ES6 Interview Questions
For programming in JavaScript, the most up-to-date edition is known as ECMAScript 6 or Edition of ES6 — also referred to by other names including ECMAScript 6. Due to these new features and enhancements being integrated in its pervious Edition, many applicants for interviews in the field of JavaScript use ES6 as part of their answer.
With the many powerful capabilities offered by ES6, such as arrow functions, template literals, promises and modules – you could create more efficient and clearer code than ever.
Being well-versed in ES6 is crucial if you hope to succeed at any JavaScript interview. This article discusses commonJavaScriptES6 interview question with advice for how best to answer them.
1.What is ES6?
ES6 (ECMAScript 6) is a version of JavaScript introduced in 2015. It has many new features such as fat arrow functions, constant declarations with a single keyword, modules, classes, spread operators, new methods with different types of literals, and primitive data types.
2.Mention the difference between “let” and “var” in JavaScript?
“let” is block scoped and only accessible inside the block it’s defined, while “var” is function scoped and accessible outside the function.
3.Discuss the purpose of using the “const” keyword in JavaScript?
“const” is used to define constants and variables, which are block scoped and only accessible inside the block they’re defined. Constants cannot be re-assigned.
4.List out the difference between dot notation and bracket notation in JavaScript?
Dot notation allows us to access the name property and reassign it to a different value, while bracket notation is used when we don’t know ahead of time what property or method we’re going to access.
5.Tell the difference between the “walk” keyword in JavaScript and other programming languages?
In other programming languages, the “walk” keyword returns a reference to the current object when calling a function as a method in an object. In JavaScript, it returns the global object, which is the window object in browsers.
6.How does the “walk” keyword prevent potential problems in JavaScript?
The strict mode is enabled by default, which prevents potential problems.
7.Mention the “walk” keyword be used to always return a reference to the personal object in JavaScript?
In JavaScript, functions are objects, and the “dot” symbol represents all the members of the walk object. By using this syntax, we can use the “walk” keyword to always return a reference to the personal object.
8.Discuss the purpose of using the “bind” method in JavaScript?
The “bind” method allows us to set the value of a function permanently, like in the case of a walk function. By passing a person object as an argument, we get a walk function that is always attached to the person object.
9.What is modern JavaScript’s favorite feature?
Arrow functions, which can be used to simplify code.
10.How can arrow functions be used to simplify code in JavaScript?
By defining a constant like square and setting it to a function that returns number times number, we can convert it to an arrow function using a fat arrow between parameters and the body of the function. This allows for a cleaner and more compact syntax, making it easier to read.
11.Mention the purpose of using an error function in JavaScript?
The error function in JavaScript reduces noise and improves the readability of the code by filtering jobs where the job is active.
12.What is the problem with using the “setTimeout” function in JavaScript?
When a call to the “setTimeout” function is wrapped inside a callback function, it returns the window object instead of the person object. This is because the callback function is not part of any objects, and the strict mode does not override this behavior.
13.How can the problem with the “setTimeout” function be solved in JavaScript?
A solution is to declare a variable outside of the callback function and set it to the person object. This way, when the callback function is changed to an arrow function, it inherits the “this” keyword in the context in which the code is defined. This ensures that the person object is referenced in the callback function, and the error functions do not rebind the “this” keyword.
14.Tell about the purpose of introducing the “map” method in ES6?
The “map” method in ES6 is useful for rendering lists in React by transforming each element in an array.
15.How can the “map” method be used in JavaScript?
A callback function is passed to the “map” method to transform each element in the array. The function takes one item at a time and returns a new item.
16.Mention the aim of template literals be used in JavaScript to improve code cleanliness?
Template literals can be used to define a template for a string, add a dollar sign and curly braces, and render a variable dynamically. This creates a new array with the desired result.
17.What is object destructuring in JavaScript?
Object destructuring is a modern JavaScript feature used in React applications to extract specific properties from objects and store them in variables. It eliminates the repetition of object dot in multiple places.
18.Tell about the spread operator in JavaScript?
The spread operator is another modern JavaScript feature that allows for easy cloning of an array by spreading each item in the first array and then adding another element at the end. It can also be used to combine two objects into one.
19.Discuss the process of spread operator be applied on objects in JavaScript?
To apply the spread operator on objects in JavaScript, define a constant and create a new object with the main property set to match. Then, combine the two objects into one object by declaring another constant called combined.
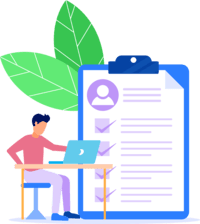
ES6 Training
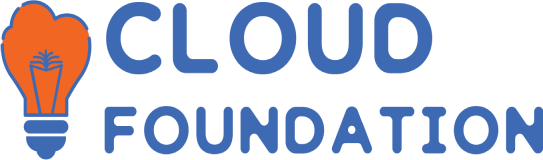
20.What is the purpose of using classes in JavaScript?
