Android Interview Questions
Android, an open-source operating system developed by Google for mobile devices, relies on an altered Linux kernel with primary programming in Java as a primary language allowing developers to capitalize on both Java ecosystem and robust Linux kernel functionality for development purposes.
It includes libraries, tools and services which simplify application creation while offering consistent user experiences across devices.
1. What is Kotlin, and what platforms does it target?
Kotlin is a statically typed object-oriented programming language developed by JetBrains, it targets three platforms: Java virtual machine (JVM), Android, and JavaScript.
2. What are some advantages of learning Kotlin?
Kotlin offers a modern, pragmatic approach to programming with a focus on practical use cases, it combines object-oriented and functional programming features, making it a versatile choice for developers. Kotlin was officially adopted by Google as the language for Android development in 2017.
3. What are some features of Kotlin?
Kotlin is concise, safe, and easy to learn, with features such as operator overloading, lambda expressions, and string templates, it is also fully interoperable with Java code.
4. Which organizations use Kotlin for their projects?
Kotlin is used by various organizations, including Pinterest, Coursera, Pivotal and Gradle.
5. How do you install Kotlin development environment?
You can use the Kotlin playground for simple testing or install IntelliJ IDEA for a more comprehensive development environment. Before setting up Kotlin, make sure you have Java installed on your system.
6. What is the role of “fun” in Kotlin?
In Kotlin, “fun” is a keyword used to create functions, similar to Java’s “final” keyword. Functions are named “fun” and parameters are passed in braces.
7. What are constructors in Kotlin and what are their types?
In Kotlin, constructors are used to generate an object during instantiation. There are two types of constructors: main and secondary constructor. The primary constructor is part of the class header and defines properties, whereas secondary constructors are called when the object is formed.
8. How are properties initialized in Kotlin?
Properties in Kotlin are initialized using initializer blocks, which are executed as soon as the object is created. Initializer blocks can be used to initialize properties and print values.
9. How do Kotlin’s getters and setters vary from one another?
Getters and setters in Kotlin are used to get and set the value of a property, respectively. They are optional and auto-generated if not created in the program.
10. What is inheritance in Kotlin and how does it work?
Inheritance is a feature of object-oriented programming in Kotlin that allows users to create new classes from existing ones. The derived class inherits all features from the base class and can have additional features. The syntax of inheritance involves the keyword ‘open’ before the base class.
11. What are visibility modifiers in Kotlin and how are they used?
Visibility modifiers in Kotlin are keywords that set the visibility of classes, objects, interfaces, constructors, functions, properties, and setters. They are used to control access to these elements from outside the class or package.
12. In Kotlin, what distinguishes the terms ‘if-else’ and ‘when’?
‘If-else’ and ‘when’ are both used to perform conditional logic in Kotlin, but they differ in syntax and use cases. ‘If-else’ is used when there are multiple conditions that need to be checked, while ‘when’ is used when there is a single condition with multiple possible outcomes.
13. What are loops in Kotlin and how do they work?
Loops in Kotlin are used to execute a set of operations multiple times. The most common type of loop in Kotlin is the ‘while’ loop, which executes code as long as a certain condition is true.
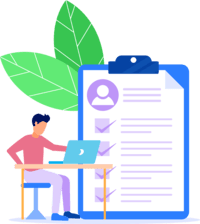
Android Training
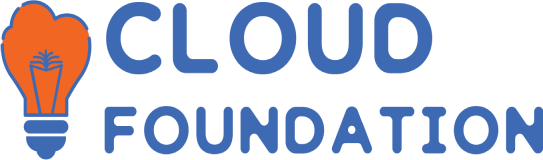
The primary distinction between ‘while’ and ‘do while’ loops in Kotlin is the order in which the code is performed. A ‘while’ loop checks the condition before executing the code, but a ‘do while’ loop executes the code first, then checks the condition.
15. What is the syntax of a while loop in Kotlin and how is it used?
The syntax of a while loop in Kotlin involves writing code that executes whatever is written inside the loop body, as long as a certain condition is true.
The condition is checked at the beginning of each iteration. An example of a while loop is to print the numbers 1 through 5.
16. What is the objective of Kotlin loops, and how can they help to simplify code?
Loops in Kotlin are used to repeat a series of processes numerous times, simplifying code and improving the overall developer experience. Using loops instead of manually repeating code allows developers to write more efficient and effective code in less time and effort.
17. What is the main distinction between Kotlin’s ‘for’ and ‘while’ loops?
In Kotlin, the syntax and use case differ most significantly between a ‘for’ loop and a ‘while’ loop. A ‘for’ loop is used to repeat the process over a sequence of data, whereas a ‘while’ loop is used to conduct a series of actions depending on a predefined condition.
18.What does the XML file do in Android app development?
XML files contain the name, value key, and value, making them essential for Android app development. The name must be used carefully to prevent putting values everywhere. XML files include layout, design components, and pictures, according to the content.
19.What is the main activity and content of an Android app?
The primary activity of an Android app is a Kotlin-compatible Java class. Opening and closing base braces start the class, which overrides a parent class method. The function is called on create and includes activities major design features. On create is the first method called when the app opens. The app provides stop, resume, and destruct functions. Any software engineering rule requires understanding Android app life cycles.
20. What is the basic structure of JSON data?
JSON data is represented by curly braces and arrays. Objects are represented by curly braces, while arrays are represented by square brackets.
21. What is a view holder and why do Android apps need recycler view adapters?
A recycler view adapter is essential for a beautiful, functioning app. This UI UX framework component has a recycler view dot view holder. The view holder holds data if the layout manager fails to generate a view, such as while filling data. Image, music, title, play, and pause views are initialized.
