in data structures?
Negative indexing starts from the right and moves towards the left, and understanding this order is essential for accessing elements in data structures.
It also helps in efficient data retrieval and manipulation.
49. What is the purpose of using Arrays in programming?
Arrays store and organise multiple values of the same data type in a single variable.
They allow for easy addition or removal of elements and can be used to perform various operations on the data.
50. What is the length function used for in Arrays?
The length function is used to find the length of an Array in programming. It returns an integer value based on the Array’s name, indicating the number of elements in the Array.
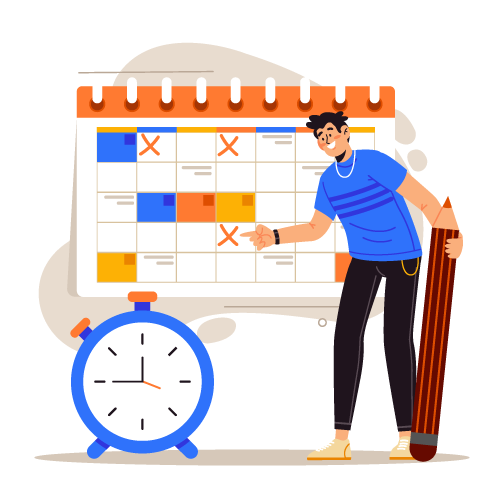
Array Online Training
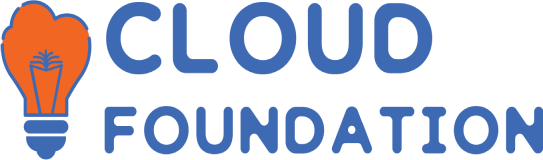
The three functions used to manipulate Arrays are append, extend, and insert.
The append function adds an element to the end of the variety, the extend function adds multiple elements to the end, and the insert function adds a new element to a specific position in the original Array.
52. Can an Array be created using a float value?
No, an Array cannot be created using a float value in programming. This would result in a type error.
53. What is the difference between Arrays extend and insert functions?
The extend function adds multiple elements to the end of an Array, while the insert function adds a new element to a specific position in the original Array.
The extend function specifies values within square brackets, while the insert function specifies an index number for the new element.
54. How do you add elements to an Array using the pop or remove functions?
You can add elements to an Array using the push function.
This function adds an element to the end of the variety and does not return it.
You can also add elements to an Array using the splice function.
This function adds or removes elements from an Array at a specified index.
55. What is the difference between the pop and remove functions?
The pop function returns an element from an Array, while the remove function removes an element from an Array but does not return it.
The pop function can take no or one parameter, while the remove function takes one parameter.
56. How can I use the pop function to remove the last element from an Array without specifying any parameter?
You can use the pop function to remove the last element from an Array without specifying any parameter by calling the function on the Array without arguments.
This will remove the last element from the Array and return it.
57. How do you re-print an Array using the pop function without specifying any parameter?
You can re-print an Array using the pop function without specifying any parameter by first calling the pop function on the Array to remove the last element.
This will remove the last element from the Array and return it.
You can then print the updated Array to see the changes made by the pop function.
58. What is Array concatenation using as the data type?
Array concatenation uses as the data type combines two or more arrays into a single array.
This demonstrates the concept of Array concatenation using as the data type and how to concatenate Arrays of different data types.
59. How does slicing an Array work?
Slicing an Array involves fetching specific values from the Array using the colon symbol.
Shows how to cuta variety from zero to three, starting from zero and continuing until three, but not including the value at three.
They then move on to reprinting the Array d and slicing it from zero to five, starting from zero and continuing until five, but not including the value at five.
60. What are negative index values in programming?
Negative index values in programming reveal that zero colon minus two outputs all values between zero and minus two but does not include the current value.
61. What is colon minus one in programming?
The concept of colon minus one in programming prints a reversed copy of the original Array. However, it is not preferred due to memory exhaustion.
62. How do for and while loops work in programming?
The for-loop rates over an Arraya specified number of times, while the while-loop rates until a condition is met or accurate. Illustrates these loops using a random Array and a Jupyter Notebook.
63. How do you slice an Array and print specific elements using a for loop?
To slice an Array and print specific elements using a for loop, you can initialise an iterator to zero, specify a condition, and increment it.
Then, you can use the length function to loop through the Array and print each element one after the other.