The purpose of using classes in JavaScript is to create objects with at least one method. They can also help solve problems by implementing a walk method in a single place, making it easier to find bugs in future versions.
21.Define name parameter in the constructor method of a JavaScript class?
The name parameter in the constructor method of a JavaScript class is passed from the outside and is set to the name argument.
22.discribe the “new” keyword or operator in JavaScript?
The “new” keyword or operator is important in JavaScript, as it allows for easy modification of the method. It is particularly useful when creating personal objects using a class.
23.What is inheritance in React?
Inheritance in React is a technique where a teacher class inherits all methods defined in the person class. To do this, the person class is extended with the keyword “extends person.”
24.How is inheritance implemented in React?
To implement inheritance in React, the author adds the “extends person” keyword to the person class. When creating a teacher object, the name and the teach method are inherited from the person class.
25.Describe the modularity implemented in JavaScript?
Modularity in JavaScript involves splitting code into multiple files, each called a module. To implement modularity, each class is moved into a separate file, such as the person class and the teacher class.
To make the objects defined in a module visible, they must be exported from the class and imported wherever needed.
26.What is private objects in a module?
Private objects in a module refer to functions defined within a module that are only accessible to that module and not outside of it.
27.Define named exports in a module?
Named exports in a module refer to the main objects exported from a module. Named exports are explicitly defined and can be imported using a specific name. The default export is the main object that is exported from a module, and is typically used when there is only a single object to export.
28.What is the default export in a JavaScript module?
The default export in a JavaScript module is the teacher object.
29.Mention the import statement used in JavaScript?
The import statement is used to import the default export from a module in JavaScript.
30.List out the difference between named and default exports in JavaScript?
Named exports are specific objects that are exported from a module, while default exports are the main object that is exported from a module.
31.How does the concept of named exports work in React modules?
In React modules, the component class is used to extend the module’s behavior and methods.
32.Why is it important to understand the difference between named and default exports in JavaScript?
It is important to understand the difference between named and default exports in JavaScript to avoid confusion and ensure correct usage of the exported objects.
33.Define promises in JavaScript?
Promises are objects that represent an operation that has not been executed yet and can be attached to callbacks when returned.
34.What is the purpose of using promises in JavaScript?
The purpose of using promises in JavaScript is to handle asynchronous operations more efficiently and effectively.
35.What is the callback functional style in JavaScript?
The callback functional style in JavaScript involves executing a sequence of operations, such as a login function that expects an email and password.
36.How does the callback functional style work in JavaScript?
In the callback functional style, the server validates the email and password and returns a response, either a success or error response.
To continue execution, the function needs to expect another argument, a success callback, and a failure callback. When the login function is called, the user object is passed to the load user profile function, which then expects a success callback and error handler callback.
The success callback returns the user object, while the error callback returns the error object. Finally, the profile is passed to the load user profile function, which then loads profile posts.
37.Define the purpose of the “login” function?
The “login” function checks if the user has an email and password and executes a success or failure callback function after one second.
38.What happens if the “login” function does not receive the email and password?
The failure callback is called, and an error message is sent.
39.Tell about the “load user profile” function work?
The “load user profile” function simulates a server call using a timeout of one second and expects both the success and failure callbacks. If the server call is successful, the success callback function is called, and an array of posts is passed. After the success callback, the error handler is called, and the error message is sent.
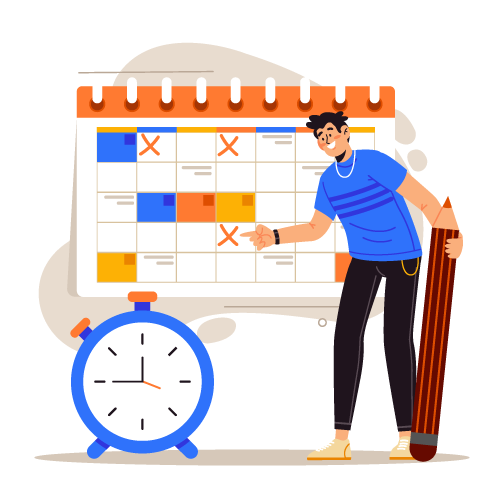
ES6 Online Training
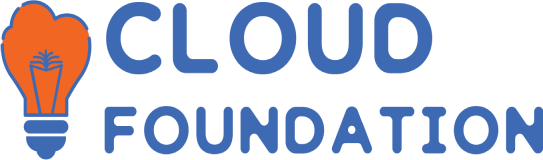
40.What is callback hell?
Callback hell is a pyramid of callbacks that can be cumbersome when dealing with nested or sequential asynchronous operations. It can become challenging to read through the code and track down changes at every step.
41.What is the purpose of using promises in JavaScript?
Promises help manage the complexity of nested or sequential asynchronous operations and ensure that the system works correctly. They allow developers to better understand the behavior of their application and ensure that the system is functioning properly.
