LINQ Interview Questions and Answers
LINQ, or Language-Integrated Query, is a powerful tool used in computer programming to simplify the process of working with data,
LINQ developers can write queries that are both easy to read and understand while also taking advantage of the full capabilities of their programming language, whether you’re working with databases, XML files, or other data types, LINQ can help you do the job quickly and efficiently.
1.What is LINQ?
LINQ is a language-integrated query that allows for querying various data stores, including SQL Server, XML documents, and memory objects.
2. How does LINQ work?
To fetch data from an SQL Server database, a developer must understand a new .net and transact SQL specific to the SQL Server database; LINQ enables developers to work with these different data sources using a similar programming style without knowing specific syntax or technologies.
3. What is the benefit of using LINQ?
The benefit of using LINQ is its intelligence and compile-time error checking, a Dot Net application can be developed in any Dot Net-supported programming language, and a LINQ query can be written using any of these languages; a LINQ provider is responsible for converting the LINQ query into a format that the underlying data source.
4. What is the purpose of the LINQ example?
The LINQ example aims to verify that the query is error-free and that spelling problems are avoided; this is accomplished by integrating LINQ into SQL classes, which generates a student class for us and eliminates the need for manual data retrieval.
5. What is the purpose of using the “where” keyword in storing anonymous types?
The “where” keyword filters out items from the result based on a specific condition, in storing anonymous types, it is used to loop through each item in the result and print the properties of each item and their index position.
6. What is a LINQ query?
A LINQ query retrieves data from an online SQL Server database, it uses IntelliSense to display all properties of the student object and converts the LINQ query into transacting SQL that the underlying database.
7. What is the aim of IntelliSense in a LINQ query?
IntelliSense’s purpose in a LINQ query is to display all properties of the student object; this allows users to quickly select the data they need and ensures accurate results.
8. How important is compile time error checking in a LINQ query?
Compile time error checking is essential in a LINQ query to ensure accurate results, Opaque strings in the query can cause errors if not properly checked.
9. What dynamic SQL is generated by a LINQ to the SQL provider?
The dynamic SQL generated by a LINQ to the SQL provider is the SQL code executed by the database; this code is generated based on the LINQ query and is committed to retrieving the desired data.
10. What is the purpose of a LINQ query in retrieving even numbers from a list of numbers from 1 to 10?
The purpose of a LINQ query in retrieving even numbers from a list of numbers from 1 to 10 is to work with the LINQ to the SQL provider and convert it into a transact SQL query; the grid view one dot data source is used.
The condition is to select even numbers where number mod 2 equals 0, this logic is applied to every number within the array of numbers, and the data bind is invoked.
This allows the user to easily select and display only the even numbers within the list.
11. What is the purpose of LINQ queries in SQL Server?
LINQ queries in SQL Server allow the actual SQL provider to convert the query into a syntax specific to the underlying data source.
12. What are extension methods?
Extension methods are added to existing classes in Visual Studio, such as the Enumerable and List classes; they can extend the functionality of these classes.
13. Can extension methods be implemented on the string class in Visual Studio?
No, extension methods cannot be implemented on the string class in Visual Studio; the string class does not belong to the .NET framework.
14. What is the alternative to extending the string class in Visual Studio?
An alternative to extending the string class in Visual Studio is to create a wrapper or helper class that can be added to the project as a class file; this allows for easier access to the method and its implementation in the code.
15. How does the “change first letter case” method work?
The “change first letter case” method works by converting the input string into a character array, checking if it is uppercase using ease up of function and returning true or false; if it is true, it converts the letter to lowercase using the lower function; otherwise, it is in lowercase, the entire character array is then created and returned.
16. What changes must be made to the original function to use it as an instance method?
To use the original function as an instance method, the class must be made a static class, and the type to extend should be specified; the type that is being developed should be passed as the first parameter to the function.
If other parameters are passed, they must differ from the first one. The first parameter should be the type that is being extended, and the keyword should proceed with the type name.
17. What are extension methods in C#?
Extension methods are unique static methods in C# that allow developers to add methods to existing types without creating a new derived type or recompiling or modifying the original type; they are designed to make the developer’s life easier by allowing them to be used as instance methods on the list class.
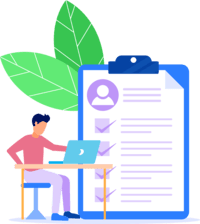
LINQ Training
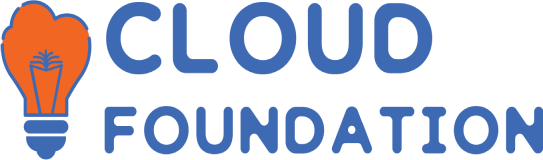
We can use the take method to return a specified number of elements from the start of the collection, with the number of items to be returned using the count parameter, for example, we can store the result in a console application using a loop for each string in the result collection and print out the value of that string on the console screen.
