Java Concurrency – Multithreading Tutorial
What is Java
Java is a highly utilized computer platform and programming language used for developing applications for various devices.
Utilised widely to produce mobile applications, enterprise software solutions and operating systems.
Java programming facilitates the execution of various functions and classes.By extending the run method and creating separate classes, developers can implement their implementations more effectively while improving their code base.
Java Virtual Machine in Java concurrency – multithreading
The Java Virtual Machine, or JVM, manages multithreaded Java applications while handling concurrency features like multithreading. Multithreading enables programs to complete several tasks at the same time or concurrently in parallel multithreading can significantly increase responsiveness and performance of applications.
The JVM is at the core of Java’s handling of multithreading. It oversees thread lifespan, scheduling and synchronisation – when called upon an thread it finds that its target does not equal run and calls its run method instead.
This indicates that target is different from run, which causes JVM to discover when calling run method that target does not equal run and then call run method again.

What is Java multi-threading and concurrency?
Java Multithreading and Concurrency provides an introduction to multitasking history and development. Through concurrent execution of multiple tasks in parallel, it ensures more efficient use of time and resources.
Java’s thread class is an integral component of its system; when running programs with single trading environments, program flow stops or programs simply stop running altogether.
Because Java is a single-traded system, when its main thread stops executing or the program ends altogether. This concept is key to ensure efficient multitasking within Java.
Multitasking has greatly increased computer efficiency and effectiveness, providing more effective resource use while decreasing repetitive tasks.
Multitasking’s development has presented both challenges and opportunities, such as improved memory management needs or developing more energy-efficient hardware. Multi-threading is not exclusive to Java programming languages such as Python, C, or C++ – its benefits extend across programming disciplines like these and others such as C# or Swift.
Multi-threading in Java
Multi-threading works similarly to multitasking; however, instead of running just one application at the same time, multiple execution threads can run within it for simultaneous multitasking. This makes multi-threading more efficient and effective overall.
Computers can run multiple programs simultaneously; however, each thread of execution in an application must run concurrently on one CPU core in order to remain secure and efficient for communication purposes.
Dependent upon who controls them, threads may run on either the CPU, operating system or both simultaneously.
Once each thread completes its execution on one thread type it then switches over to the next thread for execution on its chosen platform before transitioning onto yet another one for further execution on that core before continuing down its next route.
This process continues until an operating system completely switches over to another application.
Multithreading refers to an approach in which multiple CPUs run separate applications at once, simultaneously, with different threads within each application executing at the same time – creating variable execution times across applications or threats.
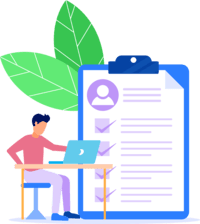
Java concurrency – multithreading Training
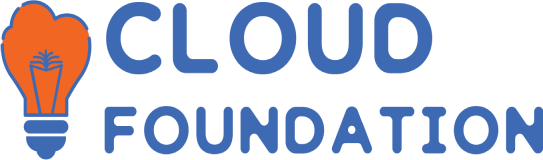
Multithreading is key for ensuring all threads in an application run simultaneously and reduce the risk of multiple threads running concurrently.
Multithreading can help achieve improved CPU utilization. By switching tasks quickly between tasks and performing needed operations on them, multithreading allows computers to make efficient use of available processor cycles without becoming idle and wasting precious cycles.
Multithreading is an integral component of computer programming, as it enables multiple threads to run concurrently across different CPUs.
Types of multitasking in Multi-threading
Multithreading provides two forms of multitasking; processed-based multitasking and thread-based multitasking.
Process-based multitasking entails running separate programs concurrently while thread-based multitasking involves running two programs at the same time.
Example of context switching (wherein one program switches with another). This occurs when someone types documents on MS Word while creating images with MS Paint; context switching allows users to easily switch back and forth between programs.
Thread Management and Performance in Multi-Threaded Java Applications
“The idle threadsare two separate processes running simultaneously that may use different threads to process information from disk or network drives and may contribute to poor system performance” if left running continuously, potentially increasing system load significantly and impacting overall system efficiency.
One thread might process data from disk or network drives while the other might process networked information; this can lead to performance issues in your system as a whole.
UI thread in Java
UI thread continues to transition back and forth between active and inactive states, giving the impression that application remains responsive to user input. But due to potential inefficiency issues, application may eventually use less CPU time than anticipated.
Multi-CPU computers often struggle with running background tasks for long duration.
Long-running tasks might run on their own CPUs, which might differ from that used for running the main UI thread. To execute it properly, initialisation must first take place prior to starting up long-running task(s).