22. Why is consistency in JavaScript view creation and management important?
The author stresses the need of a consistent strategy to building and managing JavaScript views to ensure well-structured and understandable code. Give views meaningful names, configure height and width, and show text using text views.
23. What are popular Android Studio view choices for app design?
Text view, numerous buttons, radio buttons, check boxes, floating action buttons, and floating action are mentioned. It covers linearly out constraint, frame layout, table layout, and more.
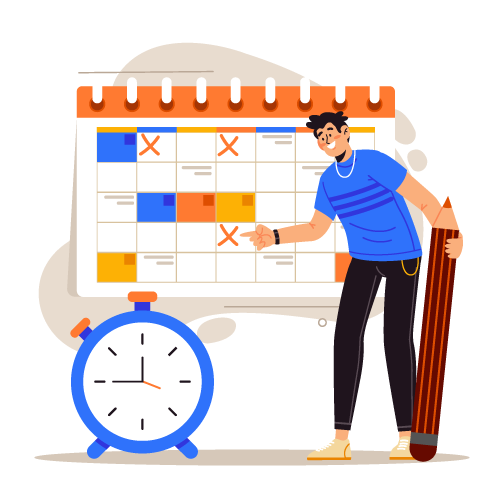
Android Online Training
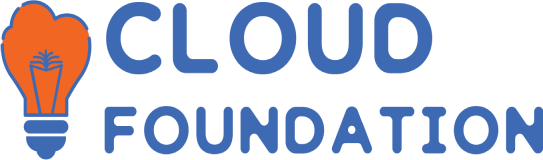
24. Why are intents important in Android app development?
Android app development relies on intents to enable users switch between activities. Effective and user-friendly experiences need intended understanding.
“Test Your Android Knowledge: Unleash Your Potential with MCQs on Android!”
1. Which operating system is used for developing applications for mobile devices, mainly for the Samsung and other brands’ smartphones and tablets?
a. iOS
b. Windows Phone OS
c. Android
d. Microsoft
2. Android is an:
a. Application
b. Operating System
c. Programming Language
d. Type of smart phone
3. Which company developed the Android operating system?
a. Microsoft
b. Apple
c. Google
d. Linux
4. Which version of Android operating system was released first?
a. Ice Cream Sandwich
b. KitKat
c. Gingerbread
d. Cupcake
5. Which feature of Android operating system allows users to download and install applications from outside the Google Play Store?
a. Bloatware
b. Widgets
c. Split-screen multitasking
d. Side loading
6. Which operating system is used for developing applications for mobile devices with the “Android” platform?
a. iOS
b. Windows
c. Linux
d. Apple
7. What is Android a short form of?
a. Advanced Robotic Operating System
b. Automated Regional Operating System
c. Amalgamated Computing System
d. Open-source mobile operating system
8. In which year was the first Android device launched?
a. 2001
b. 2005
c. 2007
d. 2008
9. Which version of Android is the most recent as of now?
a. KitKat
b. Marshmallow
c. Nougat
d. Pie
10. What is the primary interface used to develop Android applications called?
a. Java Interface
b. Swift Interface
c. Kotlin Interface
d. React Native Interface
11. Which of the following features is not available in the Android operating system?
a. Multitasking
b. Split screen
c. Virtual keyboard
d. Haptic feedback
12. Which app doesn’t comes pre-installed in every Android device?
a. Microsoft Office Suite
b. Google Maps
c. Calculator
d. Camera
13. Which of the following is an advantage of using the Android operating system?
a. It’s not customizable
b. It’s exclusive to certain device brands
c. It’s user-friendly and customizable
d. It’s expensive to use
14. Which Android app allows users to send voice-activated messages and make voice searches?
a. Google Maps
b. Google Assistant
c. Google Play Music
d. Google Drive
15. Which of the following is not a part of the Android Open Source Project (AOSP)?
a. Hardware drivers for specific devices
b. Android runtime
c. Linux kernel
d.User interface and core applications
Conclusion:
Kotlin Android Interview Questions require a sound understanding of both Kotlin syntax, as well as Android application development. Android is an open-source mobile operating system specifically tailored for smartphones and tablets.
Google developed Android as the operating system to power most mobile devices around the globe, making it one of the world’s most ubiquitous OS.
Android’s open-source nature makes customization and flexibility possible, while a large community of developers create and release new applications and updates on a continual basis.
Android offers consumers and businesses alike an intuitive user interface and robust features – all making it one of their preferred choices in an ever-evolving mobile technology market.
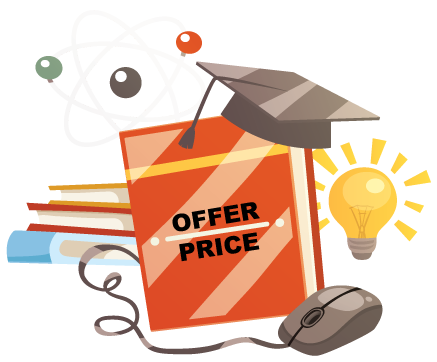
Android Course Price
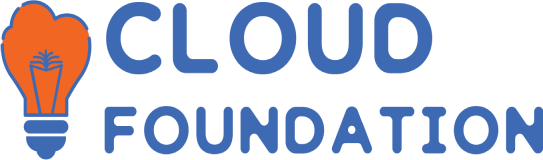
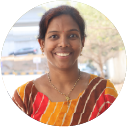
Deepthi
Author