42.Mention the aim of the “log in” function in this example?
The purpose of the “log in” function is to check if the user has an email and password and to execute a success or failure callback function after one second.
43.What happens if the “log in” function does not receive the email and password?
The failure callback is called, and an error message is sent.
44.Point of the difference between using a callback function and a promise object in this example?
Instead of passing a callback function as an argument to the function, a promise object is created and attached to the then call. This creates a chain of then calls, allowing multiple then calls to run synchronously one after another.
45.What is the purpose of the “load user profile” function in this example?
The “load user profile” function is called once the user object is obtained and executes the user object.
46.How is the “log function” used in this example?
The “log function” is called with the email and password as arguments, then another function is called that receives a user object. The user object contains an idea name and email, and the callback function then executes a low user profile.
47.What is the difference between using a try-catch block and a catch clause in this example?
A try-catch block is used to handle errors in the code. A catch clause is used to handle errors in the promise object.
48.Describe the “all” method do in the promise object?
The “all” method executes a single callback when all promises are resolved. If anything fails, it goes to the catch clause.
49.What does the “race” method do in the promise object?
The “race” method executes whatever is passed to the then function once one of the promises is resolved.
Came to end with questions and answers not lets give a try with MCQ’s!!!
1) What is ES6?
The latest version of JavaScript released this year.
Beta version of JavaScript released in 2010.
The foundation version of JavaScript before any updates.
New version of JavaScript introduced in 2015.
2) What are promises in JavaScript designed for?
Handling asynchronous operations.
Generating synchronous executions.
Managing conditional statements.
Controlling looping structures.
3) What is the purpose of using the ‘catch’ clause with promises?
Handling errors and failures.
Scaling the application.
Optimizing performance.
Controlling memory allocation.
4) What is a common use of the spread operator in JavaScript?
Declaring global variables.
Combining two arrays into one.
Creating infinite loops.
Defining anonymous functions.
5) According to the text, what are objects in JavaScript?
Complex mathematical equations.
Control structures for decision-making.
Sequential operation handlers.
Collections of key-value pairs.
6) What is the primary purpose of using the let keyword in ES6?
To concatenate strings.
Define constants that cannot be re-assigned.
To make variables accessible only within the function block where they are defined.
Declare global variables.
7) How does the spread operator in ES6 primarily benefit array manipulation?
Increases the execution time for array operations.
It directly modifies the original array.
Enables deep cloning of array objects.
It allows for the easy combination or cloning of arrays without explicitly calling methods like concat.
8) In the context of ES6 classes, what role does the ‘constructor’ method serve?
Prevents the instantiation of a class.
It initializes the class object properties when a new instance of the class is created.
Ensures that a class cannot inherit from other classes.
It acts solely as a destructor to clean up resources.
9) How does the use of promises in ES6 improve handling asynchronous operations?
Promises require more complex error handling mechanisms.
Completely eliminate the need for asynchronous operations.
Promises provide a cleaner and more manageable way to handle success or failure of asynchronous operations than traditional callback functions.
Decrease the execution speed of functions.
10) In the modularization of JavaScript code with ES6, what is the significance of ‘export’ and ‘import’ statements?
Used primarily for styling purposes.
They decrease code execution efficiency by loading all modules at once.
Increase the global scope pollution by exporting variables.
They allow for the separation of code into modules, making the application more maintainable by isolating functionalities.
Summing up!
JavaScript programming language has seen immense advancement thanks to ES6’s numerous enhancements and capabilities. Therefore, before entering any interview for JavaScript development positions make sure you possess an in-depth knowledge of ES6 and know what its implications will be for any given situation.
This Blog has covered many of the more frequently encountered ES6 JavaScript interview questions and provided advice for responding.
Utilize ES6features interview questions in your projects, whether or not you are experienced JavaScript developer. Becoming adept with ES6 will set yourself apart at future interviews but will require hard work.
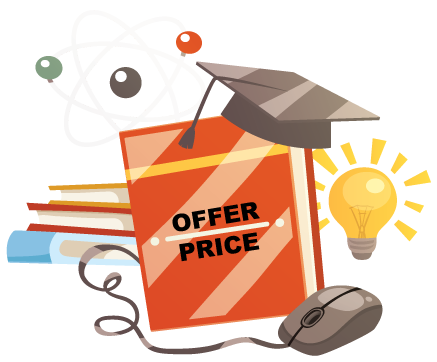
ES6 Course Price
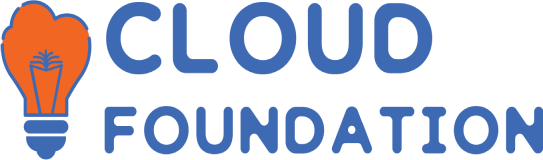
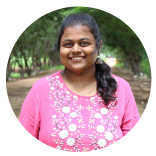
Harsha Vardhani
Author