64. What is the concept of Arrays in C programming?
Arrays in C programming are necessary for storing and processing numbers.
To declare a variable, you must take a variable of type, an integer assigned a low value in the memory.
Memory is one by two by two by 1 bytes, and the integer size depends on the machine’s independence.
The size of the integer can take 2 bytes or 4 bytes, depending on the compiler.
For simplicity, the variable’s address is in hexadecimal form, and the 4 bytes allocated to it are used to store the number.
65. What is the concept of memory locations and initialisation?
Memory locations and initialisation refers to storing and retrieving data in a computer’s memory.
It involves allocating a specific area in the memory for each variable and initialising it with the desired value.
66. How many numbers can be stored in a single variable name?
For simplicity, it is suggested that only one number be stored in a single variable name.
However, for processing large numbers of data, such as 60 students, a single variable name can keep 16 numbers using an address.
67. How does Array work?
An Array is a set of items that may be accessed and handled as a single unit.
An index or key uniquely recognises each value. Arrays are a data type used in computer programming to store and retrieve many elements of the same kind, such as strings or numbers.
They are often used to traverse over and process the data contained in the Array in loops and conditional expressions.
68. How is the size of an Array determined?
The size of an Array is determined by the number of elements you want to store in it.
You can specify the size of an Array when you declare it, and the compiler will allocate the necessary space in the memory.
69. How is an Array located in memory?
An Array is located in consecutive memory locations, starting from the address allocated by the compiler for the first element in the Array.
The memory manager gives the array the necessary space and returns the starting address.
70. What is an Array in computer systems?
An Array is a collection of data items of the same type, such as integers or floats, stored in continuous memory locations.
It is essential for keeping data in a computer system.
71. What is the definition of an Array?
The definition of an Array is a collection of more than one data time, and all data items must be of the same type.
For example, a simple Array with a size of 5, 8, or 1-day Array can be declared using the following syntax: data type, name, size, and sample.
72. What are the constraints and conditions for an Array?
The constraint for an Array is that all data items must be the same type, and the condition is that the data items should be the same.
This allows for a homogeneous data collection, where all data items are similar.
73. What common errors can occur when declaring an Array?
Some common errors when declaring an Array include using negative numbers or constants, not specifying the size, or displaying various data items of different types.
74. What is the importance of using macros to define Arrays and their sizes?
Using macros to define Arrays and their sizes emphasises the need to practise and ensure that the declarations are correct and consistent.
Macros can be used to declare hash values and size in a primary function, making managing Arrays on a laptop more accessible and efficient.
75. What should be the size of an Array?
The size of an Array should be a positive expression, as it is used to store operands.
Correct any incorrect expressions and ensure that other variables are adequately declared before an Array is declared.
The JavaScript Array Interview Questions blog is invaluable for job hunters preparing for interviews.
It offers valuable topic-related questions designed to hone candidate skills and showcase knowledge across various areas.
They provide ample opportunity for candidates to demonstrate their qualifications for any role or sector.
Following the guidelines, Java Array Questions and Answers PDF candidates can ensure they arrive fully armed for every scenario during an interview process.
Learning Array Programming Interview Questions in Java for experienced and Fresher developers can also assist developers with honing their problem-solving abilities, mainly as many involve finding an efficient method to address specific problems requiring knowledge of algorithms and data structures.
Overall, learning an array of interview questions is an investment worth making for any developer looking to develop their skills and advance their career.
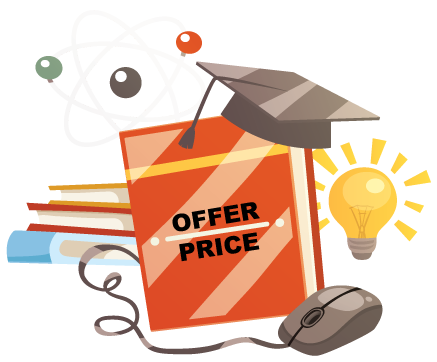
Array Course Price
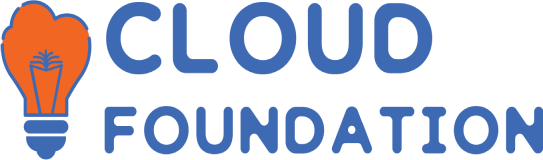
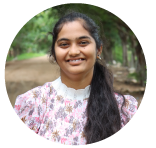
Sindhuja
Author