19. What is the difference between using an extension method and a method defined in a type?
There is no difference between calling an extension method and a method defined in a type, both can be reached using the dot notation and can be used to add functionality to existing classes.
20. How is Visual Studio’s, Where Extension Method used?
In Visual Studio, the where extension method retrieves only even numbers from a list of numbers, a lambda expression is specified using the Where method, which returns an i-enumerable of integers.
21. What is the purpose of using the wrapped class syntax style?
The wrapper class syntax style syntax makes it easier for developers to access and use extension methods without creating a new derived type or recompiling the original type, it is a way to call extension methods as if they were instance methods on the type being extended.
22. How do you create a variable of type I-enumerable of integers?
To create a variable of type I-enumerable of integers, we can use a lambda expression to specify the predicate for the where extension method and then use a 4-H loop to iterate through each integer in the collection.
23. What is the parameter called predicate, and what is it?
The parameter called the predicate is Funk InComeable, it is necessary because the Where method is implemented as an extension method.
24. What are the two rules for an extension method?
The first parameter should be the parameter that this extension method is extending, and a keyword should precede the second parameter.
25. What is the first parameter in the Where Extension Method?
The first parameter in the Where Extension Method is the type of function being extended.
26. What is the second parameter in the Where Extension Method?
The second parameter in the Where Extension Method is the type of function being passed to the method.
27. What is the first parameter type in IntelliSense?
The first parameter type in IntelliSense is a generic type T and T result.
28.What is the expected return type of the Where Extension Method?
The expected return type of the Where Extension Method is Boolean.
29. Why do we get a compilation error when we add a value of 2 to X?
When we add a value of 2 to X, we get a compilation error because delegates are type-safe function pointers, and the expression doesn’t evaluate to a Boolean true or false.
30. What is the syntax for using extension methods in C#?
The syntax for extension methods in C# involves using the dot notation to call the method on the extended object.
For example, if you have a list of integers and you want to use the where extension method to retrieve only even numbers, you would call the method like this: myList.Where(x => x % 2 == 0);
31. What is the input parameter of the function?
The input parameter of the function is string.
32. What is the first overloaded version of the where extension method in SQL?
The first overloaded version of the where extension method in SQL expects an integer input parameter and returns a Boolean value.
33. How are extension methods used in C#?
Extension methods are used in C# by calling them an instance method on the extended type; they often add functionality to existing classes without modifying the original code.
34. What is the purpose of using the select extension method in SQL?
The use of the select extension method in SQL is to select specific elements from a sequence and project new types with the properties of number and index.
35. What is the purpose of the sample data context class?
The sample data context class is the gateway into the database; it retrieves data from the SQL Server database using a LINQ query; in the code-behind file, we write a LINQ to the sample data context class to retrieve data from the SQL Server database.
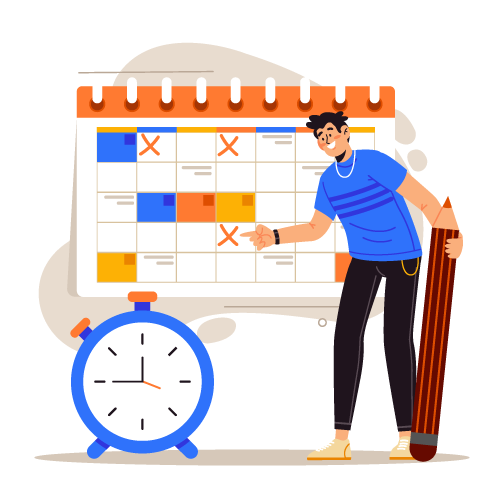
LINQ Online Training
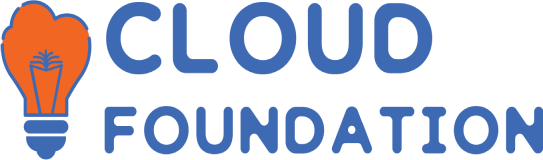
36. What is the purpose of each loop in SQLite?
For each loop, iterates over the result set for each care C in the result and prints the character on the console.
37. How can we retrieve only the distinct subject names?
We can use the distinct operator to give us unique subject names.