Background tasks run on separate CPU threads from main user interface threads to ensure responsiveness to user interactions and updates to user interface elements.
The main UI thread within an application continues to execute and respond to user input despite not using all available CPU time.
As soon as the main thread leaves a program, its other threads continue to run without interruption; however, this doesn’t cause termination for it allows another user thread to run concurrently with it.
The two important concepts of Thread
There are two important concepts:
Thread and demand thread.
Threads: are threads that execute specific tasks,
Demand threads: execute tasks that are not completed by the user thread. The program will stop when no user threads are running, regardless of whether the demon or demon threads are still executing.
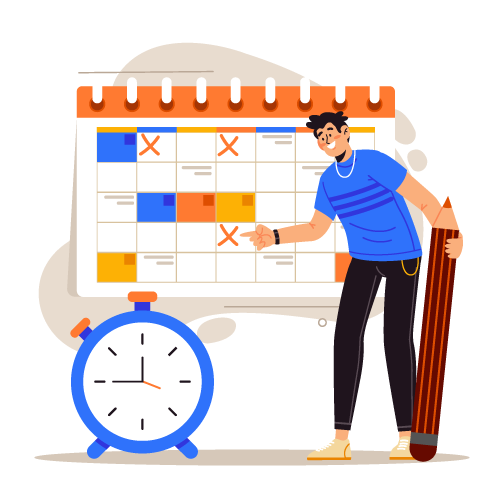
Java concurrency – multithreading Online Training
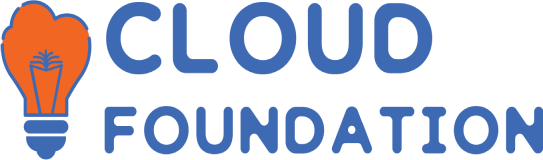
Thread Creation in Java
There are two ways to create threads:
The first method prints the thread’s, its parent’s, and the stud’s parent’s names by adding a space before printing the entire thread.
The second method involves creating a thread and another class that implements the runnable interface.
The runnable interface will give an error when overwriting the run function. The user must add an error message and overwrite the run function to fix this.
The roundabout interface is nothing but a function interface with one abstract method that is run. Any class that implements this enable interface must implement this interface and provide some abstract method.
The run method has to have some meaning, and any class implementing this enable interface must provide some abstract method. As a result, if it implements the run method, it must provide some implementation to the run method.
Process of creating a thread in a program
Initialisation of thread: the process starts by initialising a thread that can then be called up by users to execute specific tasks assigned by admins or managers. Anschliessend, thread is assigned tasks from its assigned users in the form of thread requests by them or admins as defined below:
Execution of code continues in parallel, assigning threads tasks they will complete upon executing said code. Once assigned a task for execution and completed successfully, its progress becomes known and shared across threads for execution by respective threads.
After this step is completed, execution begins by using the execute method on your code to run it and run any associated commands that use execute methods (for instance using execute and then run instead).
The code execution occurs and its respective execute method executes as well.
Ending in code execution, where both methods ‘execute’ and ‘execute’ are called upon for implementation, the process continues with code execution.
Conclusion
Java multithreading enables applications to carry out numerous tasks simultaneously, significantly increasing responsiveness and performance.
Java efficiently manages threads through its Virtual Machine (JVM), enabling applications to simultaneously execute network I/O operations or CPU operations without interruptions from previous processes.
Java developers who wish their applications ran efficiently must understand threads such as user and daemon threads and how best to manage and create them, in particular user and daemon threads, while managing resources efficiently by working with threads effectively.
By working effectively with threads they can ensure apps run quickly, responsively and utilize as many resources possible – whether developing business software, mobile apps or anything else; understanding threads makes programs better and more cost effective than before.
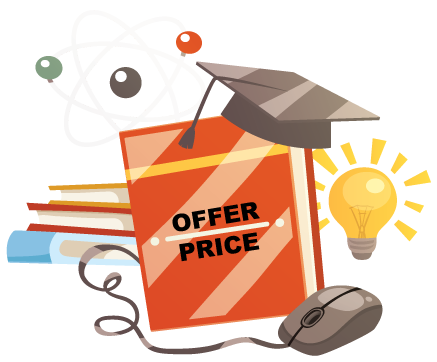
Java concurrency – multithreading Course Price
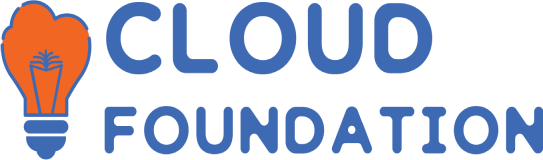
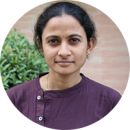
Vinitha Indhukuri
Author