Linked List Interview Questions | Interview Questions on Linked List
In the linked List Interview Questions blog, we’ll tackle common interview queries regarding linked lists.
Interview questions Linked List is an integral data structure in computer science and programming. They store collections of objects with values attached and references backwards.
We aim to cover various linked list question programming about linked lists from implementation through traversal and manipulation, critical principles like time/space complexity, and any errors/traps when using linked lists.
Providing you with a thoroughlylinked list question manual can aid in interviews and improving programming abilities.
Top linked list interview questions regarding linked lists may prove challenging; however, one can swiftly respond with proper preparation and understanding of the subject matter.
This blog linked list programming questions and answers will examine common linked list interview questions and answers about linked lists and provide comprehensive explanations to help you excel at subsequentrelated linked list exam questions interviews.
No matter your level of programming experience, novice or veteran alike.
This blog coding questions on linked list offers essential insights and recommendations that will increase proficiency and comprehension of linked lists.
1. What are linked lists?
Linked lists are structures in which items are stored in random memory and connected using arrows. They are not continuous memory allocations but are in different places, with each item secured using a reference variable. Each box in the linked list has its type and contains every box.
2. Among Java Arrays, what is the most significant restriction?
The main limitation of Java Arrays is that they are not continuous memory allocations. If an array gets full, it cannot add new items. Instead, ArrayList does something like this: it copies all the existing items and adds a new one. This is efficient but could be better.
3. Is there a way around the limitation of Java Arrays using Array List?
ArrayList copies all the existing items and adds a new one, which is efficient but could be better.
4. How can you explain fixed linked lists?
The concept of fixed linked lists is that it cannot directly access the index. The index only knows about the items in the list, not the total elements.
The nodes only know about the person the user loves and the integer value they know.
One can create a temporary node and run the for loop three times to access the third node.
5. May I ask what a custom linked list is?
A custom linked list is a linked list that uses a specific variable to point to each other. It can be implemented using a node class and a private class node with an int node next and a remote node next.
The constructor contains one constructor that takes simple values and another that takes a reference value.
6. When creating a new custom linked list, how can one set its initial size to zero?
To initialise a custom linked list with a size of 0, one can use the constructor that takes simple values and assigns a null value to the head and tail pointers.
7. How do you add a head and tail to a custom linked list?
To add a head and tail to a custom linked list, you can create a new node with the desired value, set the next pointer to null, and then set the head pointer to the new node.
8. Head and tail pointers are important in linked lists, but why?
The head and tail pointers in linked lists emphasise the importance of working with just the head, as the tail value makes inserting new items at the end of the linked list easier.
9. Can you explain how Python’s next pointer works?
The concept of a next pointer in Python is a reference variable that points to an object when adding or modifying it.
It demonstrates using this pointer to link objects and insert elements at specific points.
10. How can you put an element in a linked list at the beginning, at index one?
One must create a new node and update the head position to the second element to insert an element at the first index in a linked list. The head will then be equal to the node; if no single element is provided, the head will be created again.
11. In what ways is the size of a linked list computed?
The size of a linked list top interview questions can be calculated by starting from the head and counting until it reaches null.
12. When solving linked list problems, how long does it often take?
It just takes a few hours, and an A for loop or a simple loop is suggested to solve linked list questions and answers.
13. Why is it crucial to know how linked lists work with head and tail pointers?
Understanding the concept of head and tail pointers in linked lists and reference variables that point to nodes is crucial for working with linked lists in programming languages.
14. At what index can an element be inserted in a linked list?
To insert an element at a particular index in a linked list, one must traverse the entire list until the desired index is reached, create a new node with the desired value, and set the next pointer of the new node to the next node in the list.
15. Is there a way to cut a linked list’s tail?
To remove a tail from a linked list, one must traverse the entire list, remove the tail dot next to the second-last node, and set the next pointer of the second-last node to null.
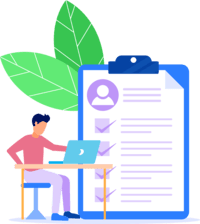
Linked List Training
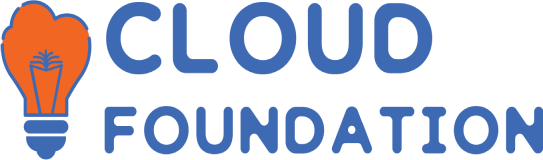
16. How can you remove a previous node from a linked list?
To remove a previous node from a linked list, one can traverse the entire list, find the node with the desired value, and set the current node’s next pointer to the next node in the list.
17. Which keys in a linked list can be accessed?
To find a value in a linked list, one can traverse the entire list, compare the value of the current node with the desired value,
18. To what does a linked list refer to as a node?
A node in a linked list is a linked list data structure questions type node with an integer value and a reference variable that points to an object in the heap. The next pointer is of node type, as it points to an object in the heap and does not jump onto it directly.
19. How does a linked list’s “head” work?
The concept of a head in a linked list is a temporary variable that is not changed during traversal. The head is always null when moving forward, and the temporary nodes are only used for traversal purposes. When changing a node, another reference variable points to the same node, indicating that any change made via this node will be represented in these nodes.
