OpenCV Tutorial
Introduction to OpenCV
OpenCV is a Python library that manipulates images, videos, and media files.
This is a popular choice for creating high-quality media files. Like humans, OpenCV also acts as artificial eyes to see and process visual data.
OpenCV can also be found in various applications, including image recognition in self-driving cars and face recognition technology such as that found on Facebook.
Such software enables computers to detect lanes more accurately while creating more beneficial information for users.
OpenCV is an accessible cross-platform library that is available under the BSD license. It is designed for ease of use with its simple installer interface.
Navigating the OpenCV Installation Process
To use OpenCV for Python, follow these steps:
Open your favourite browser on your Windows 10 operating system.
Search for “operating system” and then “Python“.
Click on the first link from python.org.
Open the Python Waddy website.
Scroll down until you see the downloads section.
Click on the link that says “Python” and then click on the link that says “Python 3.7.0.”
Contours in OpenCV
Contours are integral for shape analysis, object detection and recognition with OpenCV. The Find Contours method, Cone-to Approximation method, and Red Chain Approx Symbol can effectively locate contours within images to approximate them.
Contours in OpenCV can be defined as boundaries that connect continuous points along an object’s perimeter. They are an invaluable way to analyse, detect, and recognize shapes and objects.
To identify contours within an image file, first convert it to grayscale using the CVRM show method and then use the Canny edge detector to capture and recognise edges. Save and run the image to view the results of edge detection.
The Find Contours method returns two key elements—contours and higher keys. The Cone-to-Approximation method uses a cone-to-CV dot chain to unscrew approx and go numb, creating two keys as its output.
This function analyses any edge structures in an image and returns their values and contours—it essentially produces a Python list containing coordinates for all contours within.
Image Transformation and Rotation Overview
I created a translated image using Python’s “translate” function and shifted it left/right by 100 pixels. I saved the file and then ran “Krones formations dot py,” which shifts down/right 100 pixels of its translated image.
Once this function has been created, “rotate” takes an image angle and rotation point as inputs, setting its height and width according to its shape.
Without an explicitly stated rotation point or center rotation matrix being specified for an image, its rotation matrix will rotate around this point with no reference to scale whatsoever.
An image can be rotated by 45 degrees to produce a “rotated” version that appears “rotated” when displayed and saved into rock.
By default, rotation occurs counterclockwise; alternatively, negative values for the angle can be specified to reverse its effects and turn clockwise instead.
To further adjust an image, its rotation can be changed by 45 degrees more. To prevent skewness in the resultant picture, angles may need to be combined and altered into one final angle of 90 degrees to recreate the original picture.
Adjusting “translate” and “rotate” allows images to be transformed and represented visually more accurately.
Essential OpenCV Image Functions
OpenCV makes creating images easier with its suite of functions for image manipulation, with resize, cropping, and interpolation among its primary focuses.
Resize is used to enlarge an image, while cropping selects portions based on pixel values; both processes produce images with higher-than-original quality results.
This OpenCV workshop will introduce basic image transformation techniques such as translation, rotation, resizing, cropping and clipping.
Translation involves shifting an image along its x and y axes up, down, left or right as desired; rotating can change its position on all four planes (x/y/z/w). Clipping/cropping applies similar principles of transformation, but to different image elements – rotating an image vertically by 90 degrees can produce similar effects with cropped photos as cropping photos vertically by the same effect; clipping/cropping involves changing its dimensions using various algorithms used during cropping/cropping processes.
To translate an image, we can create the def translate function. It takes an image as input and an array x/y representing how many pixels should shift along each axis.
Rectangle function method in OpenCV
The rectangle function is an array-based method that takes several arguments: image file names, locations 1 and 2, and various adjustable parameters such as colour and thickness settings.
OpenCV provides the rectangle function for drawing rectangles; its arguments include an image filename and vertex coordinates for the top-left and bottom-right corners (x1,y1) and (x2,y2). BGR colours (0-255) for red and thickness settings are also supported.
Adjusting the colour and thickness parameters enables you to tailor the rectangle’s appearance to make an accurate and visually distinct representation. The rectangle function considers multiple arguments, such as the image itself, points 1 and 2, and the colour and thickness settings.
The image itself, along with point 1 and point 2, coordinates for points 1 and 2, are given as arguments to this function. Next are their colours corresponding with lines drawn along this image; and finally, the third argument specifies thickness values as the fourth argument.
Using OpenCV for Face Detection
OpenCV detects the presence of faces in images by using classifiers, algorithms that use probabilistic techniques to judge whether an image contains positive or negative features and whether or not faces are present in that image. It comes equipped with pre-trained classifiers like Haar cascades for rapid classification purposes.
Haar cascade classifiers tend to be less vulnerable to noise in an image than other methods, making them suitable for upper body detection like Hawk cascade and whole body and smile detection in OpenCV.
OpenCV offers various Haar cascades, such as the Hawk cascade for upper body detection and the Eye cascade for all of this functionality.
To implement face detection, we first transform images to grayscale for easier analysis of objects within them. When performing face recognition, the focus is not on skin tone or colours but on what objects exist within an image and their position in itFace recognition is an integral component of image recognition, and OpenCV provides pre-trained classifiers tailored specifically for facial identification tasks.
Thresholding and Edge Detection in OpenCV
Simple and adaptive thresholding are two distinct methods of thresholding that may be employed, respectively.
Simple thresholding requires manual specification of threshold values, while adaptive thresholding utilises block sizes or neighbourhoods to calculate an adaptive threshold based on mean/Gaussian distribution models.
Gradients appear as edge-like regions in an image. A Canny edge detector is an advanced multi-step edge detection algorithm; other approaches for computing edges in images include the Laplacian and Sobel methods.
First, an image must be converted to grayscale using the CVT colour method before the Laplacian method can compute gradients within this grayscale image.
Laplacian (). Laplacian takes as input a source image and depth or data type; when set to CV_64F, it processes gradients within that image.
The Laplacian method computes gradients in grayscale images by converting all pixel values to absolute values and computing their gradients accordingly.
Implementing Adaptive Thresholding with OpenCV
Adaptive thresholding is an innovative technique for quickly finding an image’s optimal threshold value. It uses computer algorithms to do the work independently, which is ideal when manually setting threshold values is problematic or challenging.
To implement adaptive thresholding, set the adaptive Threshold equal to CV2–Adaptive Threshold in your Image Processing software and submit an image source whose maximum value exceeds 255; your adaptation method determines how it calculates an optimal threshold value.
Adaptive thresholding sets the kernel size or window. OpenCV computes an average over the pixels nearby in that region to find its optimal threshold value; the process repeats for each part of an image and can be fine-tuned using C values between 0 and 3, which allow finer adjustments of thresholding threshold values.
Alternately, Gaussian means can be employed in place of mean calculations; this adds weight to each pixel value for potentially improved image results, but no single method works perfectly across images.
Conclusion
OpenCV is an invaluable library that plays a pivotal role in image and video processing.
From basic functions such as resizing, translating, and rotating images to advanced techniques like face detection, contour identification, and adaptive thresholding, OpenCV offers a versatile set of functions suitable for beginners and professionals alike.
OpenCV offers users easy image manipulation and analysis methods, allowing them to tackle applications for object detection, image recognition, and real-time processing in fields like autonomous cars and security systems.
Mastering OpenCV’s core functionalities can significantly expand one’s capacity to develop intelligent applications using visual data, making it a valuable asset for computer vision and machine learning developers.
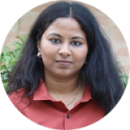
Navya Chandrika
Author