38. How is the “change first letter case” method called?
The “change first letter case” method uses the class name followed by the dot (.) and the method name, for example, StringHelper.changeFirstLetterCase(“Hello World”).
39. What is the name of the class created?
The name of the class created by string helper.
40. What is the function name within the class?
The function name within the class is change first letter case.
41.What is the purpose of using the select extension method in SQL?
The purpose of using the select extension method in SQL is to project new types with two properties: number and index.
42. What is the purpose of the function?
To change the case of the first letter within the input string passed to the method.
43. What is the condition for returning the input string without modification?
If the length of the input string dot is greater than 0, it means there is at least one character within the string, if the length is 0, or the string is empty, the input string is returned.
44. How is the function called within the class?
The class name must be used, two simple changes need to be made: making the class itself a static class, and specifying the type to extend, the string class should be the first parameter within the function, the type name should also be preceded by a keyword.
45. What is the condition for calling the function as an instance method?
The syntax for calling the “change first letter case” method is not present within the string class, so it cannot be called as an instance method.
Choose the correct answer for your better understanding of LINQ
46. What is Link?
Answer: A tool that allows developers to work with different data sources using a similar programming style without needing to know specific syntax or technologies
Database transact language.
Query language.
Programming language.
47. What is a Link Provider?
A tool that allows developers to work with different data sources using a similar programming style without knowing specific syntax or technologies.
Database transact language.
Query language.
Answer: A tool responsible for converting the link query into a format that the underlying data source can understand
48. What is the purpose of adding a font family to the area?
Answer: The text is more readable
Add a new feature to the code
To improve the performance of the application
Fix the errors occurring at runtime
49. What should be done to avoid potential spelling mistakes in the SQL query?
IntelliSense to suggest the correct spelling
Answer: A component called link to SQL classes
Add a font family to the area
Windows authentication to connect to the SQL server
50. What should be done to retrieve data from the SQL Server database using a link query?
Create an instance of the sample data context class
Set the data source for the grid as the SQL server database
Write the SQL query in the from keyword
Answer: Specify the actual source where the students are present in the data context
51. What is the purpose of using IntelliSense in a link query?
Display all properties of the student object
Generate transact SQL queries
Answer: Perform compile time error checking
Filter male and female students
52. What is the role of the link provider in a link query?
Answer: Transform the link query into transact SQL queries
Filter male and female students
Display all properties of the student object
Execute the dynamic SQL query
53. What is the purpose of using a dynamic SQL query in a link query?
Filter male and female students
Answer: Retrieve even numbers from a list of numbers
Display all properties of the student object
Execute the link query to S Q L provider
54. Which SQL Server data source?
Answer: SQL Server
In-memory objects like arrays
Oracle
MySQL
55. What is the purpose of using link queries?
Answer: Convert the query into a syntax specific to the underlying data source
Apply logic to every number within the array of numbers
Bind data
Achieve differences between different data sources
56. What is the purpose of extension methods?
Define methods that can be called on classes
Answer: To ensure the functionality and functionality of control applications
Convert the query into a syntax specific to the underlying data source
Logic to every number within the array of numbers
57. What is the first parameter in an IntelliSense extension method?
Answer: A generic type T
The output parameter
A Boolean expression
The input parameter
58. Which operators can retrieve distinct subject names?
Select
Answer: Distinct
Count
Take
LINQ (Language Integrated Query) is a powerful feature in Microsoft’s. NET framework that enables developers to communicate with object-oriented databases; during a LINQ interview, applicants may be given a variety of LINQ-related questions to check their comprehension and competency.
When answering these questions, applicants should show a solid knowledge of LINQ ideas and be able to describe how to utilise LINQ to solve issues.
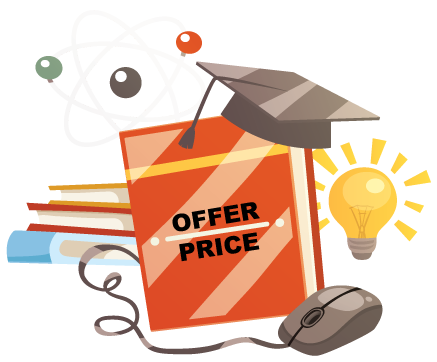
LINQ Course Price
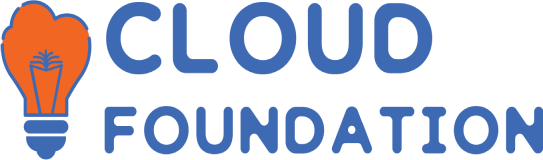
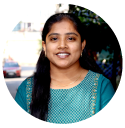
Srujana
Author