20. Is Making a new node in a linked list straightforward?
To create a new node in a linked list, you first create a further object of the node type and then set the node dot value to the new value and the node dot next to the next node in the linked list.
21. When creating a linked list, why must the first method be inserted?
The insert first method in a linked list adds a pointer to the head node, allowing for more efficient insertion of elements at the beginning of the list.
22. What is the purpose of the display part in a linked list?
The display part in a linked list prints the value of each node in the list, starting from the head node and moving forward until the end of the list is reached.
23. How do you remove an element from a linked list?
To remove an element from a linked list, you start with the head node and move forward until the component to be removed is found. You then remove the element and update the links between the nodes to maintain the list’s integrity.
24. For what does a linked list’s last node exist?
The last node in a linked list is the final node and is used for inserting new elements at the end of the list.
25. In a linked list, what does the variable that comes after the last dot do?
The last dot following variable in a linked list points to the next node, allowing for traversal of the list in both directions.
26. To what extent does it take time to traverse a linked list?
Time complexity refers to the time it takes to traverse a list of nodes in a linked list. It uses public insert at a particular index and implements a doubly linked list.
27. Before adding a node to a linked list, how can one verify it already exists?
To check if a node exists before inserting it in a linked list, you can traverse it and check if the desired node already exists before inserting a new node.
28. Can you tell me why a circular linked list is helpful?
A circular linked list is a simple implementation of a linked list that goes in a circle, with every node having an integer value and a next. No one is pointing to null until the list is empty.
29. How may one define a constructor for an element’s value in a circular linked list?
To create a constructor for a value in a circular linked list, you can initialise the nodes as null and use the constructor to check if the head and tail are equal.
30. To what end does Python’s public void function serve?
The public void function in Python prints the head node’s value in a linked list. It uses a loop to print the value of the head node multiple times until it doesn’t reach the current node.
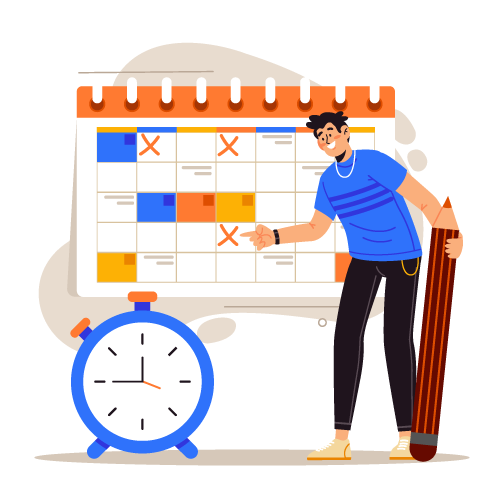
Linked List Online Training
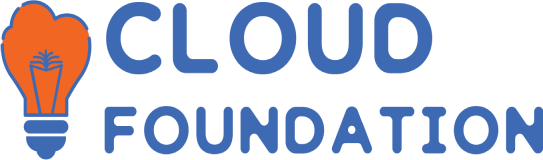
31. If you have a linked list, how can you remove a particular property?
To delete a specific value from a linked list, you can use a loop to traverse the list and check if the current node equals the desired value. If it does, you can print the value of the head and move forward to the next node.
“The Linked List MCQ Blog teaches students and professionals about linked lists. It offers an ethical list of MCQs, from simple concepts to advanced applications.
The Linked List MCQ Blog provides something for all programming levels. The site’s MCQs are polite and helpful for linked list beginners.
Positivity and fairness make them useful. The Linked List MCQ Blog contains several resources to teach this fundamental data structure.”
1. Which of the following is a limitation of Java Arrays?
a) A continuous memory allocation is not what they are.
b) Indexes attempting to access them will fail.
c) Stores only one type of data.
d) After creation, they cannot be changed.
Answer: a)A continuous memory allocation is not what they are.
2. What is the main advantage of ArrayList over Java Arrays?
a) Allocation of memory is ongoing.
b) It’s capable of storing many kinds of data.
c) Indexes do allow access to it.
d) It’s editable after the creation phase.
Answer: b) It’s capable of storing many kinds of data.
3. What is the purpose of breaking boxes into separate boxes in a linked list?
a) Clarify the linked list’s structure to benefit reader comprehension.
b) Facilitate the addition of new items to the connected list.
c) The linked list should be easier to navigate.
d) To facilitate the process of removing items from the linked list.
Answer: a) Clarify the linked list’s structure to benefit reader comprehension.
4. What is the next pointer in a linked list?
a) An identifier for the node that came before this one.
b) Array reference that references an item in the heap.
c) Reference to the root branch.
d) The final node’s pointer.
Answer: b) Array reference that references an item in the heap.
5. What is the importance of head and tail pointers in linked lists?
a) The index can be accessed directly.
b) Data stored in random memory is what it’s made for.
c) Linked lists do not require it.
d) Collaborating with a linked list’s head is emphasised as crucial.
Answer: d) Collaborating with a linked list’s head is emphasised as crucial.
6. How do you create a new linked list and initialise it with a size of 0?
a) Establish a dormant node.
b) After adding a node, set its value to head.
c) Make a uniquely sized linked list with no elements.
d) Create a private class node with int and remote nodes next. One constructor accepts simple values and one reference.
Answer: d) Create a private class node with int and remote nodes next. One constructor accepts simple values and one reference.
7. What is the time complexity of inserting elements in a linked list?
a) O (1)
b) O(n)
c) O (log n)
d) O(n-1)
Answer: b) O(n)
8. How do you insert a value at a particular index in a linked list?
a) Use the public integer delete function to remove an index.
b) Create a new node and update the head position to the second element.
c) Go to the previous node and declare a public void.
d) Remove the tail dot next to the second last node.
Answer: c) Go to the previous node and declare a public void.
9. What is the time complexity of removing a tail from a linked list?
a) O (1)
b) O(n)
c) O(log n)
d) O(n-1)
Answer: d) O(n-1)
10. How do you remove a previous node from a linked list?
a) Take off the tail dot adjacent to the most recent node.
b) Add a node and shift the head to the second element.
c) The public integer delete method deletes an index quickly.
d) Use the value’s last dot. Removed nodes should return their values.
Answer: d) Use the value’s last dot. Removed nodes should return their values.
11. What is the purpose of the head concept in a linked list?
a) Stand in for the list’s last node.
b) Put an object’s reference variable on the heap.
c) How the root node of the hierarchy.
d) Keep the node’s value.
Answer: c) How the root node of the hierarchy.
12. What is the main focus of the insert last method in the linked list?
a) Understanding the internal implementation and time complexity of linked lists.
b) Creating a new node and box and observing the final result.
c) Inserting a new node.
d) Traversing the nodes and checking if the next element equals null.
Answer: c) Inserting a new node.
13. What is the time complexity of traversing a list of nodes in a linked list?
a) O (1)
b) O(n)
c) O (log n)
d) O(n-1)
Answer: b) O(n)
14. What is the main problem with a head in a linked list?
a) Null pointers could be caused.
b) Stack overflow errors might be caused.
c) Deletions of memory could occur.
d) It could lead to segmentation errors.
Answer: a) Null pointers could be caused.
15. What is the position of the head node in a linked list when moving forward?
a) Null
b) Second element
c) First element
d) Last element
Answer: c) First element
16. What is the usage of the last dot next in a linked list?
a) Printing the value of the current node.
b) Changing the value of the current node.
c) Moving the loop forward.
d) Checking if the current node exists.
Answer: c) Moving the loop forward.
17. What is the usage of the public void in Python?
a) Looping to remove a value from a list.
b) We loop to output the head value till it reaches the current node.
c) Create a linked list insert node function.
d) Functions that output linked list head and tail values.
Answer: b) We loop to output the head value till it reaches the current node.
Conclusion This blog offers a comprehensive guide for answering common doubly linked list interview questions, from basics such as insertion, deletion and traversal to more advanced concepts such as insertion-deletion traversal (IDT).
At its core, the linked list data structure interview questions blog provides a comprehensive guide to common questions related to linked lists. It provides detailed explanations and examples to enhance your knowledge and help you prepare for interviews more efficiently.
Following its advice can increase your chances of success while setting you apart from competitors; beginners and advanced programmers can benefit.
Take time now and read and practice these Java linked list interview questions; they will surely come in handy during future job interviews!
Understanding interview questions on linked list data structure with answers is critical for aspiring programmers. Proper preparation and understanding enable you to respond confidently to any related list question.
Utilise these programming questions on the linked list blog’s tips and insights as resources in tackling related list interviews so that you keep their fear from holding back your efforts in taking your following interview. Instead, use this resource linked list Dsa questionsas an advantage over potential opponents!
By understanding its principles and practices, you’ll be better equipped to tackle linked list coding interview questions any question you ask during an interview session confidently; always approach interviews confidently while practising responses to achieve faster career success!
With knowledge and skills at your disposal, you’re well-positioned for any opportunity that comes your way, which will undoubtedly increase success rates significantly when approaching linked list coding questions or career objectives set for success by career success!
GoodLuck!
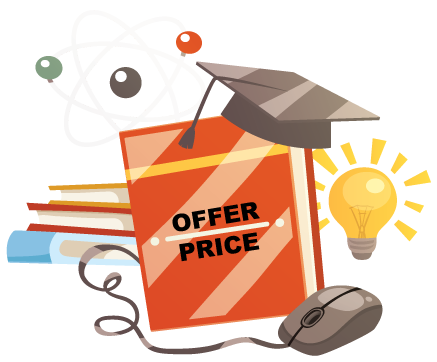
Linked List Course Price
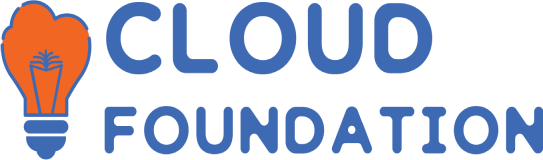
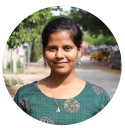
Prasanna
Author